#- Queue: PriorityQueue
Explore tagged Tumblr posts
Text
JavaCollections: Your Data, Your Way
Master the art of data structures:
List: ArrayList, LinkedList
Set: HashSet, TreeSet
Queue: PriorityQueue, Deque
Map: HashMap, TreeMap
Pro tips:
Use generics for type safety
Choose the right collection for your needs
Leverage stream API for elegant data processing
Collections: Because arrays are so last century.
#JavaCollections: Your Data#Your Way#Master the art of data structures:#- List: ArrayList#LinkedList#- Set: HashSet#TreeSet#- Queue: PriorityQueue#Deque#- Map: HashMap#TreeMap#Pro tips:#- Use generics for type safety#- Choose the right collection for your needs#- Leverage stream API for elegant data processing#Collections: Because arrays are so last century.#JavaProgramming#DataStructures#CodingEfficiency
2 notes
·
View notes
Text
📚 Comparing Java Collections: Which Data Structure Should You Use?
If you're diving into Core Java, one thing you'll definitely bump into is the Java Collections Framework. From storing a list of names to mapping users with IDs, collections are everywhere. But with all the options like List, Set, Map, and Queue—how do you know which one to pick? 🤯
Don’t worry, I’ve got you covered. Let’s break it down in simple terms, so you can make smart choices for your next Java project.
🔍 What Are Java Collections, Anyway?
The Java Collection Framework is like a big toolbox. Each tool (or data structure) helps you organize and manage your data in a specific way.
Here's the quick lowdown:
List – Ordered, allows duplicates
Set – Unordered, no duplicates
Map – Key-value pairs, keys are unique
Queue – First-In-First-Out (FIFO), or by priority
📌 When to Use What? Let’s Compare!
📝 List – Perfect for Ordered Data
Wanna keep things in order and allow duplicates? Go with a List.
Popular Types:
ArrayList – Fast for reading, not so much for deleting/inserting
LinkedList – Good for frequent insert/delete
Vector – Thread-safe but kinda slow
Stack – Classic LIFO (Last In, First Out)
Use it when:
You want to access elements by index
Duplicates are allowed
Order matters
Code Snippet:
java
🚫 Set – When You Want Only Unique Stuff
No duplicates allowed here! A Set is your go-to when you want clean, unique data.
Popular Types:
HashSet – Super fast, no order
LinkedHashSet – Keeps order
TreeSet – Sorted, but a bit slower
Use it when:
You care about uniqueness
You don’t mind the order (unless using LinkedHashSet)
You want to avoid duplication issues
Code Snippet:
java
🧭 Map – Key-Value Power Couple
Think of a Map like a dictionary. You look up values by their unique keys.
Popular Types:
HashMap – Fastest, not ordered
LinkedHashMap – Keeps insertion order
TreeMap – Sorted keys
ConcurrentHashMap – Thread-safe (great for multi-threaded apps)
Use it when:
You need to pair keys with values
You want fast data retrieval by key
Each key should be unique
Code Snippet:
java
⏳ Queue – For First-Come-First-Serve Vibes
Need to process tasks or requests in order? Use a Queue. It follows FIFO, unless you're working with priorities.
Popular Types:
LinkedList (as Queue) – Classic FIFO
PriorityQueue – Sorted based on priority
ArrayDeque – No capacity limit, faster than LinkedList
ConcurrentLinkedQueue – Thread-safe version
Use it when:
You’re dealing with task scheduling
You want elements processed in the order they come
You need to simulate real-life queues (like print jobs or tasks)
Code Snippet:
java
🧠 Cheat Sheet: Pick Your Collection Wisely
⚙️ Performance Talk: Behind the Scenes
💡 Real-Life Use Cases
Use ArrayList for menu options or dynamic lists.
Use HashSet for email lists to avoid duplicates.
Use HashMap for storing user profiles with IDs.
Use Queue for task managers or background jobs.
🚀 Final Thoughts: Choose Smart, Code Smarter
When you're working with Java Collections, there’s no one-size-fits-all. Pick your structure based on:
What kind of data you’re working with
Whether duplicates or order matter
Performance needs
The better you match the collection to your use case, the cleaner and faster your code will be. Simple as that. 💥
Got questions? Or maybe a favorite Java collection of your own? Drop a comment or reblog and let’s chat! ☕💻
If you'd like me to write a follow-up on concurrent collections, sorting algorithms, or Java 21 updates, just say the word!
✌️ Keep coding, keep learning! For More Info : Core Java Training in KPHB For UpComing Batches : https://linktr.ee/NIT_Training
#Java#CoreJava#JavaProgramming#JavaCollections#DataStructures#CodingTips#DeveloperLife#LearnJava#ProgrammingBlog#TechBlog#SoftwareEngineering#JavaTutorial#CodeNewbie#JavaList#JavaSet#JavaMap#JavaQueue#CleanCode#ObjectOrientedProgramming#BackendDevelopment#ProgrammingBasics
0 notes
Text
The Ultimate Guide to Java Collection
Java libraries are indispensable tools that streamline development by providing pre-written code for common tasks. "The Ultimate Guide to Java Libraries" explores a myriad of libraries that enhance Java programming, from handling data structures to implementing complex algorithms.
A key feature covered is collections in Java, which offer efficient ways to manage groups of objects, improving code efficiency and readability.
TpointTech is a valuable resource for developers seeking in-depth tutorials and examples on using these libraries effectively. Leveraging these libraries can significantly reduce development time and improve application performance.
Overview of Java Collections
The Java Collections Framework includes interfaces, implementations, and algorithms. The core interfaces include Collection, List, Set, Queue, and Map, each serving different purposes.
Collection Interface:
The root interface of the framework, representing a group of objects known as elements. It is extended by List, Set, and Queue interfaces.
List Interface:
An ordered collection that allows duplicate elements. Common implementations are ArrayList, LinkedList, and Vector. Lists are ideal when you need to access elements by their index.
ArrayList: Resizable array implementation, offering constant-time positional access but slower for insertion and deletion.
LinkedList: Doubly-linked list implementation, providing efficient insertion and deletion but slower access time.
Vector: Synchronized version of ArrayList, rarely used due to performance overhead.
Set Interface:
A collection that does not allow duplicate elements. It models mathematical sets and provides implementations like HashSet, LinkedHashSet, and TreeSet.
HashSet: Uses a hash table for storage, offering constant-time performance for basic operations.
LinkedHashSet: Maintains insertion order, slightly slower than HashSet.
TreeSet: Implements the SortedSet interface, ensuring elements are in ascending order, based on their natural ordering or a specified comparator.
Queue Interface:
Designed for holding elements prior to processing, typically ordered in a FIFO (first-in-first-out) manner. Common implementations include LinkedList, PriorityQueue, and ArrayDeque.
PriorityQueue: Elements are ordered according to their natural ordering or a provided comparator, useful for creating priority-based tasks.
ArrayDeque: Resizable-array implementation of the Deque interface, providing efficient insertion and deletion from both ends.
Map Interface:
Represents a collection of key-value pairs, where each key maps to one value. Popular implementations are HashMap, LinkedHashMap, and TreeMap.
HashMap: Provides constant-time performance for basic operations, assuming a good hash function.
LinkedHashMap: Maintains a doubly-linked list of its entries, preserving the order of insertion.
TreeMap: Implements the SortedMap interface, ensuring keys are in ascending order.
Advantages of Java Collections Framework
Reduces Programming Effort: With a set of ready-made data structures and algorithms, JCF eliminates the need for developers to implement complex data structures from scratch.
Increases Program Speed and Quality: Standardized interfaces and optimized implementations ensure high performance and reliability.
Interoperability: Collections can be easily passed across APIs, reducing the complexity of integration.
Ease of Maintenance: Well-documented and widely-used classes make it easier for developers to maintain and enhance code.
Common Algorithms in JCF
Java Collections Framework includes various algorithms to perform routine tasks, such as sorting, searching, and shuffling. These algorithms are static methods in the Collections utility class.
Sorting: Collections.sort(List list), sorts the specified list into ascending order.
Shuffling: Collections.shuffle(List list), randomly permutes the elements in the list.
Searching: Collections.binarySearch(List> list, T key), performs binary search on a sorted list.
Conclusion
The Java Collections Framework is indispensable for any Java developer. It offers a standardized and efficient way to manage groups of objects, making code more robust and maintainable.
By leveraging the various interfaces and implementations, such as lists, sets, queues, and maps, developers can handle data structures effectively.
Understanding collections in Java, as detailed on resources like TpointTech, is crucial for building high-performance applications. Whether you're a beginner or an experienced developer, mastering Java collections will significantly enhance your programming capabilities.
0 notes
Text
Unraveling the Power of Java Collections Framework and Interfaces
In the realm of Java programming, understanding the intricacies of the Java Collections Framework and Interfaces is pivotal for building robust and efficient applications. Let's delve into the essentials, including Java PriorityQueue and sets in Java, to harness the full potential of these foundational components.
Java Collections Framework: A Comprehensive Toolbox
The Java Collections Framework is a powerhouse of data structures and algorithms, offering a versatile toolbox for developers. It provides interfaces and classes for managing and manipulating groups of objects, ensuring flexibility and efficiency in Java programming.
Java Interfaces: Enabling Polymorphism and Abstraction
Java Interfaces play a crucial role in achieving polymorphism and abstraction in programming. By defining a set of methods that implementing classes must adhere to, interfaces allow developers to create flexible and interchangeable components within their codebase.
Java PriorityQueue: Prioritizing Efficiency
Java PriorityQueue, a class within the Collections Framework, stands out as a specialized queue implementation. It orders elements based on their natural ordering or according to a specified comparator, enabling developers to prioritize and efficiently manage tasks in their applications.
Sets in Java: Uniqueness and Order
Sets in Java, a part of the Collections Framework, ensure uniqueness of elements within a collection. Whether using HashSet, TreeSet, or LinkedHashSet, developers can leverage sets to manage distinct elements and, in some cases, maintain a specific order.
Conclusion: Elevating Java Programming Proficiency
Mastering the Java Collections Framework, Java Interfaces, PriorityQueue, and sets in Java empowers developers to create scalable and well-organized applications. These foundational concepts not only streamline data management but also enhance the overall efficiency and maintainability of Java code. As you explore these elements, you unlock the potential to elevate your proficiency in Java programming, creating more robust and sophisticated software solutions.
1 note
·
View note
Text

BFS . . . . for more information http://bit.ly/3TLpfGJ check the above link
#spanningtree#avltree#binarytree#Btree#bplustree#binarysearchtree#treedatastructure#linkedlist#array#circularlist#circularlinkedlist#circulardoublylinkedlist#datastructure#computer#algorithm#computerscience#stack#queue#priorityqueue#fifo#lifo#computerengineering#javatpoint
0 notes
Text
2b2t history, by its wiki
2010
Player Count: 50-70 (Accounts vary)
Nothing is much known about the early days of 2b2t, except that the server began in December of 2010. Some that do claim to have joined during this time say that there was nothing spectacular on the server, and that by then, spawn was already a mess.
2011
Player Count: 50-70
During 2011, a lot of things happened. The largest one being the formation of the Facepunch Republic. 2011 is also when many now famous oldfags joined, like Offtopia, xcc2 and popbob.
Early 2011 - Hausemaster teleports to random players to see the progress of his server. Sometimes he would give random items.
April 2011 - Facepunch Republic begins, 4channers take notice of this and tries to stop them.
May 2011 - Player data corruption and change of world generation, causes spawn to look weird.
July 2011 - First post of the 2b2t Comic by Judge Holden
December 2011 - January 2012 - 2b2t gets backdoored by popbob and co.
2011 - The first dupe on 2b2t, the Piston Dupe, was discovered by someone in Facepunch. This involved pushing the piston real fast to duplicate items.
2012
Player Count: 20-50
This year marked one of the most barren times on the server. The server faced drastic player loss during this time due to several facepunchers leaving, the IP changing, and the server being down for three months, causing players to believe that the server shut down for good.
February 2012 - Facepunch Republic ends
February 2012 - Judge's group base at -1 million -1 million is griefed by x0XP.
April 2012 - ImperatorTerrae finds Viking base and 'reforms' it.
June 2012 - 2k2k is founded by the Zion project
2b2t is shut down for three months and changes the IP address from 2b2t.net to 2b2t.org
2013
Player Count: 10-30
2013 is mostly painted by Valkyria, as most other groups of this time period are all but forgotten
February 2013 - Hitlerwood is founded by Coldwave, Zach3397 and Hinderjd
March 2013 - Anatolia is founded by Sato86, Drewbookman and PyroByte
April 2013 - Valkyria is founded by the members of Hitlerwood and Anatolia (Black Flag Group)
May 2013 - 1.4.7 Dupe, The End Dragon is slain
(Unknown month) 2013, Construction of HardHat's Pyramid has started.
June 2013 - 1st Incursion, IGN video (does not generate hype)
August - October 2013 - 2nd Incursion
October/November 2013 - Shenandoah is founded by jesse_number6
December 2013 - 2b2t gets backdoored, Pyrobyte and iTristan have reached the World Border
2014
Player Count: 30-20
During 2014, Valkyria had broken up into a few smaller groups. These include Gape group and the Legion of Shenandoah
January 2014 - Asgard is abandoned after being griefed by popbob during construction
May 2014 - 2k2k is buried in lavacasts and many ancient bases are annihilated by Withers (Including the NFE and Plugin Town)
June 2014 - Legion of Shenandoah begins and Gape 1 is founded
July 2014 - Infamous message shows up on the server website stating that Hausemaster has left the server forever, leaving it in the care of "an anonymous friend." The owner's personality changes immediately after this post.
September 2014 - Fenrir Project begins
October 2014 - Nova is founded in 3b3t
December 2014 - Southern Canal Project is started by ThebesAndSound
2015
Player Count: 20-40
A large population boost happened during this year, mostly from the numerous articles and a massive Reddit post.
January 2015 - 2b2t is updated to 1.8
February 2015 - Fenrir Project ends
March 2015 - Asgard II is founded
April 2015 - Asgard II is destroyed, the 3rd Incursion begins
May 2015
June 2015
August 2015
October 2015
December 2015
Aureus is founded
3rd Incursion ends
Wrath constructed
Kings Landing is founded
The last Facepunch thread closes
Vortex Coalition founded
Project Vault, (now known is the 2b2t museum), is a project determined to keep every single monument in 2b2t untouched and left as a museum to other players, begins.
Motherboard Article is released
VICE mini incursion begins and ends
Imperator's Base is destroyed by Jared2013. Around this time, the server website shuts down.
2016
Player Count: 10-250
This is when 2b2t was most densely populated. This was caused when TheCampingRusher made a video and posted it onto Youtube
February 2016
March 2016
April 2016
RPS Article released
BedTP Exploit surfaces
Space Valkyria destroyed by the Tyranny
KGB destroyed by the Tyranny
Land of Norphs destroyed by the Tyranny
May 2016
June 2016
July 2016
August 2016
September 2016
October 2016
The player count has plummeted, sometimes as low as 1-5 players online.
June 1st - Rusher begins his series on 2b2t. In the coming days, 2b2t becomes flooded with his fans.
June 4th - Rusher declares war on "OGs," a reference to players who have been on the server longer than him.
June 13th - Fit becomes "leader" of Team Veteran, which is supposedly meant to contain the rusher onslaught and preserve the server's pre-rusher culture. This, of course, is debunked later.
June - A lava wall is made around the 500x500 area of spawn, in hopes of keeping Rushers trapped in spawn; this project fails. A very similar project occurs during the 6th Incursion, but fails aswell.
June 17th - Omega City Founded
July 24th - The Fourth Incursion begins with the Veterans raiding Napkin0fTruth's base.
July 28th - 2nd largest battle in 2b2t history (19 players)
July 31st - Aureus is destroyed by the base members themselves.
July - Hardware upgrade, temporary map
The Queue is set up around this time.
August 5th - Fit versus Rusher duel, Fit wins by having more golden apples.
August 19th - Torogadude gets stripped of his priority queue.
September 16th - Largest Battle in 2b2t History: Team Veteran allies with CorruptedUnicorn to take down Rusher. The battle ends in a draw, and Rusher is able to escape.
September 19th - Rusher leaves 2b2t for the first time for about a month.
September 22th - Valley of Wheat destroyed
September - 4th Incursion ends, Newsweek Article released
October 9th - The Rusher War ends. The Veterans say that about 10,400 Rushers had been killed, but this number is frequently questioned.
October 15th -TheCampingRusher returns and makes 3 more videos on 2b2t before he leaves.
October 22nd - Rusher leaves and The Rusher War officially ends. A small presence of rushers/newfags remain, and the server has been radically changed.
October - Offtopia's Drain is destroyed after FitMC made a tour of his base.
November 2016
December 2016
November 11th - 11/11 Dupe leaked to public
November 22nd - Jonathan5454 starts 6Garden. Major construction started sometime in January 2017.
November 25th - AgentGB makes a video on 2b2t. However, he does not make a video afterwards, and states that 2b2t won't be a series.
November 28th - The Fifth Incursion is organized by jared2013, however this fails, and barely makes any attention, and now recognized as a meme.
New coord exploit. Fitlantis is found.
Large battle in Fitlantis
2b2t is updated to 1.11, ending the 11/11 Dupe
AntVenom considers making a video on the history of 2b2t. He asks for the consent of the playerbase and the "admins".
Byrnsy begins his journey to the 2b2t World Border, with hopes of completing the +x Nether Highway. The Nether Highway Expedition group is formed.
The Emperium is founded by TheDark_Emperor.
Player count drops to the low 50s before Fit starts making his videos.
2017
Player Count: 80-200 (50-100 during Temp Maps)
This year saw massive decay of the rusher population and numerous other things
January 2017
February 2017
March 2017
April 2017
May 2017
June 2017
July 2017
August 2017
September 2017
January 1st - Fit returns to 2b2t, with new jewtuber, kid friendly content (starting with minecraft world records by boat). The impact from this has kept the 2b2t playerbase in its 200s, as many players join from Fit each day.
Construction of Block Game Mecca starts
Mid January - Spawn Masons are founded
Late January - The Vortex Coalition gets "disbanded".
HermeticLock's Spawn Base is constructed and The Third Largest Battle of 2b2t History occurs there. The base was constantly rebuilt and lavacasted over and over
15 milliontown is built by the nether highway group
Highland is founded by The_Grand_Lotus
Toro's Theater begins construction
2b2t logo rebranded
Kinorana hacks all maps in 2b2t. Protests against Kinorana begin.
SpawnMasons founded by Hermeticlock.
Early March - The 4th Reich is disbanded
March 3th - Maps are reset and old maps are deleted off 2b2t. The deathspam protest takes a short ceasefire.
March 15th - The Donkey Dupe is leaked to public.
March 18th - The Donkey Dupe has been patched.
March 21st - Wintermelon is griefed
March 22nd - fr1kin discovers an exploit that can bring the server below 1tps. This had normal attention until Fit claims that the lag could crash the server.
March 26th - World Border reached legitimately through the +X Highway. The +X Highway has been completed. Point Nemo is founded.
March 29th - Point Nemo is griefed
March - A player by the name of PriorityQueue is seen at spawn floating and not taking any damage, it was initially thought that he backdoored the server, however this was later proved to be incorrect (possibly the usage of MineChat).
April 1st - 2017 April fools map. This lasted a few days. The queue was also disabled during the uptime of the map
April 9th - Apocalypse Exploit patched, /kill temporarily disabled
April 13th - 24th - Fit's journey to the World Border
May 18th - Fit visits Point Dory without the permission of its builders.
May 20th - Point Dory is griefed
May 25th - Torogadude leaks the coordinates of his own theater by accident. It got destroyed almost instantly.
June 1st - 1st Anniversary of Rusher's Invasion in 2b2t. 2b2t is flooded with lag machines and various shitposts to celebrate the anniversary.
June 4th - Battle between Spawn Masons and Peacekeepers starts.
June 9th - The +Z Highway is completed to the world border.
June 18th - Omega City is destroyed.
June 23rd - The first server crash
June 29th - HermeticLock announced the "second season" of hanging around at his spawnbase
July 6th - The second server crash
July 6th - 7th - Server shut down, as a preparation for the 1.12 update and the addition of new plugins
July 8th- 22th - 1.12 tempmap
July 23th - The original map returns. The owner announces the deletion of all non-vanilla items. However, he accidentally deleted Axe enchantments, player heads, efficiency on shears, and all dungeon spawners. The 1.12 dupe (also known as the 7/21 dupe), was also discovered, which involved spamming in the crafting book
July 24th - Dupe patched sometime around 10:00 AM (EST).
July 28th - Summermelon griefed by Fit and his crew.
July 29th - Fit is exposed by Offtopia in the 2b2t Subreddit for making stuff up just to get a reason to grief Summermelon. The real reason might have involved his youtube career to get money. Fit does this again in 2018 at offtopia's base, Viper.
August 1st - Server restarted by the owner twice at 12:00 AM August 1st (EST)
August 2nd - The 3rd Major crash causes 2b2t down for a while. (Most likely low tps)
August 4th - Jared2013 obtains bureaucrat permissions through Niftyrobo on the 2b2t wiki, and destroys the wiki.
August 5th - Armorsmith lags the server down to 1tps. Causing the playercount to drop to 50-80 players. Queue virtualy dosen't exist.
August 6th - Riot on the wiki and subreddit to demote jared2013 and niftyrobo for the removal of pages with newfag history and several newfag bases such as 6Garden.
August 7th - Wiki is restored and Jared2013 and Niftyrobo has been banned globally from entering FANDOM Wikia itself. The subreddit explodes with wiki rants due to the new admin team consisting of newfags. The new founder, DarkAnhilator, has gathered a source team, and the subreddit calms down.
September 15th - A Spanish YouTuber, ElRichMC makes a video on the server, causing his fans to join and spam the chat in Spanish. Despite the video being quite popular, ElRichMC decides not to make another video and the server returns to normal in a matter of a few weeks.
Mid September - Project Obsidian Sky is completed. The 500x500 region of spawn now has an obsidian roof at Y256. (Today in Mid 2018, most of the obsidian has been destroyed by withers).
Late September - Elytras are disabled due to large amounts of chunks being loaded in a short amount of time, causing both lag and the server file size to grow much too quickly. Elytras have been disabled for almost an entire year. The SOON™ meme appears all over the subreddit because of this.
October 2017
November 2017
December 2017
October 13th - The owner announces removal of pre - June 1st queue.
October 13th - 20th - Bots fill the server in a vain attempt to kill it off, Drachenstein buys oldfags 2 months of priority
November 19th - 20th A redditor named PM_ME_YOUR_TITS_GIRL posted a render of 2b2t on r/gaming and r/minecraft
December 19th - Construction on the Cerulean Islands begins
December 20th - Astral Order is founded after the Purge of Eden
December 21st - VoCo declares the "Vo-Perium" war
December 23rd - X- Digger Team reaches the world border.
December 25th - Armorsmith and Co. planned to lag the server to 0 tps, and succeded to get it down to 0.5 before the owner personally destroyed the machine.
2018
Player Count: 100-250
This year is most known for two incursions (6th and 7th) and the grief of the BGM
January 2018
February 2018
March 2018
April 2018
May 2018
January 1st - Team Inferno reforms into Infrared after all the insiding and griefing
January 10th - United Group Embassy is formed, an initial 15 groups joining.
January 11th - "Vo-Perium" concludes after very little ground is gained. Emperium and VoCo work on building positive relations.
February 4th - Offtopia's Viper Base griefed, led by Fit. Several more subreddit rants occur from this.
February 7th - The 2b2t Media Archive is founded by xyzvp
February 9th - Up to 17 U.G.E. representatives arrive at spawn and begin renovating.
February 13th - 16 U.G.E. representatives fight 4 members of Infrared and Highland at spawn. Renovations continue.
March 20th - Temp map begins while the main map is being transferred from a 4 Terabyte HDD to a 6 TB SSD[1], as it was rapidly approaching the upper size limit of its current hardware. Server traffic is lower than usual but still high. TPS range is around 10 to 13.
March 27th - Main map back up
March 28th - Severe rubberband and lag occours at high TPS as well as several plugins being removed temporarily which allowed for people to use "illegal items" for a short amount of time, as well as being able to use the nether roof while taking heavy amounts of damage.
March 31th - April 2nd - An unnamed April Fools' tempmap starts on March 31st, 2018, and ends April 2nd, 2018. During this period, AntVenom confirms he will be releasing his video on April 3rd. The Sixth Incursion is declared by Sato and the ender dragon on the temp map is killed by the new incursion.
April 2nd - 6th - 2nd tempmap after failure of reverting back the world.
April 3rd - The highly anticipated "Face of Minecraft Anarchy" video is released by AntVenom. New players flood the server. Total player counts reach about 350 at the most.
April 6th - The main map, with very bad timing, comes back online[1] and the owner adds AAC to the server, which causes frequent disconnects and problems in general.
April 12th - 14 million subscriber YouTuber PopularMMOs is seen logging onto the server and is geared by an unknown player. He is later seen at nether spawn and killed with an end crystal. He claims he is not making a video on the server, however not everyone is convinced.
April 16th - 24th - The Obsidian Wall, a wall surrounding 1000 cubic meters of spawn is constructed and finished by Sixth Incursion members. It is named the largest community project ever completed in server history. To get the obsidian necessary , "gulag" slave camps were set up around spawn and new players were captured and forced to mine enderchests to obtain obsidian. This is the first confirmed time slave labor is used in the server's history to complete a community project.
April 26th - The obsidian wall ends up being a failure due to the fact that players are dedicated enough to spend 7 minutes breaking 2 obsidian blocks. Spawn patrolling became a meme after the incursion members became so self-absorbed after the wall was built. Much of the wall got destroyed however it is still very recognizable in most places.
April 29th - The 2b2t Archaeological Society is founded by /r/ryanfortner3333
May 1st - Jared2013 starts "31Days31Greifs" which causes widespread damage across the server
May 3rd - Sixth Incursion ends and is deemed a success.
May 5th - Construction of Mokuzai Island starts.
May 10th - FitMC reveals secrets about the Age of Tyrrany to the general public.
May 18th - Empire's Edge, Emperium's main base, is leaked to the subreddit by an unknown user and the Emperium quickly removes their belongings and destroys the base before anyone else could.
May 25th - The Builders Haven project is founded by Beardler and managed by Jonathan5454
May 28th - All maps on the server are cleared in an attempt to stop more bases from being griefed in any way they might be. The Map Artists of 2b2t claim responsibility for it and most are understanding.
May 30th - The Boedecken, a large base kept secret for nearly two years, is greifed. FitMC makes base tour shortly after. jared2013 did not claim responsibility for the base's greif.
May 31st -
June 2018
July 2018
August 2018
September 2018
October 2018
November 2018
December 2018
June 1st - One of the largest temp-bases on 2b2t, Purgatory was griefed.
June 1st - 700k Spanish-speaking YouTuber TheDaarickPartner starts a series on 2b2t.
June 1st - Second anniversary of Rusher War.
June 1st - The largest base ever built in the server's history and possibly in SMP Minecraft, Block Game Mecca, is greifed. The base members put the blame on Beardler, one of the members of the base. (The basemates later took this back, claiming that Beardler's account was hacked due to negligence, and Beardler had no intent to leak)
June 4th - 65k YouTuber BenMascott makes a video on the server, goes mostly unnoticed
June 7th - Server reaches 50,000 in-game days
June 10th - Large lag spike that lasts for 3 hours, TPS remains 0.6 for the whole time.
June 16th - Purgatory 2, the largest temp-base on 2b2t, was griefed as a revenge act against Beardlers actions.
June 16th - Beardler quits 2b2t, and Block Game Mecca/Boedecken drama ends.
Mid July - Third Donkey Dupe is discovered
July 24th, 2018 - 2b2t wikia hosted on FANDOM was deleted and moved to Miraheze
Mid August - The servers economy is at a point that anything is worthless, including Hacked Items
August 21st - Infrared disbands for the first time
August 22nd - Builders Haven is greifed and disbanded
September 20th - FuzeIII joins 2b2t and begins a series
September 21st - The Seventh Incursion begins
September 21st - Third Donkey Dupe is patched
Sepetember 27th - FuzeIII declares the foundation of Team Baguette and "War on Team Veteran and the Americans"
October 8th - jared2013 is demoted from head of the incursion due to internal drama.
October 13th - The Seventh Incursion is called off by Henry due to lack of leadership initiative. Drama posts on reddit follow soon after.
October 13th - jared2013 makes a new discord and an Incursion revival initiative to complete original goals of the Incursion.
October 30th - jared2013's Incursion Reloaded revival officially ends a day earlier than initial deadline of the 31st due to an inside attempt on the Emperium. This caused jared's discord to be deleted.
October 31st - A 2b2t halloween party was hosted, only lasting for 4 hours
October - Fit's relevancy is questioned after being kicked out of the Seventh Incursion (see Spawn Incursions)
October - New "banning" method involving max filled books with unicode is discovered. Players are "permanently" banned from the server if they are given a certain amount of these books, which will kick them out of the server due to an overflow in packets registered by the server, unless they e-mail Hausemaster to clear their inventories. The phenomena is known as "book banning".
November 7th - 9th - Beardler returns to 2b2t for 2 days and claims he will return in Mid/Late 2019
November 11th - New book related dupe discovered. Dupe causes lag spikes when performed due to constant chunk reloading.
November 17th - BGM (Block Game Mecca) was found to consist of mostly schematic builds, which put an impact on the bases outlook and originality.
November 23rd - 1.13 is announced for 2b2t coming “soon”
November - The 2b2t Wiki is 2 years old
November - Disbanded Infrared is reformed into Iridium
November 29th - The FuzeIII vs Fit duel occurs, Fit "wins".
November 30th - The 2b2t wiki gains public attention due to FuzeIII mentioning the wiki and the general miraheze discord noticing 2b2t. This wiki achieved a record high on Thursday of 900+ views
December 19th - Queue is glitched and drops to 0, resulting in the server being unable to join
December 23rd - Coordinates for planned Christmas party is leaked, chaos ensues.
December 27th - Iridium is disbanded
December - General lack of major activity due to Queue bugs, connection errors, and lag
December - U.G.E is dissolved after long period of inactivity
2019
Player Count: 100-250
"It's the Current Year!"
January 2019
February 2019
January - Lag and queue issues are getting worse.
January 12th - Infrared is revived.
January 19th - Around 18 Vo-Perium members fight 6 Highland members at spawn, making this the largest battle to unfold since the 6th Incursion
January 26th - Infrared disbands once again
January 30th - The owner changes the server message on the player board, saying "1.13 and temporary map expected in February, 2019, with new server hardware. Elytras planned to be re-added. Death messages and end portals re-added."
January 30th - End portals re-disabled
February 20th - Indonesian YouTuber with 187k subscribers, Kurus, makes a video about 2b2t.
February 23rd - The owner changes server message on the player board, it now says "1.13 expected in late March, Book Duplication now Patched due to update of Waterfall."
February 27th - player bflyza constructs thousands of signs above the nether bedrock ceiling at (0, 0) in the nether. Any player who loads this chunk is immediately disconnected from the server, and instantly disconnects upon rejoining in a way that is functionally similar to being book-banned. Dozens of players are chunk-banned due to the exploit.
7 notes
·
View notes
Text
python a* basic game pathfinding algorithm for navigation in a 2d array
from queue import PriorityQueue import math # A* pathfinding algorithm # https://pythonprogrammingsnippets.tumblr.com/ def astar(start, goal, terrain_map): frontier = PriorityQueue() frontier.put((0, start)) came_from = {} cost_so_far = {} came_from[start] = None cost_so_far[start] = 0 while not frontier.empty(): current = frontier.get()[1] if current == goal: break for next_tile in get_neighbors(current, terrain_map): new_cost = cost_so_far[current] + get_cost(current, next_tile, terrain_map) if next_tile not in cost_so_far or new_cost < cost_so_far[next_tile]: cost_so_far[next_tile] = new_cost priority = new_cost + heuristic(goal, next_tile) frontier.put((priority, next_tile)) came_from[next_tile] = current return came_from, cost_so_far def heuristic(a, b): return math.sqrt((b[0] - a[0]) ** 2 + (b[1] - a[1]) ** 2) def get_neighbors(tile, terrain_map): neighbors = [] for x in range(tile[0] - 1, tile[0] + 2): for y in range(tile[1] - 1, tile[1] + 2): if x >= 0 and y >= 0 and x < len(terrain_map) and y < len(terrain_map[0]): if (x, y) != tile and terrain_map[x][y] != 2: neighbors.append((x, y)) return neighbors def get_cost(current, next_tile, terrain_map): diagonal = abs(current[0] - next_tile[0]) == 1 and abs(current[1] - next_tile[1]) == 1 cost = terrain_map[next_tile[0]][next_tile[1]] if diagonal: cost *= math.sqrt(2) return cost ## ----------------------- def number_to_ascii_character(number): # # 1 = normal, 2 = wall, 3 = water, 4 = lava, 5 = ice, 6 = swamp if number == 1: return " " elif number == 2: return "-" # wall else: return "?" def show_map(terrain_map, path): for x in range(len(terrain_map)): for y in range(len(terrain_map[0])): if (x, y) in path: # if last position in path, show "X" if (x, y) == path[-1]: print("E", end="") # if first position in path, show "S" elif (x, y) == path[0]: print(" B ", end="") else: print(" x ", end="") else: map_char = number_to_ascii_character(terrain_map[x][y]) print(" " + map_char + " ", end="") print("") print("") print("") ## ----------------------- def main(): terrain_map = [ [1, 2, 2, 1, 1, 1, 1, 2, 1, 1, 1, 2, 1, 1, 1, 2, 2, 2 ], [1, 2, 1, 1, 1, 1, 1, 2, 1, 1, 2, 2, 1, 2, 1, 1, 2, 2 ], [1, 2, 2, 2, 1, 1, 1, 2, 1, 1, 2, 2, 1, 2, 1, 2, 1, 2 ], [1, 2, 1, 2, 1, 2, 1, 1, 1, 1, 2, 2, 1, 1, 1, 1, 2, 1 ], [1, 1, 1, 2, 2, 1, 1, 1, 1, 1, 1, 1, 1, 2, 1, 2, 2, 1 ], [1, 1, 1, 2, 2, 1, 1, 2, 1, 1, 1, 1, 1, 2, 1, 2, 2, 1 ], [2, 2, 1, 2, 1, 1, 1, 2, 1, 1, 2, 2, 1, 2, 1, 1, 1, 2 ], [1, 1, 1, 2, 1, 2, 2, 1, 1, 1, 2, 1, 1, 2, 1, 2, 2, 2 ], [1, 1, 1, 2, 2, 1, 1, 2, 1, 1, 1, 2, 1, 2, 1, 2, 2, 2 ], [1, 1, 1, 1, 2, 2, 1, 2, 1, 1, 2, 2, 1, 2, 1, 2, 2, 2 ], [1, 1, 1, 1, 1, 1, 1, 2, 1, 1, 1, 2, 1, 1, 1, 1, 2, 2 ], [1, 1, 1, 1, 1, 1, 1, 2, 1, 1, 2, 1, 1, 1, 1, 1, 1, 2 ], [1, 1, 1, 1, 1, 1, 1, 2, 1, 1, 1, 2, 1, 2, 1, 1, 1, 1 ], [1, 1, 1, 1, 1, 1, 1, 2, 1, 1, 1, 1, 1, 2, 1, 2, 2, 1 ], [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 2, 2, 1, 1, 1, 2, 2, 1 ], [1, 1, 1, 1, 1, 1, 1, 2, 1, 1, 2, 2, 1, 2, 1, 2, 2, 1 ], ] came_from, cost_so_far = astar((0, 0), (9, 9), terrain_map) current = (9, 9) path = [] while current != (0, 0): path.append(current) current = came_from[current] path.reverse() print("Start xy: (0, 0)") print("End xy: (9, 9)") print("-= | Path | =-") print(path) show_map(terrain_map, path) if __name__ == "__main__": main()
output:
Start xy: (0, 0) End xy: (9, 9) -= | Path | =- [(1, 0), (2, 0), (3, 0), (4, 1), (5, 2), (6, 2), (7, 2), (8, 2), (9, 3), (10, 4), (10, 5), (9, 6), (8, 6), (7, 7), (8, 8), (9, 9)] - - - - - - - B - - - - - - - x - - - - - - - - - x - - - - - - x - - - - - x - - - - - - - - x - - - - - - x - - - x - - - - - x - - x - x - - - - - x - - x - E - - - - - - x x - - - - - - - - - - - - - - - - - - - - - - - -
#python#a star#astar#a*#algorithm#pathfinding#path#finding#path finding#game#2d array#navigating#navigation#routing#game ai#game programming#game programmer#games#gaming#gamer#game source code#basic tutorial#tutorial#pythonic#python programming#python source code#source code#source#code#coding
0 notes
Text
The Collection Framework in Java
What is a Collection in Java?
Java collection is a single unit of objects. Before the Collections Framework, it had been hard for programmers to write down algorithms that worked for different collections. Java came with many Collection classes and Interfaces, like Vector, Stack, Hashtable, and Array.
In JDK 1.2, Java developers introduced theCollections Framework, an essential framework to help you achieve your data operations.
Why Do We Need Them?
Reduces programming effort & effort to study and use new APIs
Increases program speed and quality
Allows interoperability among unrelated APIs
Reduces effort to design new APIs
Fosters software reuse
Methods Present in the Collection Interface
NoMethodDescription1Public boolean add(E e)To insert an object in this collection.2Public boolean remove(Object element)To delete an element from the collection.3Default boolean removeIf(Predicate filter)For deleting all the elements of the collection that satisfy the specified predicate.4Public boolean retainAll(Collection c)For deleting all the elements of invoking collection except the specified collection.5Public int size()This return the total number of elements.6Publicvoid clear()This removes the total number of elements.7Publicboolean contains(Object element)It is used to search an element.8PublicIterator iterator()It returns an iterator.9PublicObject[] toArray()It converts collection into array.
Collection Framework Hierarchy
List Interface
This is the child interface of the collectioninterface. It is purely for lists of data, so we can store the ordered lists of the objects. It also allows duplicates to be stored. Many classes implement this list interface, including ArrayList, Vector, Stack, and others.
Array List
It is a class present in java. util package.
It uses a dynamic array for storing the element.
It is an array that has no size limit.
We can add or remove elements easily.
Linked List
The LinkedList class uses a doubly LinkedList to store elements. i.e., the user can add data at the initial position as well as the last position.
It allows Null insertion.
If we’d wish to perform an Insertion /Deletion operation LinkedList is preferred.
Used to implement Stacks and Queues.
Vector
Every method is synchronized.
The vector object is Thread safe.
At a time, one thread can operate on the Vector object.
Performance is low because Threads need to wait.
Stack
It is the child class of Vector.
It is based on LIFO (Last In First Out) i.e., the Element inserted in last will come first.
Queue
A queue interface, as its name suggests, upholds the FIFO (First In First Out) order much like a conventional queue line. All of the elements where the order of the elements matters will be stored in this interface. For instance, the tickets are always offered on a first-come, first-serve basis whenever we attempt to book one. As a result, the ticket is awarded to the requester who enters the queue first. There are many classes, including ArrayDeque, PriorityQueue, and others. Any of these subclasses can be used to create a queue object because they all implement the queue.
Dequeue
The queue data structure has only a very tiny modification in this case. The data structure deque, commonly referred to as a double-ended queue, allows us to add and delete pieces from both ends of the queue. ArrayDeque, which implements this interface. We can create a deque object using this class because it implements the Deque interface.
Set Interface
A set is an unordered collection of objects where it is impossible to hold duplicate values. When we want to keep unique objects and prevent object duplication, we utilize this collection. Numerous classes, including HashSet, TreeSet, LinkedHashSet, etc. implement this set interface. We can instantiate a set object with any of these subclasses because they all implement the set.
LinkedHashSet
The LinkedHashSet class extends the HashSet class.
Insertion order is preserved.
Duplicates aren’t allowed.
LinkedHashSet is non synchronized.
LinkedHashSet is the same as the HashSet except the above two differences are present.
HashSet
HashSet stores the elements by using the mechanism of Hashing.
It contains unique elements only.
This HashSet allows null values.
It doesn’t maintain insertion order. It inserted elements according to their hashcode.
It is the best approach for the search operation.
Sorted Set
The set interface and this interface are extremely similar. The only distinction is that this interface provides additional methods for maintaining the elements' order. The interface for handling data that needs to be sorted, which extends the set interface, is called the sorted set interface. TreeSet is the class that complies with this interface. This class can be used to create a SortedSet object because it implements the SortedSet interface.
TreeSet
Java TreeSet class implements the Set interface it uses a tree structure to store elements.
It contains Unique Elements.
TreeSet class access and retrieval time are quick.
It doesn’t allow null elements.
It maintains Ascending Order.
Map Interface
It is a part of the collection framework but does not implement a collection interface. A map stores the values based on the key and value Pair. Because one key cannot have numerous mappings, this interface does not support duplicate keys. In short, The key must be unique while duplicated values are allowed. The map interface is implemented by using HashMap, LinkedHashMap, and HashTable.
HashMap
Map Interface is implemented by HashMap.
HashMap stores the elements using a mechanism called Hashing.
It contains the values based on the key-value pair.
It has a unique key.
It can store a Null key and Multiple null values.
Insertion order isn’t maintained and it is based on the hash code of the keys.
HashMap is Non-Synchronized.
How to create HashMap.
LinkedHashMap
The basic data structure of LinkedHashMap is a combination of LinkedList and Hashtable.
LinkedHashMap is the same as HashMap except above difference.
HashTable
A Hashtable is an array of lists. Each list is familiar as a bucket.
A hashtable contains values based on key-value pairs.
It contains unique elements only.
The hashtable class doesn’t allow a null key as well as a value otherwise it will throw NullPointerException.
Every method is synchronized. i.e At a time one thread is allowed and the other threads are on a wait.
Performance is poor as compared to HashMap.
This blog illustrates the interfaces and classes of the java collection framework. Which is useful for java developers while writing efficient codes. This blog is intended to help you understand the concept better.
At Sanesquare Technologies, we provide end-to-end solutions for Development Services. If you have any doubts regarding java concepts and other technical topics, feel free to contact us.
0 notes
Text
Queue in java

Hierarchy of interfaces in Java Collection Framework: Relationship between. Next we are adding 5 strings in random order into the priority queue.
See Ĭopyright © 2000–2019, Robert Sedgewick and Kevin Wayne. Queue is a Collection that allows duplicate elements but not null elements. The first line tells us that we are creating a priority queue: Queue testStringsPQ new PriorityQueue () PriorityQueue is available in java.util package.
Chronicle Queue is a persisted low-latency messaging framework for high performance and critical. However, there's one exception that we'll touch upon later. This project covers the Java version of Chronicle Queue. Each of these methods exists in two forms: one throws an exception if the operation fails, the other returns a special value (either null or false, depending on the. Besides basic Collection operations, queues provide additional insertion, extraction, and inspection operations. In fact, most Queues we'll encounter in Java work in this first in, first out manner often abbreviated to FIFO. A collection designed for holding elements prior to processing. * * This implementation uses a singly linked list with a static nested class for * linked-list nodes. After we declare our Queue, we can add new elements to the back, and remove them from the front. Recommended Articles This is a guide to Queue in Java. We have seen examples of the most commonly used methods in queue operation. There are alternative methods available for all the methods. It supports operations like insertion, retrieval, and removal. Hence we create the instance of the LinkedList and the PriorityQueue class and assign it to the queue interface. Since the Queue is an interface, we cannot create an instance of it. ****************************************************************************** * Compilation: javac Queue.java * Execution: java Queue enqueue and dequeue * operations, along with methods for peeking at the first item, * testing if the queue is empty, and iterating through * the items in FIFO order. The Queue is a special interface in Java that is used to hold the elements in insertion order. Queue interface is a part of Java Collections that consists of two implementations: LinkedList and PriorityQueue are the two classes that implement the Queue interface. Below is the syntax highlighted version of Queue.java The Queue interface of the Java collections framework provides the functionality of the queue data structure.

0 notes
Text
Generic Array Priority Queue Solution
Generic Array Priority Queue Solution
For this assignment, you will write two more implementations of a Priority Queue. Using the same interface as program #1, you will write two linked listed implementations. Your implementations will be: 1. Ordered Singly Linked List 2. Unordered Singly Linked List Both implementations must have identical behavior, and must implement the PriorityQueue interface used in program #1. The…
View On WordPress
0 notes
Text
CS Programming Assignment 3 Solution
CS Programming Assignment 3 Solution
For this assignment, you will write one more implementations of a Priority Queue. Using the same interface as program #1, you will write a binary heap implementation. Your heap implementation must have identical behavior, and must implement the PriorityQueue interface used in program #1. The implementation must have two constructors as in the first assignment–use the DEFAULT_MAX_CAPACITY in the…

View On WordPress
0 notes
Text
Коллекции?
Если коротко, то
Существует несколько типов коллекций (интерфейсов):
List - упорядоченный список. Элементы хранятся в порядке добавления, проиндексированы.
Queue - очередь. Первый пришёл - первый ушёл, то есть элементы добавляются в конец, а удаляются из начала.
Set - неупорядоченное множество. Элементы могут храниться в рандомном порядке.
Map - пара ключ-значение. Пары хранятся неупорядоченно.
У них есть реализации.
Реализации List
ArrayList - самый обычный массив. Расширяется автоматически. Может содержать элементы разных классов.
LinkedList - элементы имеют ссылки на рядом стоящие элементы. Быстрое удаление за счёт того, что элементы никуда не сдвигаются, заполняя образовавшийся пробел, как в ArrayList.
Реализации Queue
LinkedList - рассказано выше. Да, оно также реализует интерфейс Queue.
ArrayDeque - двунаправленная очередь, где элементы могут добавляться как в начало так и в конец. Также и с удалением.
PriorityQueue - элементы добавляются в сортированном порядке (по возрастанию или по алфавиту или так, как захочет разработчик)
Реализации Set
HashSet - элементы хранятся в хэш-таблице, в бакетах. Бакет каждого элемента определяется по его хэш-коду. Добавление, удаление и поиск происходят за фиксированное количество времени.
LinkedHashSet - как HashSet, но сохраняет порядок добавления элементов.
TreeSet - используется в том случает, когда элементы надо упорядочить. По умолчанию используется естественный порядок, организованный красно-чёрным деревом. (Нет, я не буду это здесь объяснять, не заставите)
LinkedList - рассказано выше. Да, оно также реализует интерфейс Queue.
ArrayDeque
Реализации Map
HashMap - то же самое что и HashSet. Использует хэш-таблицу для хранения.
TreeMap - тоже использует красно-чёрное дерево. Как и TreeSet, это достаточно медленно работающая структура для малого количества данных.
LinkedHashMap. Честно? Тяжеловато найти информацию про различия с LinkedHashSet, кроме того, что один - это Set, а второй - Map. Мда :/
А связи всего этого выглядят так
Овалы - интерфейсы; Прямоугольники - классы;
Здесь видно, что начало начал - интерфейс Iterable, ну а общий для всех интерфейс - Collection (Не путать с фреймворком Collections)
А где Map? а вот он
Map не наследуется от Collection - он сам по себе.
К этой теме также можно много чего добавить: устройство красно-черного дерева, работа HashSet'ов и HashMap'ов под капотом, сложность коллекций. Но это всё темы для следующих постов.
Ну, а ��ока надеюсь, что понятно.
#студент бормочет#List#Set#Queue#Map#ArrayList#LinkedList#ArrayDeque#PriorityOueue#HashMap#HashSet#LinkedHashMap#LinkedHashSet#TreeMap#TreeSet#как же много тегов#Коллекции
1 note
·
View note
Text
[100% OFF] Master Java Collection Framework
[100% OFF] Master Java Collection Framework
What you Will learn ? Collection Interfaces Types of Data Structures ArrayList Class LinkedList Class Iterator, ListIterator and Spliterator Queue and Stack ArrayDeque Class PriorityQueue Class Map Classes How Hashing Works HashMap Class LinkedHashMap Class TreeMap Class EnumMap, WeakHashMap and IdentityHashMap Classes HashSet Class LinkedHashSet Class TreeSet Class Collection…
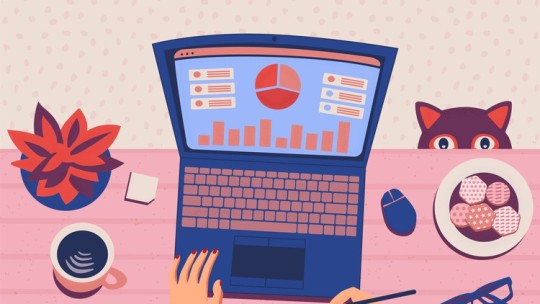
View On WordPress
0 notes
Text
While studying computer science at University of Colorado Boulder, my data structures final project was to investigate different priority queue implementations to store the same data and compare their runtime when enqueuing and dequeuing elements. The data in this project was a list of hospital patients with a name, priority, and operation time. The priority of the patient represents the time until the patient goes into labor. The patients were ordered to have lowest priority first, and then ordered by least operation time if the patients had the same priority. The three implementations were a binary heap, linked list, and the c++ standard template library priority queue.
The binary heap priority queue uses an array to store the data while ordering the data in minimum heap property; every parent node is smaller than its child node. The binary heap insert function uses a swap helper function to keep swapping the inserted value with its greater child until the inserted value satisfies the min heap property. The dequeue function removes the root element because it is always the minimum and replaces it with the last element in the heap to keep the tree complete. The heap is then “heapified” or reordered to ensure the root is still the minimum value and the heap follows the min heap property.
The linked list priority queue uses a patient node structure that contains the address to the next priority element. The insert function traverses the list linearly until the inserted element is correctly ordered by its priority. The delete minimum function is very simple because the minimum is always stored at the head of the list so the function reassigns the head of the list to the second element and deletes the first element.
The standard template priority queue was implemented by creating a comparator struct and vector of the elements being prioritized. We do not necessarily know how the queue works because its source code is not available, but we can compare its runtime to the other implementations of the priority queue.
To test each implantation, the data was read into a array for quick access. A random section of the data with a specific size (100, 200… 800) was then copied into a new array. The different sized and random arrays were then enqueued and dequeued with the three priority queues. To calculate the runtime for enqueuing and dequeuing, the time in milliseconds was recorded before and after the loop with the function call and then stored in a matrix. The mean and standard deviations were then calculated for each queue and respective functions.
The code along with the data, charts and graphs for the mean enqueue and dequeue (writeup.pdf) can be found here on my github: https://github.com/jaq-h/priorityQueue
0 notes
Text

DFS . . . . for more information http://bit.ly/3Zk1l6g check the above link
#spanningtree#avltree#binarytree#Btree#bplustree#binarysearchtree#treedatastructure#linkedlist#array#circularlist#circularlinkedlist#circulardoublylinkedlist#datastructure#computer#algorithm#computerscience#stack#queue#priorityqueue#fifo#lifo#computerengineering#javatpoint
0 notes
Link
Start Learning Java Programming Step By Step with 200+ code examples. 250 Amazing Steps For Absolute Java Beginners!
What you’ll learn
You will Learn Java the MODERN WAY – Step By Step – With 200 HANDS-ON Code Examples
You will Understand the BEST PRACTICES in Writing High Quality Java Code
You will Solve a Wide Range of Hands-on Programming EXERCISES with Java
You will Learn to Write AWESOME Object Oriented Programs with Java
You will Acquire ALL the SKILLS to demonstrate an EXPERTISE with Java Programming in Your Job Interviews
You will learn ADVANCED Object Oriented Programming Concepts – Abstraction, Inheritance, Encapsulation and Polymorphism
You will learn the Basics of Object Oriented Programming – Interfaces, Inheritance, Abstract Class and Constructors
You will learn the Basics of Programming – variables, choosing a data type, conditional execution, loops, writing great methods, breaking down problems into sub problems and implementing great Exception Handling
You will learn Basics of Functional Programming with Java
You will gain Expertise in using Eclipse IDE and JShell
You will learn the basics of MultiThreaded Programming – with Executor Service
You will learn about a wide variety of Java Collections – List, Map, Set and Queue Interfaces
Requirements
You have an attitude to learn while having fun 🙂
You have ZERO Programming Experience and Want to Learn Java
Description
Zero Java Programming Experience? No Problem.
Do you want to take the first steps to Become a Great Java Programmer? Do you want to Learn Java Step By Step in a Fail Safe in28Minutes Way? Do you want to Learn to Write Great Java Programs?
******* Some Amazing Reviews From Our Learners *******
★★★★★ it’s an awesome course , i was a complete beginner and it helped me a lot. One of the best courses i have every taken on Udemy.
★★★★★ This is the best Java course I’ve come across. It’s straight to the point without any missing details. You can get an idea of what you’re getting into working with Java fast with this course. I really like it.
★★★★★ The experienece was extremely amazing. The course was highly detailed and comprehensive and all the topic were covered properly with due examples to their credit. The instructor is passionateabout what he is doing and hence it makes the course much more worth to learn. Kudos to the instructor for such an amazing job.
★★★★★ Never thought taking an online course will be so helpful. The instructor is quite engaging, gives good amount of exercises.
★★★★★ This course is wonderful! I really enjoy it. It really is for beginners, so it’s very helpful for people which don’t know nothing about programming.
★★★★★ Very comprehensive and detail course the instructor takes the patience to explain everything and goes a step forward in thinking what kind of errors could happen to the students really good instructor!
★★★★★ It’s very well thought out. I enjoy the constant exercises and the challenge they present to make things happen.
******* Course Overview *******
Java is one of the most popular programming languages. Java offers both object oriented and functional programming features.
We take an hands-on approach using a combination of JShell and Eclipse as an IDE to illustrate more than 200 Java Coding Exercises, Puzzles and Code Examples. This course assumes no previous ( beginner ) programming or Java experience. If you’ve never programmed a computer before, or if you already have experience with another programming language and want to quickly learn Java, this is a perfect course for you.
In more than 250 Steps, we explore the most important Java Programming Language Features
Basics of Java Programming – Expressions, Variables and Printing Output
Java Operators – Java Assignment Operator, Relational and Logical Operators, Short Circuit Operators
Java Conditionals and If Statement
Methods – Parameters, Arguments and Return Values
Object Oriented Programming – Class, Object, State and Behavior
Basics of OOPS – Encapsulation, Abstraction, Inheritance and Polymorphism
Basics about Java Data Types – Casting, Operators and More
Java Built in Classes – BigDecimal, String, Java Wrapper Classes
Conditionals with Java – If Else Statement, Nested If Else, Java Switch Statement, Java Ternary Operator
Loops – For Loop, While Loop in Java, Do While Loop, Break and Continue
Immutablity of Java Wrapper Classes, String and BigDecimal
Java Dates – Introduction to LocalDate, LocalTime and LocalDateTime
Java Array and ArrayList – Java String Arrays, Arrays of Objects, Primitive Data Types, toString and Exceptions
Introduction to Variable Arguments
Basics of Designing a Class – Class, Object, State and Behavior. Deciding State and Constructors.
Understanding Object Composition and Inheritance
Java Abstract Class and Interfaces. Introduction to Polymorphism.
Java Collections – List Interface(ArrayList, LinkedList and Vector), Set Interface (HashSet, LinkedHashSet and TreeSet), Queue Interface (PriorityQueue) and Map Interface (HashMap, HashTable, LinkedHashMap and TreeMap() – Compare, Contrast and Choose
Generics – Why do we need Generics? Restrictions with extends and Generic Methods, WildCards – Upper Bound and Lower Bound.
Functional Programming – Lambda Expression, Stream and Operations on a Stream (Intermediate Operations – Sort, Distinct, Filter, Map and Terminal Operations – max, min, collect to List), Functional Interfaces – Predicate Interface,Consumer Interface, Function Inteface for Mapping, Method References – static and instance methods
Introduction to Threads and MultiThreading – Need for Threads
Implementing Threads – Extending Thread Class and Implementing Runnable Interface
States of a Thread and Communication between Threads
Introduction to Executor Service – Customizing number of Active Threads. Returning a Future, invokeAll and invokeAny
Introduction to Exception Handling – Your Thought Process during Exception Handling. try, catch and finally. Exception Hierarchy – Checked Exceptions vs Unchecked Exceptions. Throwing an Exception. Creating and Throwing a Custom Exception – CurrenciesDoNotMatchException. Try with Resources – New Feature in Java 7.
List files and folders in Directory with Files list method, File walk method and find methods. Read and write from a File.
******* What You Can Expect from Every in28Minutes Course *******
in28Minutes created 20 Best Selling Courses providing Amazing Learning Experiences to 250,000 Learners across the world.
Each of these courses come with
✔ Amazing Hands-on Step By Step Learning Experiences
✔ Real Project Experiences using the Best Tools and Frameworks
✔ Awesome Troubleshooting Guides with 200+ FAQs Answered
✔ Friendly Support in the Q&A section
✔ Free Udemy Certificate of Completion on Completion of Course
✔ 30 Day “No Questions Asked” Money Back Guarantee!
~~~ Here are a Few Reviews on The in28Minutes Way ~~~
★★★★★ Excellent, fabulous. The way he has prepared the material and the way he teaches is really awesome. What an effort .. Thanks a million
★★★★★ A lot of preparation work has taken place from the teacher and this is visible throughout the course.
★★★★★ This guy is fantastic. Really. Wonderful teaching skills, and goes well out of his way to make sure that everything he is doing is fully understood. This is the kind of tutorial that gets me excited to work with a framework that I may otherwise not be.
★★★★★ The best part of it is the hands-on approach which the author maintained throughout the course as he had promised at the beginning of the lecture. He explains the concepts really well and also makes sure that there is not a single line of code you type without understanding what it really does.
★★★★★ I also appreciate the mind and hands approach of teaching something and then having the student apply it. It makes everything a lot clearer for the student and uncovers issues that we will face in our project early.
★★★★★ Amazing course. Explained super difficult concepts (that I have spent hours on the internet finding a good explanation) in under 5 minutes.
Zero risk. 30 day money-back guarantee with every purchase of the course. You have nothing to lose!
Start Learning Now. Hit the Enroll Button!
******* Step By Step Details *******
Introduction to Java Programming with Jshell using Multiplication Table
Step 00 – Getting Started with Programming Step 01 – Introduction to Multiplication Table challenge Step 02 – Launch JShell Step 03 – Break Down Multiplication Table Challenge Step 04 – Java Expression – An Introduction Step 05 – Java Expression – Exercises Step 06 – Java Expression – Puzzles Step 07 – Printing output to console with Java Step 08 – Printing output to console with Java – Exercise Statements Step 09 – Printing output to console with Java – Exercise Solutions Step 10 – Printing output to console with Java – Puzzles Step 11 – Advanced Printing output to console with Java Step 12 – Advanced Printing output to console with Java – Exercises and Puzzles Step 13 – Introduction to Variables in Java Step 14 – Introduction to Variables in Java – Exercises and Puzzles Step 15 – 4 Important Things to Know about Variables in Java Step 16 – How are variables stored in memory? Step 17 – How to name a variable? Step 18 – Understanding Primitive Variable Types in Java Step 19 – Understanding Primitive Variable Types in Java – Choosing a Type Step 20 – Java Assignment Operator Step 21 – Java Assignment Operator – Puzzles on Increment, Decrement and Compound Assignment Step 23 – Java Conditionals and If Statement – Introduction Step 24 – Java Conditionals and If Statement – Exercise Statements Step 25 – Java Conditionals and If Statement – Exercise Solutions Step 26 – Java Conditionals and If Statement – Puzzles Step 27 – Java For Loop to Print Multiplication Table – Introduction Step 28 – Java For Loop to Print Multiplication Table – Exercise Statements Step 29 – Java For Loop to Print Multiplication Table – Exercise Solutions Step 30 – Java For Loop to Print Multiplication Table – Puzzles Step 31 – Programming Tips : JShell – Shortcuts, Multiple Lines and Variables TODO Move up Step 32 – Getting Started with Programming – Revise all Terminology
Introduction to Method with Multiplication Table
Step 00 – Section 02 – Methods – An Introduction Step 01 – Your First Java Method – Hello World Twice and Exercise Statements Step 02 – Introduction to Java Methods – Exercises and Puzzles Step 03 – Programming Tip – Editing Methods with JShell Step 04 – Introduction to Java Methods – Arguments and Parameters Step 05 – Introduction to Java Method Arguments – Exercises Step 06 – Introduction to Java Method Arguments – Puzzles and Tips Step 07 – Getting back to Multiplication Table – Creating a method Step 08 – Print Multiplication Table with a Parameter and Method Overloading Step 09 – Passing Multiple Parameters to a Java Method Step 10 – Returning from a Java Method – An Introduction Step 11 – Returning from a Java Method – Exercises Step 99 – Methods – Section Review
Introduction to Java Platform
Step 00 – Section 03 – Overview Of Java Platform – Section Overview Step 01 – Overview Of Java Platform – An Introduction – java, javac, bytecode and JVM Step 02 – Java Class and Object – First Look Step 03 – Create a method in a Java class Step 04 – Create and Compile Planet.java class Step 05 – Run Planet calss with Java – Using a main method Step 06 – Play and Learn with Planet Class Step 07 – JDK vs JRE vs JVM
Introduction to Eclipse – First Java Project
Step 01 – Creating a New Java Project with Eclipse Step 02 – Your first Java class with Eclipse Step 03 – Writing Multiplication Table Java Program with Eclipse Step 04 – Adding more methods for Multiplication Table Program Step 05 – Programming Tip 1 : Refactoring with Eclipse Step 06 – Programming Tip 2 : Debugging with Eclipse Step 07 – Programming Tip 3 : Eclipse vs JShell – How to choose?
Introduction To Object Oriented Programming
Step 00 – Introduction to Object Oriented Programming – Section Overview Step 01 – Introduction to Object Oriented Programming – Basics Step 02 – Introduction to Object Oriented Programming – Terminology – Class, Object, State and Behavior Step 03 – Introduction to Object Oriented Programming – Exercise – Online Shopping System and Person Step 04 – Create Motor Bike Java Class and a couple of objects Step 05 – Exercise Solutions – Book class and Three instances Step 06 – Introducing State of an object with speed variable Step 07 – Understanding basics of Encapsulation with Setter methods Step 08 – Exercises and Tips – Getters and Generating Getters and Setters with Eclipse Step 09 – Puzzles on this and initialization of member variables Step 10 – First Advantage of Encapsulation Step 11 – Introduction to Encapsulation – Level 2 Step 12 – Encapsulation Exercises – Better Validation and Book class Step 13 – Introdcution to Abstraction Step 14 – Introduction to Java Constructors Step 15 – Introduction to Java Constructors – Exercises and Puzzles Step 16 – Introduction to Object Oriented Programming – Conclusion
Primitive Data Types And Alternatives
Step 00 – Primitive Data Types in Depth – Section Overview Step 01 – Basics about Java Integer Data Types – Casting, Operators and More Step 02 – Java Integer Data Types – Puzzles – Octal, Hexadecimal, Post and Pre increment Step 03 – Java Integer Data Types – Exercises – BiNumber – add, multiply and double Step 04 – Java Floating Point Data Types – Casting , Conversion and Accuracy Step 05 – Introduction to BigDecimal Java Class Step 06 – BigDecimal Puzzles – Adding Integers Step 07 – BigDecimal Exercises – Simple Interest Calculation Step 08 – Java Boolean Data Type – Relational and Logical Operators Step 09 – Java Boolean Data Type – Puzzles – Short Circuit Operators Step 10 – Java Character Data Type char – Representation and Conversion Step 11 – Java char Data Type – Exercises 1 – isVowel Step 12 – Java char Data Type – Exercises 2 – isDigit Step 13 – Java char Data Type – Exercises 3 – isConsonant, List Upper Case and Lower Case Characters Step 14 – Primitive Data Types in Depth – Conclusion
Conditionals
Step 00 – Conditionals with Java – Section Overview Step 01 – Introduction to If Else Statement Step 02 – Introduction to Nested If Else Step 03 – If Else Statement – Puzzles Step 04 – If Else Problem – How to get User Input in Java? Step 05 – If Else Problem – How to get number 2 and choice from user? Step 06 – If Else Problem – Implementing with Nested If Else Step 07 – Java Switch Statement – An introduction Step 08 – Java Switch Statement – Puzzles – Default, Break and Fall Through Step 09 – Java Switch Statement – Exercises – isWeekDay, nameOfMonth, nameOfDay Step 10 – Java Ternary Operation – An Introduction Step 11 – Conditionals with Java – Conclusion
Loops
Step 00 – Java Loops – Section Introduction Step 01 – Java For Loop – Syntax and Puzzles Step 02 – Java For Loop – Exercises Overview and First Exercise Prime Numbers Step 03 – Java For Loop – Exercise – Sum Upto N Numbers and Sum of Divisors Step 04 – Java For Loop – Exercise – Print a Number Triangle Step 05 – While Loop in Java – An Introduction Step 06 – While Loop – Exericises – Cubes and Squares upto limit Step 07 – Do While Loop in Java – An Introduction Step 08 – Do While Loop in Java – An Example – Cube while user enters positive numbers Step 09 – Introduction to Break and Continue Step 10 – Selecting Loop in Java – For vs While vs Do While
Reference Types
Step 00 – Java Reference Types – Section Introduction Step 01 – Reference Types – How are they stored in Memory? Step 02 – Java Reference Types – Puzzles Step 03 – String class – Introduction and Exercise – Print each word and char on a new line Step 04 – String class – Exercise Solution and Some More Important Methods Step 05 – Understanding String is Immutable and String Concat, Upper Case, Lower Case, Trim methods Step 06 – String Concatenation and Join, Replace Methods Step 07 – Java String Alternatives – StringBuffer and StringBuilder Step 08 – Java Wrapper Classes – An Introduction – Why and What? Step 09 – Java Wrapper Classes – Creation – Constructor and valueOf Step 10 – Java Wrapper Classes – Auto Boxing and a Few Wrapper Constants – SIZE, BYTES, MAX_VALUE and MIN_VALUE Step 11 – Java Dates – Introduction to LocalDate, LocalTime and LocalDateTime Step 12 – Java Dates – Exploring LocalDate – Creation and Methods to play with Date Step 13 – Java Dates – Exploring LocalDate – Comparing Dates and Creating Specific Dates Step 14 – Java Reference Types – Conclusion
Arrays and ArrayLists
Step 00 – Introduction to Array and ArrayList – Section Introduction with a Challenge Step 01 – Understanding the need and Basics about an Array Step 02 – Java Arrays – Creating and Accessing Values – Introduction Step 03 – Java Arrays – Puzzles – Arrays of Objects, Primitive Data Types, toString and Exceptions Step 04 – Java Arrays – Compare, Sort and Fill Step 05 – Java Arrays – Exercise – Create Student Class – Part 1 – Total and Average Marks Step 06 – Java Arrays – Exercise – Create Student Class – Part 2 – Maximum and Minimum Mark Step 07 – Introduction to Variable Arguments – Need Step 08 – Introduction to Variable Arguments – Basics Step 09 – Introduction to Variable Arguments – Enhancing Student Class Step 10 – Java Arrays – Using Person Objects and String Elements with Exercises Step 11 – Java String Arrays – Exercise Solutions – Print Day of Week with Most number of letters and more Step 12 – Adding and Removing Marks – Problem with Arrays Step 13 – First Look at ArrayList – An Introduction Step 14 – First Look at ArrayList – Refactoring Student Class to use ArrayList Step 15 – First Look at ArrayList – Enhancing Student Class with Add and Remove Marks Step 16 – Introduction to Array and ArrayList – Conclusion
Object Oriented Programming Again
Step 00 – Object Oriented Programming – Level 2 – Section Introduction Step 01 – Basics of Designing a Class – Class, Object, State and Behavior Step 02 – OOPS Example – Fan Class – Deciding State and Constructors Step 03 – OOPS Example – Fan Class – Deciding Behavior with Methods Step 04 – OOPS Exercise – Rectangle Class Step 05 – Understanding Object Composition with Customer Address Example Step 06 – Understanding Object Composition – An Exercise – Books and Reviews Step 07 – Understanding Inheritance – Why do we need it? Step 08 – Object is at top of Inheritance Hierarchy Step 09 – Inheritance and Overriding – with toString() method Step 10 – Java Inheritance – Exercise – Student and Employee Classes Step 11 – Java Inheritance – Default Constructors and super() method call Step 12 – Java Inheritance – Puzzles – Multiple Inheritance, Reference Variables and instanceof Step 13 – Java Abstract Class – Introductio Step 14 – Java Abstract Class – First Example – Creating Recipes with Template Method Step 15 – Java Abstract Class – Puzzles Step 16 – Java Interface – Example 1 – Gaming Console – How to think about Intefaces? Step 17 – Java Interface – Example 2 – Complex Algorithm – API defined by external team Step 18 – Java Interface – Puzzles – Unimplemented methods, Abstract Classes, Variables, Default Methods and more Step 19 – Java Interface vs Abstract Class – A Comparison Step 20 – Java Interface Flyable and Abstract Class Animal – An Exercise Step 21 – Polymorphism – An introduction
Collections
Step 01 – Java Collections – Section Overview with Need For Collections Step 02 – List Interface – Introduction – Position is King Step 03 – List Inteface – Immutability and Introduction of Implementations – ArrayList, LinkedList and Vector Step 04 – List Inteface Implementations – ArrayList vs LinkedList Step 05 – List Inteface Implementations – ArrayList vs Vector Step 06 – List Inteface – Methods to add, remove and change elements and lists Step 07 – List and ArrayList – Iterating around elements Step 08 – List and ArrayList – Choosing iteration approach for printing and deleting elements Step 09 – List and ArrayList – Puzzles – Type Safety and Removing Integers Step 10 – List and ArrayList – Sorting – Introduction to Collections sort static method Step 11 – List and ArrayList – Sorting – Implementing Comparable Inteface in Student Class Step 12 – List and ArrayList – Sorting – Providing Flexibility by implementing Comparator interface Step 13 – List and ArrayList – A Summary Step 14 – Set Interface – Introduction – No Duplication Step 15 – Understanding Data Structures – Array, LinkedList and Hashing Step 16 – Understanding Data Structures – Tree – Sorted Order Step 17 – Set Interface – Hands on – HashSet, LinkedHashSet and TreeSet Step 18 – Set Interface – Exercise – Find Unique Characters in a List Step 19 – TreeSet – Methods from NavigableSet – floor,lower,upper, subSet, head and tailSet Step 20 – Queue Interface – Process Elements in Order Step 21 – Introduction to PriorityQueue – Basic Methods and Customized Priority Step 22 – Map Interface – An Introduction – Key and Value Step 23 – Map Interface – Implementations – HashMap, HashTable, LinkedHashMap and TreeMap Step 24 – Map Interface – Basic Operations Step 25 – Map Interface – Comparison – HashMap vs LinkedHashMap vs TreeMap Step 26 – Map Interface – Exercise – Count occurances of characters and words in a piece of text Step 27 – TreeMap – Methods from NavigableMap – floorKey, higherKey, firstEntry, subMap and more Step 28 – Java Collections – Conclusion with Three Tips
Generics
Step 01 – Introduction to Generics – Why do we need Generics? Step 02 – Implementing Generics for the Custom List Step 03 – Extending Custom List with a Generic Return Method Step 04 – Generics Puzzles – Restrictions with extends and Generic Methods Step 05 – Generics and WildCards – Upper Bound and Lower Bound
Introduction to Functional Programming
Step 01 – Introduction to Functional Programming – Functions are First Class Citizens Step 02 – Functional Programming – First Example with Function as Parameter Step 03 – Functional Programming – Exercise – Loop a List of Numbers Step 04 – Functional Programming – Filtering – Exercises to print odd and even numbers from List Step 05 – Functional Programming – Collect – Sum of Numbers in a List Step 06 – Functional Programming vs Structural Programming – A Quick Comparison Step 07 – Functional Programming Terminology – Lambda Expression, Stream and Operations on a Stream Step 08 – Stream Intermediate Operations – Sort, Distinct, Filter and Map Step 09 – Stream Intermediate Operations – Exercises – Squares of First 10, Map String List to LowerCase and Length of String Step 10 – Stream Terminal Operations – 1 – max operation with Comparator Step 11 – Stream Terminal Operations – 2 – min, collect to List, Step 12 – Optional class in Java – An Introduction Step 13 – Behind the Screens with Functional Interfaces – Implement Predicate Interface Step 14 – Behind the Screens with Functional Interfaces – Implement Consumer Interface Step 15 – Behind the Screens with Functional Interfaces – Implement Function Inteface for Mapping Step 16 – Simplify Functional Programming code with Method References – static and instance methods Step 17 – Functions are First Class Citizens Step 18 – Introduction to Functional Programming – Conclusion
Introduction to Threads And Concurrency
Step 01 – Introduction to Threads and MultiThreading – Need for Threads Step 02 – Creating a Thread for Task1 – Extending Thread Class Step 03 – Creating a Thread for Task2 – Implement Runnable Interface Step 04 – Theory – States of a Thread Step 05 – Placing Priority Requests for Threads Step 06 – Communication between Threads – join method Step 07 – Thread utility methods and synchronized keyword – sleep, yield Step 08 – Need for Controlling the Execution of Threads Step 09 – Introduction to Executor Service Step 10 – Executor Service – Customizing number of Threads Step 11 – Executor Service – Returning a Future from Thread using Callable Step 12 – Executor Service – Waiting for completion of multiple tasks using invokeAll Step 13 – Executor Service – Wait for only the fastest task using invokeAny Step 14 – Threads and MultiThreading – Conclusion
Introduction to Exception Handling
Step 01 – Introduction to Exception Handling – Your Thought Process during Exception Handling Step 02 – Basics of Exceptions – NullPointerException and StackTrace Step 03 – Basics of Handling Exceptions – try and catch Step 04 – Basics of Handling Exceptions – Exception Hierarchy, Matching and Catching Multiple Exceptions Step 05 – Basics of Handling Exceptions – Need for finally Step 06 – Basics of Handling Exceptions – Puzzles Step 07 – Checked Exceptions vs Unchecked Exceptions – An Example Step 08 – Hierarchy of Errors and Exceptions – Checked and Runtime Step 09 – Throwing an Exception – Currencies Do Not Match Runtime Exception Step 10 – Throwing a Checked Exception – Throws in method signature and handling Step 11 – Throwing a Custom Exception – CurrenciesDoNotMatchException Step 12 – Write less code with Try with Resources – New Feature in Java 7 Step 13 – Basics of Handling Exceptions – Puzzles 2 Step 14 – Exception Handling – Conclusion with Best Practices
Files and Directories
Step 01 – List files and folders in Directory with Files list method Step 02 – Recursively List and Filter all files and folders in Directory with Step Files walk method and Search with find method Step 03 – Read content from a File – Files readAllLines and lines methods Step 04 – Writing Content to a File – Files write method Step 05 – Files – Conclusion
More Concurrency with Concurrent Collections and Atomic Operations
Step 01 – Getting started with Synchronized Step 02 – Problem with Synchronized – Less Concurrency Step 03 – Enter Locks with ReEntrantLock Step 04 – Introduction to Atomic Classes – AtomicInteger Step 05 – Need for ConcurrentMap Step 06 – Implementing an example with ConcurrentHashMap Step 07 – ConcurrentHashMap uses different locks for diferrent regions Step 08 – CopyOnWrite Concurrent Collections – When reads are more than writes Step 09 – Conclusion
Java Tips
Java Tip 01 – Imports and Static Imports Java Tip 02 – Blocks Java Tip 03 – equals method Java Tip 04 – hashcode method Java Tip 05 – Class Access Modifiers – public and default Java Tip 06 – Method Access Modifiers – public, protected, private and default Java Tip 07 – Final classes and Final methods Java Tip 08 – Final Variables and Final Arguments Java Tip 09 – Why do we need static variables? Java Tip 09 – Why do we need static methods? Java Tip 10 – Static methods cannot use instance methods or variables Java Tip 11 – public static final – Constants Java Tip 12 – Nested Classes – Inner Class vs Static Nested Class Java Tip 13 – Anonymous Classes Java Tip 14 – Why Enum and Enum Basics – ordinal and values Java Tip 15 – Enum – Constructor, variables and methods Java Tip 16 – Quick look at inbuild Enums – Month, DayOfWeek
Zero risk. 30 day money-back guarantee with every purchase of the course. You have nothing to lose!
Start Learning Now. Hit the Enroll Button!
Who this course is for:
You have ZERO programming experience and want to learn Java Programming
You are a Beginner at Java Programming and want to Learn to write Great Java Programs
You want to learn the Basics of Object Oriented Programming with Java
You want to learn the Basics of Functional Programming with Java
Created by in28Minutes Official Last updated 1/2019 English English [Auto-generated]
Size: 2.80 GB
Download Now
https://ift.tt/2A4bRbM.
The post Java Programming for Complete Beginners – Learn in 250 Steps appeared first on Free Course Lab.
0 notes