#what are data types in python
Explore tagged Tumblr posts
Text
What are the data types in Python?

Python training It supports several built-in data types, which are fundamental for working with data and performing various operations. Here are the most common data types in Python:
Numeric Types
int: Represents integer values, both positive and negative.pythonCopy codemy_int = 42
float: Represents floating-point (decimal) numbers.pythonCopy codemy_float = 3.14159
complex: Represents complex numbers with a real and imaginary part.pythonCopy codemy_complex = 2 + 3j
Text Type
str: Represents strings, which are sequences of characters enclosed in single or double quotes.pythonCopy codemy_string = "Hello, Python!"
Sequence Types
list: Represents ordered collections of items. Lists can contain elements of different data types and are mutable.pythonCopy codemy_list = [1, 2, 3, "Python", True]
tuple: Similar to lists but immutable, meaning their contents cannot be changed once created.pythonCopy codemy_tuple = (1, 2, 3, "Python", True)
range: Represents a sequence of numbers, commonly used for iterations.pythonCopy codemy_range = range(1, 6) # Represents [1, 2, 3, 4, 5]
Mapping Type
dict: Represents dictionaries, which are collections of key-value pairs. Dictionaries are unordered.pythonCopy codemy_dict = {"name": "Alice", "age": 30, "city": "New York"}
Set Types
set: Represents an unordered collection of unique elements.pythonCopy codemy_set = {1, 2, 3, 4, 5}
frozenset: Similar to sets but immutable, meaning their contents cannot be changed after creation.pythonCopy codemy_frozenset = frozenset({1, 2, 3})
Boolean Type
bool: Represents Boolean values, either True or False.pythonCopy codeis_valid = True
Binary Types
bytes: Represents a sequence of bytes and is immutable.pythonCopy codemy_bytes = b"Hello"
bytearray: Similar to bytes but mutable.pythonCopy codemy_bytearray = bytearray([72, 101, 108, 108, 111])
None Type
NoneType: Represents the absence of a value or a null value. It is often used to indicate that a variable does not point to any object.pythonCopy codemy_var = None
0 notes
Text
Hey everyone!
I know it’s been a minute, but with the recent news that Automattic has laid off a portion of its workforce (including a sizable percent of tumblr's staff), it's a good time to have a quick chat about the future.
Now, as far as we know, tumblr isn't going anywhere just yet. No need to panic! However, I do recommend that you take into consideration a few things.
1: Backup your tumblr! Here's tumblr's official guide to doing so. It's always a good idea to have backups of your data and now is a great time to do so.
*I’ll also be including a handful of other links walking you guys through other backup methods at the end of this post. As I understand it, each of them have different pros and cons, and it might be a good idea to have more than one type of backup depending on what you want to save/how you’d like the backup to look/etc.
2: Have some place your mutuals/friends can find you! A carrd or linktree is a great way to list off anywhere you might find yourself on the internet.
3: Once again, don't panic! We don’t know that anything is happening to tumblr anytime soon—it just doesn’t hurt to have a backup. Better to have a plan now instead of being blindsided later.
*The other backup methods I’ve been able to find:
—First off, someone put together a document with several backup methods & pros and cons for each. (I believe it originated from a tumblr post, but with search being the way it is I haven’t been able to track it down.) This goes over a lot, but I’m adding a few more links to this post in case they might be helpful too.
—This post and this video were a good guide to the older “bbolli tumblr-utils backup for beginners” method mentioned in the doc (I used them during the ban in 2018 to make sure I had my main blog saved).
—I’ve also found a handful of python & python 3 tumblr backup tutorial videos out there, in case those would be helpful for you. (I haven’t personally tried these methods out yet, but the videos seem to go over the updated version of tumblr-utils.
#psa#tumblr backup options#doc rambles#again: don’t panic#we don’t know anything is happening right now#but it’s always handy to have a backup just in case!
754 notes
·
View notes
Text
research & development is ongoing
since using jukebox for sampling material on albedo, i've been increasingly interested in ethically using ai as a tool to incorporate more into my own artwork. recently i've been experimenting with "commoncanvas", a stable diffusion model trained entirely on works in the creative commons. though i do not believe legality and ethics are equivalent, this provides me peace of mind that all of the training data was used consensually through the terms of the creative commons license. here's the paper on it for those who are curious! shoutout to @reachartwork for the inspiration & her informative posts about her process!
part 1: overview
i usually post finished works, so today i want to go more in depth & document the process of experimentation with a new medium. this is going to be a long and image-heavy post, most of it will be under the cut & i'll do my best to keep all the image descriptions concise.
for a point of reference, here is a digital collage i made a few weeks ago for the album i just released (shameless self promo), using photos from wikimedia commons and a render of a 3d model i made in blender:
and here are two images i made with the help of common canvas (though i did a lot of editing and post-processing, more on that process in a future post):
more about my process & findings under the cut, so this post doesn't get too long:
quick note for my setup: i am running this model locally on my own machine (rtx 3060, ubuntu 23.10), using the automatic1111 web ui. if you are on the same version of ubuntu as i am, note that you will probably have to build python 3.10.6 yourself (and be sure to use 'make altinstall' instead of 'make install' and change the line in the webui to use 'python3.10' instead of 'python3'. just mentioning this here because nobody else i could find had this exact problem and i had to figure it out myself)
part 2: initial exploration
all the images i'll be showing here are the raw outputs of the prompts given, with no retouching/regenerating/etc.
so: commoncanvas has 2 different types of models, the "C" and "NC" models, trained on their database of works under the CC Commercial and Non-Commercial licenses, respectively (i think the NC dataset also includes the commercial license works, but i may be wrong). the NC model is larger, but both have their unique strengths:
"a cat on the computer", "C" model
"a cat on the computer", "NC" model
they both take the same amount of time to generate (17 seconds for four 512x512 images on my 3060). if you're really looking for that early ai jank, go for the commercial model. one thing i really like about commoncanvas is that it's really good at reproducing the styles of photography i find most artistically compelling: photos taken by scientists and amateurs. (the following images will be described in the captions to avoid redundancy):
"grainy deep-sea rover photo of an octopus", "NC" model. note the motion blur on the marine snow, greenish lighting and harsh shadows here, like you see in photos taken by those rover submarines that scientists use to take photos of deep sea creatures (and less like ocean photography done for purely artistic reasons, which usually has better lighting and looks cleaner). the anatomy sucks, but the lighting and environment is perfect.
"beige computer on messy desk", "NC" model. the reflection of the flash on the screen, the reddish-brown wood, and the awkward angle and framing are all reminiscent of a photo taken by a forum user with a cheap digital camera in 2007.
so the noncommercial model is great for vernacular and scientific photography. what's the commercial model good for?
"blue dragon sitting on a stone by a river", "C" model. it's good for bad CGI dragons. whenever i request dragons of the commercial model, i either get things that look like photographs of toys/statues, or i get gamecube type CGI, and i love it.
here are two little green freaks i got while trying to refine a prompt to generate my fursona. (i never succeeded, and i forget the exact prompt i used). these look like spore creations and the background looks like a bryce render. i really don't know why there's so much bad cgi in the datasets and why the model loves going for cgi specifically for dragons, but it got me thinking...
"hollow tree in a magical forest, video game screenshot", "C" model
"knights in a dungeon, video game screenshot", "C" model
i love the dreamlike video game environments and strange CGI characters it produces-- it hits that specific era of video games that i grew up with super well.
part 3: use cases
if you've seen any of the visual art i've done to accompany my music projects, you know that i love making digital collages of surreal landscapes:
(this post is getting image heavy so i'll wrap up soon)
i'm interested in using this technology more, not as a replacement for my digital collage art, but along with it as just another tool in my toolbox. and of course...
... this isn't out of lack of skill to imagine or draw scifi/fantasy landscapes.
thank you for reading such a long post! i hope you got something out of this post; i think it's a good look into the "experimentation phase" of getting into a new medium. i'm not going into my post-processing / GIMP stuff in this post because it's already so long, but let me know if you want another post going into that!
good-faith discussion and questions are encouraged but i will disable comments if you don't behave yourselves. be kind to each other and keep it P.L.U.R.
202 notes
·
View notes
Text
Nov. 9th Journal
Day one of the 50-day challenge
🦉 Habit 1: Studying - Data Analysis Course Assignment Unit 3 - Data Analysis Course 3.3 - Data Analysis Course 3.4 - Do one practice with Cognos - Type out the rest of my handwritten notes - Complete the Text-Based Python Slot Game - Make a wireframe for the app I've been wanting to make for years
🍂Habit 2: Physical Activity - 10 minute AB workout - 30 minutes of walking 🕯️Habit 3: Self-care - No soda today - Include one vegetable in every meal - 1/2 bottle of water today (gotta start small) - Hang my clothes - Do my hair with heatless curls - Fill up a lip care tube for myself - Set up my home office desk 📰 Habit 4: Reading - You Need a Budget, by Jesse Mecham (100 Pages) 🏛️Habit 5: Language Learning - Really sit down and think about what I want to learn, because right now I want to learn it all
🎻 Song of The Day: Mouvement I, Voyage Vers la Lune - Franz Gordon 🤎 Quote of The Day: “There is no shame in not knowing something. The shame is not being willing to learn.” - Alison Croggon, The Naming 📜 Currently Studying: Python, Data Analysis 🪶 Recommendation of The Day: please start learning that new school you've always wanted to. It's never too late to change an old habit or to learn a new skill. There are a lot of internal and external struggles when you begin but the entire Journey and learning by itself is always worth it.
Images are mine but please just credit if you use!
This Challenge is entirely credited to @ros3ybabe
Divider credits to @strangergraphics
#studyblr#study blog#studyspo#study motivation#to do list#daily journal#dark academia#light academia#langauge learning#coding#it girl self care#it girl#that girl#glow up#clean girl#becoming that girl#bunnies 60 day challenge#journal#journaling
25 notes
·
View notes
Note
hi! research question I'd love your input on:
do you know how to generate size-of-fandom stats? I'm researching the Ghost fandom and while I know from the This Week in Tumblr posts about what their size is *now,* I'd like to try to compare it to past years, and be able to make statements like "x% of the fandom is reblogging explicit content."
I'm also looking for deeper info on how tumblr works if someone deactivates - do their notes vanish too?
It sounds like you want to gather Tumblr information only -- is that right? I don't have a lot of expertise with Tumblr data (I think I last gathered some over a decade ago), but it looks like their API still lets you retrieve posts with a certain tag and specify a timestamp, if you're willing to do a bit of programming. So you could, e.g., retrieve the last N posts of each month that use a particular fandom tag. And then you can compare those samples of posts to see how the content has changed over time. If you want to do that, there are libraries in Python and probably other languages that can make it easier to work with the Tumblr API.
I believe the posts retrieved this way don't include reblogs, so you'd also have to look at the post notes to get info about how many reblogs different types of posts are getting. As to your question of deactivated accounts within those notes, I'm not certain of the answer. I frequently see reblog chains where some accounts in the chain have deactivated, so those notes are not entirely gone. But I don't know if the replies/likes from deactivated accounts disappear from post notes. Anyone else know?
12 notes
·
View notes
Note
So, uhhh, first ever message on tumblr, here's to hoping this is actually the place to post that stuff?
It's great, As a Game Master (mostly for rpgs on discord) I'm a big fan of the freedom of expression when it comes to characters. I just finished a run I'm pretty happy with, so I figured I should do as asked and share the results below.
(A word of warning, since I can only draw anime-style, this is now an anime.)
As a bonus, here's some design I doodled up for the character, to help myself visualize her.
Additional Data:
Adrian: Crush Merlin: To Befriend Pet: Cat (Roland)(Like the Paladin of Charlemagne) Wears: Frilly blouse and long skirt (She started wearing those to get reactions out of Adrian while avoiding more extreme outfits) Camelot Sequence: Lucid dreamer, talked to Merlin in the past (monty python reference to prove she's from the future), got directed to Arthur, asked Past!Arthur about lucid dreaming, Caught Current!Arthur about Lucid Dreaming (He now knows who she is), then spilled all the beans to Merlin after waking up (He knows who she is). Items: Magic 8-Ball, Cat memento, pouch of pearls, miniature garden (Audrey III!) Gas Station: Followed Merlin, Destroy Cameras, Clean traces, Get matches.
And now, for the wall of texts that nobody asked for but that you're all getting anyway!
Character Design
The design I went with was 'the super sussy character who actually is kinda hiding the least'.
Physically, she's basically hopeless, with her teeny tiny 10% on physical, which puts her firmly into the 'dead weight' category. Considering what's going on in here, it's a bit sus.
Even more sus is the amnesia background, because here's that girl popping out of seemingly nowhere with no memories right around the time the failed Lesser Circle happened, who just so happens to be a potential harbinger? Sus.
Appearance wise, her best features are her eyes (my dice determined they should be violet), but they are downturned (Tareme) ones, that usually express either gentleness, or belong to suspicious people, with a gentle, caring and overall traditionally feminine/motherly behavior (wears frilly outfits, cooks gourmet food, tends to her (mutant) plants). In other words, she's very much an 'ara ara'-type character. (The cuckoo is mostly limited to the confines of her mind).
However, she is an imposter whose special move consists of spilling all the tea (ironic, her favourite drink is Jasmine…), all the time. Had a weird dream (Part 1)? Write a report and drop it on Adrian's lap, almost shoving the marked arm under his nose. Freak encounter in a horse trailer? Write a report and drop it on the club leader's lap. Meet a strange but weirdly helpful stranger? Drop the Weird dream Dossier (part 1) on his lap. Questions about the self medication? Ha! She's got her entire (albeit short) life written in a folder ready for perusal! Had a weird dream (Part 2)? You better bet she's writing it down and dropping it on Merlin and Adrian's laps.
…
This is probably a side-effect of being overworked all the time and getting in the habit of making biology reports for everything, isn't it?
IC Thoughts on characters:
Merlin: Super strong incubus who may or may not be the actual Antichrist, may or may not want to cause the Apocalypse themselves to put humanity out of their misery if the Arthur plan doesn't pan out, and yet may very well be the most trustworthy person in this entire group, if only because waking Arthur up seems to be their number 1 priority. After all, all they needed to do was stand aside and do nothing if they wanted the Lesser Circle to fail, right?
Adrian: 'Friend' for several years, eventually got a crush on him a few months ago, but his ambiguous way of almost-but-not-quite friendzoning her is beginning to wear her down. Slightly suspicious due to his being much too prepared for the crisis at hand for a random bystander, but he had all the opportunities in the world to get rid of/corrupt her in all the years they knew each other, and she herself has this 'tiny' Impostor secret going on so she's trusting him so far.
IC thoughts about the adventure: 4 (Reluctant/panicking, but not forced)
What is there to be said. The end of the world, man-eating monsters, plagues, the end of the world, homicides, abductions, did she forget to mention the end of the world? For a woman with little inclination toward physical fighting and who would rather read a book in a corner, this is very much outside of her field of competence.
With that said, she almost got eaten by the monster under her bed and Merlin healed her when she was at Death's Doors, so she's giving them the benefit of doubt, for now.
Character affinities:
Probably Percival (cuckoo connection), Arthur (dream shenanigans) or Broderick (this entire thing is crazy!) would work best?
Here's to hoping this didn't get too long, I tend to get too verbose when I become enthusiastic about something.
It's impossible to be too verbose, especially compared to me. Also, my imagination runs in 4K surround-sound anime-style, so it was anime all along! Olwen fits in perfectly!
#10% on physical...#Merlin: ( ⚆﹏⚆ )#Gwen: Finally someone I can outrun!#oks-asks#Also with this Ask I have finally cleared my Inbox for the first time in months and-- *more Asks come in as I add in the pics* -- nevermind
21 notes
·
View notes
Text
Ace Attorney Animals on Ao3
Got curious which Ace Attorney animals (and robots/mascots/etc…) were featured in the most fics, so I grabbed some quick Ao3 tag data to investigate. Here’s a graph of what I found:
(The colors are based on fandom: AA = blue, GAA = brown, PLvPW = orange)
Method details below the cut
Data/method: I used the Ao3 tag search for the “逆転裁判 | Gyakuten Saiban | Ace Attorney”, “大逆転裁判 | Dai Gyakuten Saiban | The Great Ace Attorney Chronicles (Video Games)”, and “Layton Kyouju vs Gyakuten Saiban | Professor Layton vs. Phoenix Wright: Ace Attorney” fandoms (one at a time), with the tag type set to “Character” and the wrangling status set to “Canonical”. I copy/pasted the tag counts from each page of results and ran that text through a simple python script to extract the relevant info. I collected the data on January 18th, 2025.
Note that this approach only captures instances of the characters being tagged, so fics that include a character without tagging them wouldn’t be counted here
Also, I thought it was worth mentioning that Moozilla/Taurusaurus, Sniper (the penguin), the Proto Badger, Pink Badger, and Bad Badger all have canonical tags that are currently unused. Very sad 😞
#Missile should be higher up imo#he's so underrated#Ace Attorney#Great Ace Attorney#PLvPW#graphs#Ace Attorney in Graphs#Ao3#Fandom statistics#sC original#Pess#Taka#Mikeko#Vongole#Charley#Missile
13 notes
·
View notes
Text
Your All-in-One AI Web Agent: Save $200+ a Month, Unleash Limitless Possibilities!
Imagine having an AI agent that costs you nothing monthly, runs directly on your computer, and is unrestricted in its capabilities. OpenAI Operator charges up to $200/month for limited API calls and restricts access to many tasks like visiting thousands of websites. With DeepSeek-R1 and Browser-Use, you:
• Save money while keeping everything local and private.
• Automate visiting 100,000+ websites, gathering data, filling forms, and navigating like a human.
• Gain total freedom to explore, scrape, and interact with the web like never before.
You may have heard about Operator from Open AI that runs on their computer in some cloud with you passing on private information to their AI to so anything useful. AND you pay for the gift . It is not paranoid to not want you passwords and logins and personal details to be shared. OpenAI of course charges a substantial amount of money for something that will limit exactly what sites you can visit, like YouTube for example. With this method you will start telling an AI exactly what you want it to do, in plain language, and watching it navigate the web, gather information, and make decisions—all without writing a single line of code.
In this guide, we’ll show you how to build an AI agent that performs tasks like scraping news, analyzing social media mentions, and making predictions using DeepSeek-R1 and Browser-Use, but instead of writing a Python script, you’ll interact with the AI directly using prompts.
These instructions are in constant revisions as DeepSeek R1 is days old. Browser Use has been a standard for quite a while. This method can be for people who are new to AI and programming. It may seem technical at first, but by the end of this guide, you’ll feel confident using your AI agent to perform a variety of tasks, all by talking to it. how, if you look at these instructions and it seems to overwhelming, wait, we will have a single download app soon. It is in testing now.
This is version 3.0 of these instructions January 26th, 2025.
This guide will walk you through setting up DeepSeek-R1 8B (4-bit) and Browser-Use Web UI, ensuring even the most novice users succeed.
What You’ll Achieve
By following this guide, you’ll:
1. Set up DeepSeek-R1, a reasoning AI that works privately on your computer.
2. Configure Browser-Use Web UI, a tool to automate web scraping, form-filling, and real-time interaction.
3. Create an AI agent capable of finding stock news, gathering Reddit mentions, and predicting stock trends—all while operating without cloud restrictions.
A Deep Dive At ReadMultiplex.com Soon
We will have a deep dive into how you can use this platform for very advanced AI use cases that few have thought of let alone seen before. Join us at ReadMultiplex.com and become a member that not only sees the future earlier but also with particle and pragmatic ways to profit from the future.
System Requirements
Hardware
• RAM: 8 GB minimum (16 GB recommended).
• Processor: Quad-core (Intel i5/AMD Ryzen 5 or higher).
• Storage: 5 GB free space.
• Graphics: GPU optional for faster processing.
Software
• Operating System: macOS, Windows 10+, or Linux.
• Python: Version 3.8 or higher.
• Git: Installed.
Step 1: Get Your Tools Ready
We’ll need Python, Git, and a terminal/command prompt to proceed. Follow these instructions carefully.
Install Python
1. Check Python Installation:
• Open your terminal/command prompt and type:
python3 --version
• If Python is installed, you’ll see a version like:
Python 3.9.7
2. If Python Is Not Installed:
• Download Python from python.org.
• During installation, ensure you check “Add Python to PATH” on Windows.
3. Verify Installation:
python3 --version
Install Git
1. Check Git Installation:
• Run:
git --version
• If installed, you’ll see:
git version 2.34.1
2. If Git Is Not Installed:
• Windows: Download Git from git-scm.com and follow the instructions.
• Mac/Linux: Install via terminal:
sudo apt install git -y # For Ubuntu/Debian
brew install git # For macOS
Step 2: Download and Build llama.cpp
We’ll use llama.cpp to run the DeepSeek-R1 model locally.
1. Open your terminal/command prompt.
2. Navigate to a clear location for your project files:
mkdir ~/AI_Project
cd ~/AI_Project
3. Clone the llama.cpp repository:
git clone https://github.com/ggerganov/llama.cpp.git
cd llama.cpp
4. Build the project:
• Mac/Linux:
make
• Windows:
• Install a C++ compiler (e.g., MSVC or MinGW).
• Run:
mkdir build
cd build
cmake ..
cmake --build . --config Release
Step 3: Download DeepSeek-R1 8B 4-bit Model
1. Visit the DeepSeek-R1 8B Model Page on Hugging Face.
2. Download the 4-bit quantized model file:
• Example: DeepSeek-R1-Distill-Qwen-8B-Q4_K_M.gguf.
3. Move the model to your llama.cpp folder:
mv ~/Downloads/DeepSeek-R1-Distill-Qwen-8B-Q4_K_M.gguf ~/AI_Project/llama.cpp
Step 4: Start DeepSeek-R1
1. Navigate to your llama.cpp folder:
cd ~/AI_Project/llama.cpp
2. Run the model with a sample prompt:
./main -m DeepSeek-R1-Distill-Qwen-8B-Q4_K_M.gguf -p "What is the capital of France?"
3. Expected Output:
The capital of France is Paris.
Step 5: Set Up Browser-Use Web UI
1. Go back to your project folder:
cd ~/AI_Project
2. Clone the Browser-Use repository:
git clone https://github.com/browser-use/browser-use.git
cd browser-use
3. Create a virtual environment:
python3 -m venv env
4. Activate the virtual environment:
• Mac/Linux:
source env/bin/activate
• Windows:
env\Scripts\activate
5. Install dependencies:
pip install -r requirements.txt
6. Start the Web UI:
python examples/gradio_demo.py
7. Open the local URL in your browser:
http://127.0.0.1:7860
Step 6: Configure the Web UI for DeepSeek-R1
1. Go to the Settings panel in the Web UI.
2. Specify the DeepSeek model path:
~/AI_Project/llama.cpp/DeepSeek-R1-Distill-Qwen-8B-Q4_K_M.gguf
3. Adjust Timeout Settings:
• Increase the timeout to 120 seconds for larger models.
4. Enable Memory-Saving Mode if your system has less than 16 GB of RAM.
Step 7: Run an Example Task
Let’s create an agent that:
1. Searches for Tesla stock news.
2. Gathers Reddit mentions.
3. Predicts the stock trend.
Example Prompt:
Search for "Tesla stock news" on Google News and summarize the top 3 headlines. Then, check Reddit for the latest mentions of "Tesla stock" and predict whether the stock will rise based on the news and discussions.
--
Congratulations! You’ve built a powerful, private AI agent capable of automating the web and reasoning in real time. Unlike costly, restricted tools like OpenAI Operator, you’ve spent nothing beyond your time. Unleash your AI agent on tasks that were once impossible and imagine the possibilities for personal projects, research, and business. You’re not limited anymore. You own the web—your AI agent just unlocked it! 🚀
Stay tuned fora FREE simple to use single app that will do this all and more.
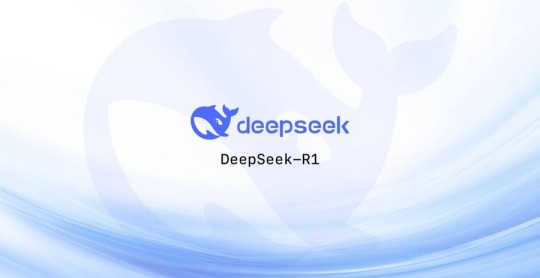
7 notes
·
View notes
Text
Python for Beginners: Launch Your Tech Career with Coding Skills
Are you ready to launch your tech career but don’t know where to start? Learning Python is one of the best ways to break into the world of technology—even if you have zero coding experience.
In this guide, we’ll explore how Python for beginners can be your gateway to a rewarding career in software development, data science, automation, and more.
Why Python Is the Perfect Language for Beginners
Python has become the go-to programming language for beginners and professionals alike—and for good reason:
Simple syntax: Python reads like plain English, making it easy to learn.
High demand: Industries spanning the spectrum are actively seeking Python developers to fuel their technological advancements.
Versatile applications: Python's versatility shines as it powers everything from crafting websites to driving artificial intelligence and dissecting data.
Whether you want to become a software developer, data analyst, or AI engineer, Python lays the foundation.
What Can You Do With Python?
Python is not just a beginner language—it’s a career-building tool. Here are just a few career paths where Python is essential:
Web Development: Frameworks like Django and Flask make it easy to build powerful web applications. You can even enroll in a Python Course in Kochi to gain hands-on experience with real-world web projects.
Data Science & Analytics: For professionals tackling data analysis and visualization, the Python ecosystem, featuring powerhouses like Pandas, NumPy, and Matplotlib, sets the benchmark.
Machine Learning & AI: Spearheading advancements in artificial intelligence development, Python boasts powerful tools such as TensorFlow and scikit-learn.
Automation & Scripting: Simple yet effective Python scripts offer a pathway to amplified efficiency by automating routine workflows.
Cybersecurity & Networking: The application of Python is expanding into crucial domains such as ethical hacking, penetration testing, and the automation of network processes.
How to Get Started with Python
Starting your Python journey doesn't require a computer science degree. Success hinges on a focused commitment combined with a thoughtfully structured educational approach.
Step 1: Install Python
Download and install Python from python.org. It's free and available for all platforms.
Step 2: Choose an IDE
Use beginner-friendly tools like Thonny, PyCharm, or VS Code to write your code.
Step 3: Learn the Basics
Focus on:
Variables and data types
Conditional statements
Loops
Functions
Lists and dictionaries
If you prefer guided learning, a reputable Python Institute in Kochi can offer structured programs and mentorship to help you grasp core concepts efficiently.
Step 4: Build Projects
Learning by doing is key. Start small:
Build a calculator
Automate file organization
Create a to-do list app
As your skills grow, you can tackle more complex projects like data dashboards or web apps.
How Python Skills Can Boost Your Career
Adding Python to your resume instantly opens up new opportunities. Here's how it helps:
Higher employability: Python is one of the top 3 most in-demand programming languages.
Better salaries: Python developers earn competitive salaries across the globe.
Remote job opportunities: Many Python-related jobs are available remotely, offering flexibility.
Even if you're not aiming to be a full-time developer, Python skills can enhance careers in marketing, finance, research, and product management.
If you're serious about starting a career in tech, learning Python is the smartest first step you can take. It’s beginner-friendly, powerful, and widely used across industries.
Whether you're a student, job switcher, or just curious about programming, Python for beginners can unlock countless career opportunities. Invest time in learning today—and start building the future you want in tech.
Globally recognized as a premier educational hub, DataMites Institute delivers in-depth training programs across the pivotal fields of data science, artificial intelligence, and machine learning. They provide expert-led courses designed for both beginners and professionals aiming to boost their careers.
Python Modules Explained - Different Types and Functions - Python Tutorial
youtube
#python course#python training#python#learnpython#pythoncourseinindia#pythoncourseinkochi#pythoninstitute#python for data science#Youtube
3 notes
·
View notes
Text
Why Learning Python is the Perfect First Step in Coding
Learning Python is an ideal way to dive into programming. Its simplicity and versatility make it the perfect language for beginners, whether you're looking to develop basic skills or eventually dive into fields like data analysis, web development, or machine learning.
Start by focusing on the fundamentals: learn about variables, data types, conditionals, and loops. These core concepts are the building blocks of programming, and Python’s clear syntax makes them easier to grasp. Interactive platforms like Codecademy, Khan Academy, and freeCodeCamp offer structured, step-by-step lessons that are perfect for beginners, so start there.
Once you’ve got a handle on the basics, apply what you’ve learned by building small projects. For example, try coding a simple calculator, a basic guessing game, or even a text-based story generator. These small projects will help you understand how programming concepts work together, giving you confidence and helping you identify areas where you might need a bit more practice.
When you're ready to move beyond the basics, Python offers many powerful libraries that open up new possibilities. Dive into pandas for data analysis, matplotlib for data visualization, or even Django if you want to explore web development. Each library offers a set of tools that helps you do more complex tasks, and learning them will expand your coding skillset significantly.
Keep practicing, and don't hesitate to look at code written by others to see how they approach problems. Coding is a journey, and with every line you write, you’re gaining valuable skills that will pay off in future projects.
FREE Python and R Programming Course on Data Science, Machine Learning, Data Analysis, and Data Visualization
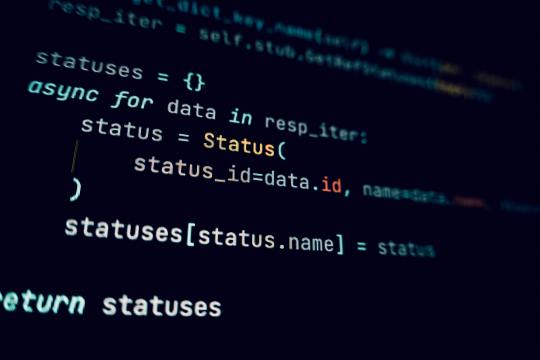
#learntocode#python for beginners#codingjourney#programmingbasics#web development#datascience#machinelearning#pythonprojects#codingcommunity#python#free course
10 notes
·
View notes
Text
Coding Update 6
I think its been a while since I've updated. I fell behind a little on my learning cause life has been really difficult lately.
Hope y'all had a good Thanksgiving and having a good start into the holiday season!! Yadda yadda more under the cut.
So I just finished Part 1 of my book. This mostly contained the introduction to Python, obviously, while learning a lot of the major functions of the program. I think it took me a bit to get into the swing of coding, especially cause it felt like I've had to rewire my own brain doing this haha.
The good news is I feel a LOT more comfortable with Python now. Not like "i can do anything!" yet but enough that it's actually super fun and I'm excited to work on projects!
The last part of the chapter taught me to use the "pytest" ability. I.E: writing test code so that I can make sure my programs are working properly and as intended. That part was really interesting, mostly because it was super duper busted at first for me.
That ended up being because where my "default folder" is set is like my main python hub, so i have to use the uh. What's it called? True access link? Where I write the entire string to the code's location.
Which also taught me that in the Terminal I have to use quotes for the location cause before I learned proper coding practices, I used spaces in some of the initial folders.
We're good now though.
The next part, Part II, is all about learning to build fully functional projects!! I'm so HYPED. There's four projects, of which it was like choose whatever you want! But I'm gonna start with an Alien Invasion remake. You know, the game where you're the little ship at the bottom shooting at aliens as they slowly decend on the screen. I should learn a lot from this one.
The other project I'm looking forward to is a simple online blog database. It'll have users create accounts, be accessible online, and you can make little journal posts! That should actually teach me a lot of stuff that I want to do.
There's another for data visualization, which I think I'll send to my cousin. He works in a lab at MIT and I know they use python for their programs. Maybe I can work my way into his work by doing that lmao.
Anyway, I'm really excited for all of this. It should teach me a ton of usable skills, and then i can add these projects to my portfolio to show off. Also I can spin off and make my own stuff.
Also also, if anyone wants to help me test my projects, feel free to let me know! I already know a few who are more than willing, but I'd appreciate any and all feedback as I go.
Oh! It also recommended learning version control, which I know almost nothing about. So I'll learn how to use GitHUB to store projects and recall old ones as I go if things break horribly. Which will be fun! Cause I know that for sure is going to be an important skill to have.
For a last fun fact, did you know places are like "requirements: typing 30 words per second." Do you know how fast I can type? At my peak I'm like over 110. I baseline at like 95. I don't know if that's actually fast but it makes me feel like the specialist little guy.
I hope you all have a good holiday season. Sorry no code in this post, I'm writing it so I can give you all an update, and I'm dog tired today. But but I promise to snip actual code for you as I go forward. And It'll be fun, especially cause this alien project will teach me about making VISUAL things!
Seasons Greasons Tumblr! -Kit
6 notes
·
View notes
Text
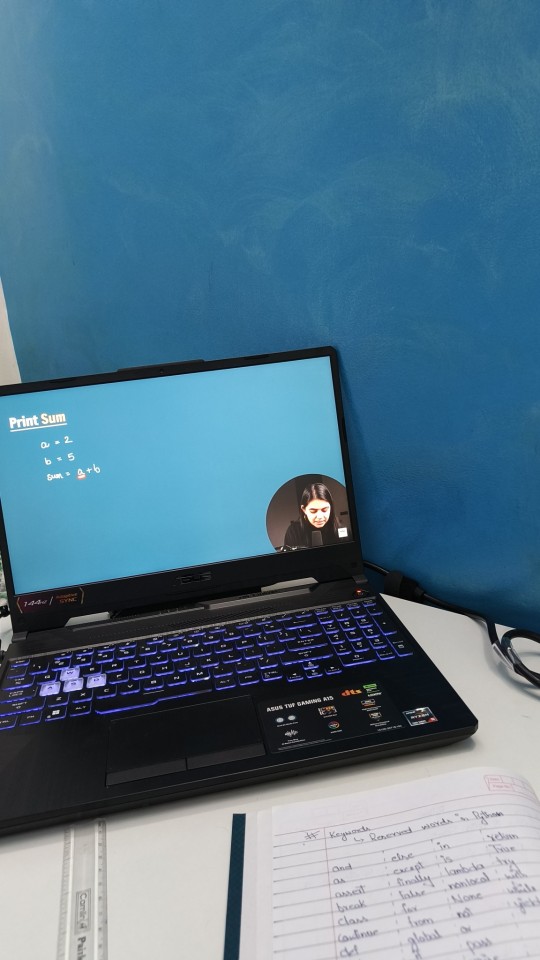

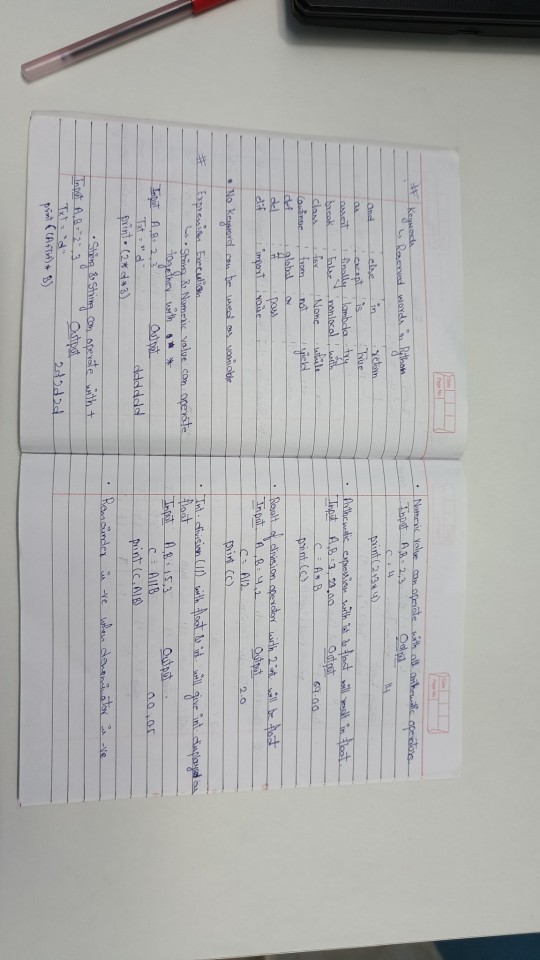


So I studied the basics about Python today, what are variables and the rules about it, what are data types and what are the different kinds of inputs, learned a lot about the conditional statements and I still have to work on my syntax because it kept throwing errors whenever I ran a conditional statement. In all had a great Day 1, looking forward to tomorrow and lesseee what happens.
18 notes
·
View notes
Text
Best Python Training in Marathahalli, Bangalore – Become a Python Expert & Launch a Future-Ready Career!
youtube
Want to master Python programming and build a successful IT career? Join eMexo Technologies for the Best Python Training in Marathahalli, Bangalore – your path to becoming a skilled Python developer with job-ready skills and industry certification.
Our Python Certification Course in Marathahalli, Bangalore is designed to equip you with in-demand programming skills, whether you're a beginner or an experienced professional. With real-time projects, hands-on exercises, and expert mentorship, you’ll gain the confidence to build real-world applications and secure your dream job.
🌟 Who Should Join Our Python Course in Marathahalli, Bangalore?
This Python Course in Marathahalli, Bangalore is ideal for:
Students and freshers looking to start their programming career
Software developers and IT professionals upskilling in Python
Data analysts and automation testers using Python for scripting
Anyone looking to crack technical interviews or get Python certified
📘 What You’ll Learn in Our Python Certification Course Marathahalli, Bangalore:
Core Python Programming: Variables, data types, loops, functions, OOP concepts
Advanced Python Concepts: File handling, exception handling, modules, decorators
Web Development with Python: Introduction to Django/Flask frameworks
Database Integration: Using Python with MySQL and SQLite
Automation & Scripting: Build scripts for real-time problem-solving
Live Projects: Real-world applications like calculators, dashboards, and web apps
🚀 Why Choose eMexo Technologies for Python Training in Marathahalli, Bangalore?
We are more than just a Python Training Center in Marathahalli, Bangalore – we are your learning partner. Our focus is on providing career-oriented Python training through certified instructors, hands-on practice, and real-time case studies.
What Makes Us the Best Python Training Institute in Marathahalli, Bangalore:
✅ Industry-expert trainers with real-world Python experience ✅ Fully-equipped classrooms and interactive online sessions ✅ 100% practical-oriented training with live project support ✅ Personalized career guidance, resume building & mock interviews ✅ Dedicated Python training placement in Marathahalli, Bangalore
📅 Upcoming Python Training Batch Details:
Start Date: July 1st, 2025
Time: 10:00 AM IST
Location: eMexo Technologies, Marathahalli, Bangalore
Mode: Both Classroom & Online Training Available
👥 Who Can Benefit from This Python Training Marathahalli, Bangalore?
Students & fresh graduates planning to enter the IT sector
Working professionals aiming to switch to Python development
Testers, analysts, and engineers looking to automate workflows
Anyone passionate about coding and application development
🎯 Get Certified. Get Placed. Get Ahead.
Join the top-rated Python Training Institute in Marathahalli, Bangalore and open doors to careers in software development, automation, web development, and data science.
📞 Call or WhatsApp: +91-9513216462 📧 Email: [email protected] 🌐 Website: https://www.emexotechnologies.com/courses/python-training-in-marathahalli-bangalore/
🚀 Limited Seats Available – Enroll Today and Start Your Python Journey!
🔖 Hashtags:
#PythonTrainingInMarathahalliBangalore#PythonCertificationCourseInMarathahalliBangalore#PythonCourseInMarathahalliBangalore#PythonTrainingCenterInMarathahalliBangalore#PythonTrainingInstituteInMarathahalliBangalore#eMexoTechnologies#PythonProjects#PythonTrainingPlacementInMarathahalliBangalore#ITTrainingBangalore#PythonJobs#BestPythonTrainingInstituteInMarathahalliBangalore#LearnPython#PythonProgramming#PythonForBeginners#Youtube
2 notes
·
View notes
Text
November 8th Journal
Looking through my to-do lists this month, I'm realizing that part of the reason why I'm not able to cross things off my list so easily is because the points I add to my list are fairly ambiguous and don't have a clear finish point. For instance, when I would write "learn binary trees on Python"; well what does that mean? Having actual actionable and completable items on my to-do list helps to better set daily goals for myself as well as to actually complete those goals. It often get intimidated seeing such large tasks that I didn't know how to approach so breaking those down for myself has made a world's difference. Yes, yes I'm a yapper.
To-do: 🪶Data course 4.3 🪶Data course 4.4 🪶Data course assignment 1 - unit 3 🪶Data course assignment 2 - unit 4 🪶Type out the rest of my notes 🪶Add visuals to my notes 🪶Finish text-based slot machine game 🪶Latin level 2 🪶Some data tasks for work
#study blog#studyblr#studyspo#study motivation#to do list#daily journal#dark academia#python#coding#language learning#light academia#langblr#study aesthetic#studying#self improvement
21 notes
·
View notes
Text
Best Python Training in Marathahalli, Bangalore – Become a Python Expert & Launch a Future-Ready Career!
youtube
Want to master Python programming and build a successful IT career? Join eMexo Technologies for the Best Python Training in Marathahalli, Bangalore – your path to becoming a skilled Python developer with job-ready skills and industry certification.
Our Python Certification Course in Marathahalli, Bangalore is designed to equip you with in-demand programming skills, whether you're a beginner or an experienced professional. With real-time projects, hands-on exercises, and expert mentorship, you’ll gain the confidence to build real-world applications and secure your dream job.
🌟 Who Should Join Our Python Course in Marathahalli, Bangalore?
This Python Course in Marathahalli, Bangalore is ideal for:
Students and freshers looking to start their programming career
Software developers and IT professionals upskilling in Python
Data analysts and automation testers using Python for scripting
Anyone looking to crack technical interviews or get Python certified
📘 What You’ll Learn in Our Python Certification Course Marathahalli, Bangalore:
Core Python Programming: Variables, data types, loops, functions, OOP concepts
Advanced Python Concepts: File handling, exception handling, modules, decorators
Web Development with Python: Introduction to Django/Flask frameworks
Database Integration: Using Python with MySQL and SQLite
Automation & Scripting: Build scripts for real-time problem-solving
Live Projects: Real-world applications like calculators, dashboards, and web apps
🚀 Why Choose eMexo Technologies for Python Training in Marathahalli, Bangalore?
We are more than just a Python Training Center in Marathahalli, Bangalore – we are your learning partner. Our focus is on providing career-oriented Python training through certified instructors, hands-on practice, and real-time case studies.
What Makes Us the Best Python Training Institute in Marathahalli, Bangalore:
✅ Industry-expert trainers with real-world Python experience ✅ Fully-equipped classrooms and interactive online sessions ✅ 100% practical-oriented training with live project support ✅ Personalized career guidance, resume building & mock interviews ✅ Dedicated Python training placement in Marathahalli, Bangalore
📅 Upcoming Python Training Batch Details:
Start Date: July 1st, 2025
Time: 10:00 AM IST
Location: eMexo Technologies, Marathahalli, Bangalore
Mode: Both Classroom & Online Training Available
👥 Who Can Benefit from This Python Training Marathahalli, Bangalore?
Students & fresh graduates planning to enter the IT sector
Working professionals aiming to switch to Python development
Testers, analysts, and engineers looking to automate workflows
Anyone passionate about coding and application development
🎯 Get Certified. Get Placed. Get Ahead.
Join the top-rated Python Training Institute in Marathahalli, Bangalore and open doors to careers in software development, automation, web development, and data science.
📞 Call or WhatsApp: +91-9513216462 📧 Email: [email protected] 🌐 Website: https://www.emexotechnologies.com/courses/python-training-in-marathahalli-bangalore/
🚀 Limited Seats Available – Enroll Today and Start Your Python Journey!
🔖 Hashtags:
#PythonTrainingInMarathahalliBangalore#PythonCertificationCourseInMarathahalliBangalore#PythonCourseInMarathahalliBangalore#PythonTrainingCenterInMarathahalliBangalore#PythonTrainingInstituteInMarathahalliBangalore#eMexoTechnologies#PythonProjects#PythonTrainingPlacementInMarathahalliBangalore#ITTrainingBangalore#PythonJobs#BestPythonTrainingInstituteInMarathahalliBangalore#LearnPython#PythonProgramming#PythonForBeginners#Youtube
2 notes
·
View notes
Text
Understanding Outliers in Machine Learning and Data Science
In machine learning and data science, an outlier is like a misfit in a dataset. It's a data point that stands out significantly from the rest of the data. Sometimes, these outliers are errors, while other times, they reveal something truly interesting about the data. Either way, handling outliers is a crucial step in the data preprocessing stage. If left unchecked, they can skew your analysis and even mess up your machine learning models.
In this article, we will dive into:
1. What outliers are and why they matter.
2. How to detect and remove outliers using the Interquartile Range (IQR) method.
3. Using the Z-score method for outlier detection and removal.
4. How the Percentile Method and Winsorization techniques can help handle outliers.
This guide will explain each method in simple terms with Python code examples so that even beginners can follow along.
1. What Are Outliers?
An outlier is a data point that lies far outside the range of most other values in your dataset. For example, in a list of incomes, most people might earn between $30,000 and $70,000, but someone earning $5,000,000 would be an outlier.
Why Are Outliers Important?
Outliers can be problematic or insightful:
Problematic Outliers: Errors in data entry, sensor faults, or sampling issues.
Insightful Outliers: They might indicate fraud, unusual trends, or new patterns.
Types of Outliers
1. Univariate Outliers: These are extreme values in a single variable.
Example: A temperature of 300°F in a dataset about room temperatures.
2. Multivariate Outliers: These involve unusual combinations of values in multiple variables.
Example: A person with an unusually high income but a very low age.
3. Contextual Outliers: These depend on the context.
Example: A high temperature in winter might be an outlier, but not in summer.
2. Outlier Detection and Removal Using the IQR Method
The Interquartile Range (IQR) method is one of the simplest ways to detect outliers. It works by identifying the middle 50% of your data and marking anything that falls far outside this range as an outlier.
Steps:
1. Calculate the 25th percentile (Q1) and 75th percentile (Q3) of your data.
2. Compute the IQR:
{IQR} = Q3 - Q1
Q1 - 1.5 \times \text{IQR}
Q3 + 1.5 \times \text{IQR} ] 4. Anything below the lower bound or above the upper bound is an outlier.
Python Example:
import pandas as pd
# Sample dataset
data = {'Values': [12, 14, 18, 22, 25, 28, 32, 95, 100]}
df = pd.DataFrame(data)
# Calculate Q1, Q3, and IQR
Q1 = df['Values'].quantile(0.25)
Q3 = df['Values'].quantile(0.75)
IQR = Q3 - Q1
# Define the bounds
lower_bound = Q1 - 1.5 * IQR
upper_bound = Q3 + 1.5 * IQR
# Identify and remove outliers
outliers = df[(df['Values'] < lower_bound) | (df['Values'] > upper_bound)]
print("Outliers:\n", outliers)
filtered_data = df[(df['Values'] >= lower_bound) & (df['Values'] <= upper_bound)]
print("Filtered Data:\n", filtered_data)
Key Points:
The IQR method is great for univariate datasets.
It works well when the data isn’t skewed or heavily distributed.
3. Outlier Detection and Removal Using the Z-Score Method
The Z-score method measures how far a data point is from the mean, in terms of standard deviations. If a Z-score is greater than a certain threshold (commonly 3 or -3), it is considered an outlier.
Formula:
Z = \frac{(X - \mu)}{\sigma}
is the data point,
is the mean of the dataset,
is the standard deviation.
Python Example:
import numpy as np
# Sample dataset
data = {'Values': [12, 14, 18, 22, 25, 28, 32, 95, 100]}
df = pd.DataFrame(data)
# Calculate mean and standard deviation
mean = df['Values'].mean()
std_dev = df['Values'].std()
# Compute Z-scores
df['Z-Score'] = (df['Values'] - mean) / std_dev
# Identify and remove outliers
threshold = 3
outliers = df[(df['Z-Score'] > threshold) | (df['Z-Score'] < -threshold)]
print("Outliers:\n", outliers)
filtered_data = df[(df['Z-Score'] <= threshold) & (df['Z-Score'] >= -threshold)]
print("Filtered Data:\n", filtered_data)
Key Points:
The Z-score method assumes the data follows a normal distribution.
It may not work well with skewed datasets.
4. Outlier Detection Using the Percentile Method and Winsorization
Percentile Method:
In the percentile method, we define a lower percentile (e.g., 1st percentile) and an upper percentile (e.g., 99th percentile). Any value outside this range is treated as an outlier.
Winsorization:
Winsorization is a technique where outliers are not removed but replaced with the nearest acceptable value.
Python Example:
from scipy.stats.mstats import winsorize
import numpy as np
Sample data
data = [12, 14, 18, 22, 25, 28, 32, 95, 100]
Calculate percentiles
lower_percentile = np.percentile(data, 1)
upper_percentile = np.percentile(data, 99)
Identify outliers
outliers = [x for x in data if x < lower_percentile or x > upper_percentile]
print("Outliers:", outliers)
# Apply Winsorization
winsorized_data = winsorize(data, limits=[0.01, 0.01])
print("Winsorized Data:", list(winsorized_data))
Key Points:
Percentile and Winsorization methods are useful for skewed data.
Winsorization is preferred when data integrity must be preserved.
Final Thoughts
Outliers can be tricky, but understanding how to detect and handle them is a key skill in machine learning and data science. Whether you use the IQR method, Z-score, or Wins
orization, always tailor your approach to the specific dataset you’re working with.
By mastering these techniques, you’ll be able to clean your data effectively and improve the accuracy of your models.
#science#skills#programming#bigdata#books#machinelearning#artificial intelligence#python#machine learning#data centers#outliers#big data#data analysis#data analytics#data scientist#database#datascience#data
4 notes
·
View notes