#Builder Design Pattern in java
Explore tagged Tumblr posts
Text
java- single constructor Vs multiple constructors
❌ The Multiple Constructor Example
public class Human {
private String name;
private int limbs;
private String skinColor;
public Human(String name) {
this(name, 4, "Unknown"); // Magic numbers!
}
public Human(String name, int limbs) {
this(name, limbs, "Unknown");
}
Why this fails: Hidden assumptions (Why default limbs = 4?), duplicate validation (What if limbs < 0?), brittle maintenance (Adding bloodType breaks all constructors)
✅ The Single Constructor Solution
public class Human {
private final String name; // Required
private final int limbs; // Required
private final String skinColor; // Required
public Human(String name, int limbs, String skinColor) {
Objects.requireNonNull(name);
if (limbs < 0) throw new IllegalArgumentException("Limbs cannot be negative");
this.name = name;
this.limbs = limbs;
this.skinColor = skinColor;
}
}
benefits: No magic defaults -Forces explicit values, validation in one place - Fail fast principle, immutable by design - Thread-safe and predictable
Handling Optional Fields: The Builder Pattern For complex cases (like optional eyeColor), use a Builder:
Human britta = new Human.Builder("Britta", 4)
.skinColor("dark")
.eyeColor("blue")
.build();
Why Builders win: Clear defaults (`.skinColor("dark")` vs. constructor overloading), flexible (Add new fields without breaking changes), readable (Named parameters > positional args)
When Multiple Constructors Make Sense
Simple value objects (e.g., Point(x, y)), framework requirements (JPA/Hibernate no-arg constructor), most classes need just one constructor. Pair it with: factory methods for alternative creation logic and builders for optional parameters
This approach eliminates: hidden defaults, validation duplication and maintenance nightmares Do you prefer single or multiple constructors? Have you been bitten by constructor overload? Share your war stories in the comments!
#Java #CleanCode #OOP #SoftwareDevelopment #Programming
1 note
·
View note
Text
Discuss common design patterns and their implementation in Java.
Introduction
Overview of design patterns in software development.
Why design patterns are crucial for writing scalable, maintainable, and reusable code.
Categories of design patterns:
Creational — Object creation mechanisms.
Structural — Class and object composition.
Behavioral — Communication between objects.
1. Creational Design Patterns
1.1 Singleton Pattern
Ensures that only one instance of a class is created.
Used for logging, database connections, and configuration management.
Implementation:javapublic class Singleton { private static Singleton instance; private Singleton() {} // Private constructor public static synchronized Singleton getInstance() { if (instance == null) { instance = new Singleton(); } return instance; } }
✅ Use Case: Database connection manager.
1.2 Factory Pattern
Provides an interface for creating objects without specifying their exact class.
Implementation:javainterface Shape { void draw(); }class Circle implements Shape { public void draw() { System.out.println("Drawing Circle"); } }class Square implements Shape { public void draw() { System.out.println("Drawing Square"); } }class ShapeFactory { public static Shape getShape(String type) { if (type.equalsIgnoreCase("CIRCLE")) return new Circle(); if (type.equalsIgnoreCase("SQUARE")) return new Square(); return null; } }// Usage Shape shape = ShapeFactory.getShape("CIRCLE"); shape.draw();
✅ Use Case: UI component creation (e.g., buttons, text fields).
1.3 Builder Pattern
Used for constructing complex objects step by step.
Implementation:javaclass Car { private String engine; private int wheels; public static class Builder { private String engine; private int wheels; public Builder setEngine(String engine) { this.engine = engine; return this; } public Builder setWheels(int wheels) { this.wheels = wheels; return this; } public Car build() { return new Car(this); } } private Car(Builder builder) { this.engine = builder.engine; this.wheels = builder.wheels; } }// Usage Car car = new Car.Builder().setEngine("V8").setWheels(4).build();
✅ Use Case: Configuring objects with multiple optional parameters (e.g., HTTP requests).
2. Structural Design Patterns
2.1 Adapter Pattern
Allows incompatible interfaces to work together.
Implementation:javainterface MediaPlayer { void play(String audioType); }class MP3Player implements MediaPlayer { public void play(String audioType) { System.out.println("Playing MP3 file"); } }class MP4Player { void playMP4() { System.out.println("Playing MP4 file"); } }class MediaAdapter implements MediaPlayer { private MP4Player mp4Player; public MediaAdapter() { mp4Player = new MP4Player(); } public void play(String audioType) { if (audioType.equalsIgnoreCase("MP4")) { mp4Player.playMP4(); } } }// Usage MediaPlayer player = new MediaAdapter(); player.play("MP4");
✅ Use Case: Integrating third-party libraries.
2.2 Decorator Pattern
Dynamically adds behavior to objects.
Implementation:javainterface Coffee { String getDescription(); double cost(); }class SimpleCoffee implements Coffee { public String getDescription() { return "Simple Coffee"; } public double cost() { return 5.0; } }class MilkDecorator implements Coffee { private Coffee coffee; public MilkDecorator(Coffee coffee) { this.coffee = coffee; } public String getDescription() { return coffee.getDescription() + ", Milk"; } public double cost() { return coffee.cost() + 1.5; } }// Usage Coffee coffee = new MilkDecorator(new SimpleCoffee()); System.out.println(coffee.getDescription() + " - $" + coffee.cost());
✅ Use Case: Adding toppings to a coffee order in an online coffee shop.
3. Behavioral Design Patterns
3.1 Observer Pattern
Defines a one-to-many dependency between objects.
Implementation:javaimport java.util.ArrayList; import java.util.List;interface Observer { void update(String message); }class Subscriber implements Observer { private String name; public Subscriber(String name) { this.name = name; } public void update(String message) { System.out.println(name + " received: " + message); } }class Publisher { private List<Observer> observers = new ArrayList<>(); public void addObserver(Observer observer) { observers.add(observer); } public void notifyObservers(String message) { for (Observer observer : observers) { observer.update(message); } } }// Usage Publisher newsPublisher = new Publisher(); Observer user1 = new Subscriber("Alice"); Observer user2 = new Subscriber("Bob");newsPublisher.addObserver(user1); newsPublisher.addObserver(user2);newsPublisher.notifyObservers("New article published!");
✅ Use Case: Event-driven notifications (e.g., stock market updates, messaging apps).
3.2 Strategy Pattern
Defines a family of algorithms, encapsulates them, and makes them interchangeable.
Implementation:javainterface PaymentStrategy { void pay(int amount); }class CreditCardPayment implements PaymentStrategy { public void pay(int amount) { System.out.println("Paid $" + amount + " using Credit Card."); } }class PayPalPayment implements PaymentStrategy { public void pay(int amount) { System.out.println("Paid $" + amount + " using PayPal."); } }class PaymentContext { private PaymentStrategy strategy; public void setPaymentStrategy(PaymentStrategy strategy) { this.strategy = strategy; } public void pay(int amount) { strategy.pay(amount); } }// Usage PaymentContext context = new PaymentContext(); context.setPaymentStrategy(new PayPalPayment()); context.pay(100);
✅ Use Case: Payment gateway selection (Credit Card, PayPal, etc.).
Conclusion
Design patterns enhance code reusability, scalability, and maintainability.
Each pattern solves a specific design problem efficiently.
Choosing the right pattern is key to writing better Java applications.
🔹 Next Steps:
Explore Java Design Pattern Libraries like Spring Framework.
Implement patterns in real-world projects.
Experiment with combining multiple patterns.
0 notes
Text
Understanding Workflow Automation: A Technical Deep Dive
Today, it has become common to see more and more firms engage workflow automation to manage business operations more efficiently and minimize mistakes in their operations. Workflow automation refers to the execution of tasks, forwarding of information, or carrying out processes without human interaction with the use of technology. In addition to speeding up task completion, the automation approach assists in the delivery of consistent and accurate outcomes in a multitude of business functions.
Defining Workflow Automation:
Workflow automation is the software mechanism of using the automatic flow of tasks, documents, and information across work-related activities based on defined business rules. It aims to perform these tasks independently, improving everyday productivity.
Key Components of Workflow Automation Systems:
Process Mapping and Modeling: Before being automated, such workflows need to be mapped as a way to identify areas to improve. Each step, each decision point, and the way information flows would be depicted by detailed diagrams created for this very purpose. A standardized method that tools like BPMN provide includes modeling these processes.
Automation Software: As the heart of workflow automation software, this represents the actual running of predefined actions. The latter can be rather simple task scheduling tools or are complex systems to integrate with an array of different applications and database systems. Two examples include the open-source, Java-based jBPM which is a workflow engine that reads business processes represented in BPMN 2.0.
Integration capabilities: This refers to how smoothly the data moves across the applications, especially to automate data retrieval, update, and synchronize between platforms. These integrations usually come preinstalled in many of the latest workflow automation tools or can be used through connectors and APIs.
User Interface and Experience: The use of a non-technical, user-friendly interface means that end users can easily design, monitor, and manage their workflows. Users will intuitively be able to interact with the automation system by drag-and-drop builders, visual flowcharts, and dashboards without reliance on IT.
Technical Mechanisms Behind Workflow Automation:
Workflow automation functions using a mix of pre-configured rules, triggers, and actions:
Triggers: It means an event or set of circumstances that sets the workflow running. An example might be getting a new email, form submission, or reaching a certain hour in the day.
Conditions: Logical statements that determine the path of the workflow. For instance, if a purchase order is more than a specific amount, then it may need managerial approval.
Actions: The activities carried out in the process. This could include sending notifications, updating databases, or generating reports.
All these components ensure that processes are carried out with precision and efficiency.
Workflow Automation Advanced Concepts
RPA is the use of software to replicate human-like activities within automated systems. While RPA would directly interact at the user level because it works outside of any APIs, using the traditional type of automation depends on APIs.
Artificial Intelligence Integration: Integration of AI in the workflow automation would enable systems to handle unstructured data, take decisions, and learn from patterns. For example, AI may be used in routing incoming emails based on the content analysis for routing them into appropriate departments.
Event-Driven Architecture: This is a design paradigm that handles the production, detection, and reaction to events. The workflow automation event-driven approach ensures systems respond to change in real time, such as changes in the stock level or customer inquiry. This increases agility and responsiveness.
Challenges and Considerations:
While workflow automation offers numerous benefits, it's essential to approach implementation thoughtfully:
Process Optimization: Automating an inefficient process can amplify existing issues. It is very important to optimize workflows before automating them for maximum benefit.
Scalability: With an increase in organizations, the workflow can get complicated. Choosing the right automation tool that scales up with the needs of the organization is necessary to avoid future bottlenecks.
Security: Automated workflows often handle sensitive data. Information will be secure through the means of strong access controls and encryption for the confidentiality and integrity.
Future Trends in Workflow Automation
Workflow automation is changing rapidly:
Hyperautomation is the amalgamation of multiple technologies including RPA, AI, ML, aiming for total automation of the complex business process.
Low Code/No-Code Platforms. Such platforms are empowered to create workflows by end-users with minimum programming skills and therefore democratize automation across an organization.
Intelligent Process Automation: IPA combines the capabilities of AI with RPA to execute even more complex business processes that include decision-making capacities, like fraud detection or handling customer service conversations.
Conclusion:
Workflow automation is basically in the top of technological progress, giving the organization the tool to better its efficiency, accuracy, and adaptability. Based on its technical foundations, businesses will find a way to keep up with the stream of the advance that workflow automation dictates to compete with a fast-changing digital environment.
0 notes
Text
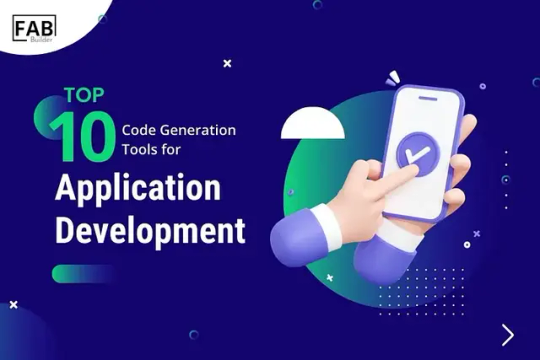
Top 10 Code Generation Tools for Application Development
Code generation tools are now necessary in the quickly changing software development industry to increase output, preserve code quality, and follow best practices. These tools aid developers by producing boilerplate code, automating tedious coding chores, and guaranteeing consistency throughout big codebases. The top 10 code generating tools that may greatly improve your application development process are covered in this article. Fab Builder is a standout tool among these due to its special features and skills.
1. Fab Builder
Overview
Fab Builder is a powerful code generation tool designed to streamline the development process. It allows developers to define code templates and generate code based on predefined patterns. Fab Builder supports various programming languages and frameworks, making it a versatile tool for any development team.
Key Features:
Support for multiple languages and frameworks
Easy integration with existing projects
Cloud And Local File Storage
Flexible Security
Use Cases:
Enterprise Applications: Fab Builder can be used to quickly generate standard components across various enterprise applications, ensuring consistency and adherence to company coding standards.
Microservices: It can greatly minimise the initial setup time by automating the generation of standard code for microservices.
REST APIs: All required code, such as controllers, routes, and models, may be generated by developers along with specifying API endpoints.
2. JHipster
Overview
JHipster is a platform for building and distributing Spring Boot + Angular/React/Vue web apps as well as Spring microservices. The greatest features from the Java and JavaScript ecosystems are combined.
Key Features:
Full-stack code generation
Microservices architecture support
Integration with popular tools like Docker and Kubernetes
Use Cases:
Full-Stack Applications: Generate complete Spring Boot and Angular/React/Vue applications with a single command, allowing developers to focus on business logic.
Microservices: JHipster’s support for microservices architecture makes it easy to create and manage multiple interconnected services.
DevOps Integration: Seamless integration with Docker and Kubernetes helps in deploying applications to production with ease.
3. Yeoman
Overview
Yeoman is a feature-rich, free and open-source scaffolding tool for contemporary websites. It offers boilerplate code for several frameworks and libraries as part of its generator ecosystem, which might assist you in getting new projects off the ground.
Key Features:
Extensive library of generators
Easy to extend and customize
Integration with popular frameworks like Angular, React, and Vue.js
Use Cases:
Web Applications: Yeoman can quickly set up new web applications with all the necessary boilerplate code, saving developers hours of manual setup.
Project Prototyping: Ideal for rapid prototyping, Yeoman allows teams to generate the basic structure of a project and start development immediately.
Educational Purposes: Beginners can use Yeoman to learn about different frameworks by generating projects and exploring the generated code.
4. CodeSmith Tools
Overview
With CodeSmith Generator, you can automatically generate code for any.NET application using a template-based code generator.
Key Features:
.NET-specific code generation
Highly customizable templates
Integration with Visual Studio
Use Cases:
Entity Framework: Generate Entity Framework models and configurations from database schemas.
Custom Templates: Develop custom templates for specific business needs, ensuring all generated code meets your requirements.
Code Consistency: Maintain consistent code structures and practices across all .NET projects.
5. Swagger Codegen
Overview
Using an OpenAPI Specification, developers may use Swagger Codegen to automatically produce client libraries, server stubs, API documentation, and settings. This tool helps in creating APIs quickly and ensures consistency.
Key Features:
Support for multiple programming languages
Generation of client SDKs and server stubs
Easy integration with CI/CD pipelines
Use Cases:
API Development: Swagger Codegen can be used to generate server stubs for new APIs, providing a solid starting point for further development.
Client SDKs: To facilitate other developers’ integration with your service, automatically produce client SDKs for your APIs in a variety of programming languages.
Documentation: Generate documentation straight from the OpenAPI Specification to ensure it is current.
6. Rails Scaffold
Overview
With the Ruby on Rails framework, files and folders may be created automatically with the help of Rails Generators. By adhering to Rails principles, these generators may provide views, controllers, models, migrations, and more.
Key Features:
Built-in Rails support
Customizable templates
Helps maintain DRY principles
Use Cases:
CRUD Applications: Quickly generate all necessary components for a new CRUD (Create, Read, Update, Delete) resource.
Database Migrations: Automatically create and manage database migrations, ensuring schema consistency.
Rapid Prototyping: Ideal for quickly setting up new Rails applications and prototyping new features.
7. Hibernate Tools
Overview
Hibernate Tools is a suite of tools for Hibernate and JPA development. It includes features for code generation, reverse engineering, and database schema synchronization.
Key Features:
Extensive collection of templates
Easy to create and share templates
Supports both Python and other languages
Use Cases:
Python Packages: Generate the boilerplate code for new Python packages, including setup scripts, documentation, and test configurations.
Data Science Projects: Create consistent project structures for data science projects, making it easier to manage and share your work.
Custom Templates: Develop and distribute custom templates for your team, ensuring all new projects adhere to company standards.
8. Angular CLI
Overview
The official command-line interface for Angular is called Angular CLI. It streamlines the Angular application development, scaffolding, initialization, and maintenance processes.
Key Features:
Angular-specific code generation
Built-in support for unit testing and end-to-end testing
Integration with Webpack
Use Cases:
Component Generation: Quickly generate new Angular components, services, and modules, ensuring they follow Angular’s best practices.
Project Setup: Set up new Angular projects with all necessary configurations, saving time on initial setup.
Testing: Generate test configurations and run unit tests with built-in commands, promoting test-driven development.
9. Plop
Overview
Plop is a micro-generator framework that helps you create files, templates, and boilerplate code. It’s highly configurable and can be integrated into any project.
Key Features:
Simple and lightweight
Highly customizable
Works with any project setup
Use Cases:
Component Generation: Automatically generate React components, Redux actions, and reducers, following your project’s conventions.
Code Consistency: Ensure consistent structure and coding standards across your project by using predefined templates.
Template Management: Manage and reuse templates across different projects, reducing redundancy.
10. Microsoft Power Automate
Overview
Microsoft Power Automate, formerly known as Microsoft Flow, is a service that helps automate workflows and tasks across applications and services. It includes capabilities for code generation and workflow automation.
Key Features:
Rapid application development
Integration with the Spring ecosystem
Customizable code generation
Use Cases:
Spring Applications: Quickly generate the structure and necessary components for new Spring applications.
Code Consistency: Ensure consistent coding standards and architecture across all Spring projects.
Prototyping: Rapidly prototype new features and applications using predefined templates and commands.
Conclusion
Choosing the right code generation tool can greatly enhance your development efficiency and code quality. Fab Builder stands out among these tools with its versatility, multi-language support, and powerful features. By incorporating Fab Builder and other tools mentioned in this blog into your development workflow, you can streamline your processes, reduce manual coding efforts, and focus on creating innovative solutions.
Each tool has its strengths and is suited for different scenarios, so consider your project requirements and workflow preferences when making a decision. Happy coding!
#low code platform#code generation#code generation tools#low code app development#low code application development#low code#application developer#app developer#application development
0 notes
Text
Coding with Confidence: The Java Course in Pune at Cyber Success!
Object- Oriented Programming in Java
Introduction:
Java Programming is a high-stage, object-oriented programming language developed by Sun Microsystems (now owned by Oracle) in 1990. It was designed with the intention of being platform-independent, permitting builders to write down code as soon as possible and run it on any tool that helps Java, thanks to its "write once, run anywhere" (WORA) philosophy.
In the world of software development, Java programming stands as a pillar of strength. At Cyber Success, an IT training institute in Pune, we understand the important role Java plays in shaping modern applications. Our full-stack Java developer course is designed for aspiring developers with the knowledge and skills needed to excel in the dynamic software world.
Object-Oriented Programming (OOP) is a powerful paradigm that permits developers to create modular, reusable, and scalable software program structures. At its core, OOP revolves around the concept of "objects," which can be instances of instructions that encapsulate data (attributes) and conduct (strategies). Java, being an object-oriented language, completely embraces those principles, making it a famous preference for growing a wide variety of applications, from simple desktop tools to complex company structures.
* Applying OOP Principles in Java Programming:
Java Programming's rich support for OOP makes it an ideal choice for implementing these principles.
* Classes:
In Java, elegance is described by using the usage of the magnificence key-word followed via the elegance call. It consists of information contributors (fields or attributes) and member abilities (techniques) that outline the behavior of items of that beauty. Classes act as templates from which gadgets are created. They encapsulate statistics and behavior right into a single unit, promoting code reusability and maintainability.
* Objects:
Objects are times of commands. They constitute real global entities and might have interaction with every other thru strategies defined via their respective instructions. Each object has its very personal nation (attributes) and behavior (techniques). Objects are created by using the use of the new key phrase observed through the magnificence call, and they could get admission to the participants in their beauty using the dot (.) operator.
* Key Points:
Classes are blueprints or templates for creating devices.
Objects are instances of commands and represent actual-international entities.
Classes encapsulate records (attributes) and behavior (strategies) proper into an unmarried unit.
Objects have states (attributes) and behaviors (strategies) described with the useful resource of their class.
Objects can engage with every other via strategies.
Key Principles of OOP
Encapsulation
Encapsulation is the practice of bundling the facts (attributes) and techniques (features) that operate on the data into an unmarried unit, referred to as a class.
Inheritance
Inheritance is a mechanism where a brand new class (subclass) can inherit attributes and techniques from a current elegance (superclass).
Polymorphism
Polymorphism is the potential of objects to take on different forms based totally on the context in which they're used.
Abstraction
Abstraction is the act of hiding the implementation information of a category and only exposing the important capabilities.
Object-Oriented Programming (OOP) offers many advantages to Java programming, which is a well-known proposition of software application development.
Here are a few major OOP blessings in Java:
Modularity:
OOP allows creators to break down a complex software program into small executable (trainable) modules. Each Grandeur carries its own individual statistics (characteristics) and conduct (patterns), making the code easier to capture, store, and execute.
Reusability:
OOP encourages code reusability through the concept of inheritance. In Java, commands can inherit attributes and methods from exception directives, allowing constructors to reuse current code and avoid unnecessary functionality.
Scalability:
Scalability is allowed in such a way that OOP developers can define new functionality and capabilities in a software device without impacting the current code. Rewrites can be made to improve device performance without increasing the current codebase.
Flexibility:
OOP provides flexibility in software design by enabling developers to translate real international functions into objects. This allows software programs to be developed that better reflect real-world scenarios, making it much easier
Learn Java programming, from its basics to advanced concepts. At the Cyber Success IT Institute, we have an experienced faculty of trainers. Whether you are an experienced software pro, new to coding, or stepping into IT, our Java course in Pune, will be the best to consider for career opportunities in the future. Attend two demo sessions for free, join the best Java course in Pune, and become a master of the coding world.

In our Full-Stack Java Development Course you will learn,
J2SE (Core Java)
J2EE (Advance Java)
Hibernate
Spring Boot with Microservices
Development Tools
Database (SQL/PL)
Why to choose Cyber Success for Full-stack Java Developer Course,
Comprehensive curriculum:
Cyber Success’s Java course in Pune covers the basics, data structures, object-oriented programming, and advanced topics. From high-speed internet to advanced labs, we have everything you need to get interested in technology. You will learn the basics of core Java and its databases (SQL and PL).
Experienced Instructors:
At Cyber Success Our key point in our Java classes in Pune is our trainers. Our trainers are experts in their field with over five years of experience. They have an in-depth knowledge of the Java programming language, enabling them to skillfully explain problematic concepts and provide a practical approach. Our instructors provide a rich blend of knowledge and real-world experience in the classroom to ensure that scholars are not merely educated but given new tips, demanding the tech industry, and guided to deal with situations. At our institute, you will get top-notch Java education in Pune, including possible full exposure to Java.
Hands-on projects:
At Cyber Success, we believe in studying through practical application. Our Java training in Pune is designed to offer hands-on learning through initiatives, coding
physical activities, and realistic assignments. This approach allows college students to use their theoretical understanding in real-world scenarios, honing their competencies and getting them ready for the demanding situations of the tech enterprise.
Job Placement Assistance:
We are pleased to announce that, in order for our students to find rewarding positions in the tech industry, we offer100% job placement support. For helping us use the skills they gained in their Java training in Pune to help them find meaningful assignments.
In addition, we conduct weekly mock interview sessions to prepare students for real job interviews.
Campus drive:
At Cyber Success, we are dedicated to grooming proficient software engineers for the future. Our intention is to turn IT aspirations into reality for people obsessed with coming into this dynamic field. From the start, we have stayed true to our ideas of hard work, honesty, and ardor, eschewing deceptive practices. This commitment, coupled with our team of seasoned trainers, has installed us as the ultimate IT education center in India.
Mastering Object-Oriented Programming in Java is not just about getting to know a programming language; it is approximately embracing a paradigm that shapes the way we think and build software. At Cyber Success, we're dedicated to empowering our students with the understanding and skills they need to emerge as successful full-stack Java developers.
Success starts with a dream! Let Cyber Success turn your dreams into a successful career. Join us today!
Attend 2 Demo Sessions for free!
Register now to secure your future: https://www.cybersuccess.biz/contact-us/.
📍 Our Visit : Cyber Success, Asmani Plaza, 1248 A, opp. Cafe Goodluck Road, Pulachi Wadi, Deccan Gymnasium, Pune, Maharashtra 411004
📞 For more information, call: +91 9226913502, 9168665644.
“ YOUR WAY TO SUCCESS - CYBER SUCCESS 👍”
0 notes
Text
The 6 High Paying Roles That Will Turn Your Data Skills into Gold!🌟
Hey there, data enthusiasts!
Data science is taking the world by storm, and with it, a plethora of exciting and lucrative career opportunities are opening up. If you're someone who loves numbers, technology, and unraveling mysteries, then data science might just be your calling.
Data Architect:
Imagine being the mastermind behind an organization's data management framework, ensuring that data flows seamlessly across the company. That's what data architects do. They're like data whisperers, understanding the complexities of data and designing systems that make it accessible and useful. To be a data architect, you'll need to be fluent in programming languages like Python, Java, and C++, and have a solid grasp of data modeling tools and predictive modeling.
Data Scientist:
Data scientists are the detectives of the data world, using their scientific skills and algorithms to uncover hidden insights from vast amounts of information. They're like data archaeologists, digging through layers of data to find patterns, trends, and predictions that can change the game. To be a data scientist, you'll need to be proficient in Python, SQL, statistical analysis, and data visualization.
Data Modeler:
Data modelers are the bridge builders between the business world and the data realm. They translate business requirements into data models that computers can understand and use effectively. It's like creating a blueprint for data, ensuring that it's organized, consistent, and ready for action. To be a data modeler, you'll need to master SQL, metadata management, and data modeling tools.
Big Data Engineer:
In the age of big data, big data engineers are the wranglers who manage and analyze massive volumes of information. They're like data cowboys, taming the wild west of data and making it useful. They build data pipelines, design and manage data infrastructures, and handle the ETL (Extract, Transform, Load) process. To be a big data engineer, you'll need to be proficient in Python, SQL, and Java, and have expertise in automation and scripting.
AI Engineer:
Artificial Intelligence (AI) engineers are the masterminds behind intelligent systems and machines that mimic human intelligence to solve complex problems. They're like robot creators, designing and building systems that can learn, adapt, and make decisions on their own. To be an AI engineer, you'll need to be proficient in programming languages, statistics, deep learning, and problem-solving.
Machine Learning Engineer:
Machine Learning (ML) engineers are the automation masters, designing and implementing self-running software that automates predictive models and enables machines to work independently. They're like data magicians, turning data into intelligent tools that can make predictions, optimize processes, and revolutionize industries. To be an ML engineer, you'll need to be proficient in programming languages like Python, Java, and SQL, as well as artificial intelligence and statistics.
The Data Science Adventure Awaits
The field of data science is a thrilling adventure filled with exciting challenges and rewarding opportunities. With the ever-increasing demand for skilled data professionals, these roles are not only in high demand but also command impressive salaries. So, if you're ready to embark on a rewarding career path, consider exploring the world of data science and unleash your inner data ninja!
Remember, data science is not just about numbers and algorithms; it's about creativity, problem-solving, and the ability to make a real impact on the world. So, grab your data toolbox and get ready to dive into the exciting world of data science!
1 note
·
View note
Text
Builder Design Pattern Tutorial Explained with Examples for Beginners and Students
Full video link https://youtu.be/8UelSNeFlTc Hello friends, a new #video about #BuilderPattern #Java #Design #Pattern is published on #codeonedigest #youtube channel. This video covers topics 1. What is #BuilderDesignPattern? 2. What is the use of Buil
Builder design pattern is a type of Creational Java design pattern. Builder design pattrn with examples for students, beginners and software engineers. Complete tutorial of Builder design pattern explained with the code examples. Java design pattern is the backbone of software architecture design & development. Gang of Four design patterns are articulated for Object oriented programming…
View On WordPress
#builder design pattern example#Builder Design Pattern in java#builder design pattern in java example#builder design pattern in spring boot#builder design pattern real world example#builder pattern challenge#builder pattern example#builder pattern in design pattern#builder pattern in design pattern java#builder pattern in java example#builder pattern in spring boot#builder pattern java#builder pattern vs factory pattern
0 notes
Text
#design patterns#design pattern tutorial#design pattern#design patterns tutorial#builder design pattern#design patterns in java#facade design pattern#design pattern video tutorial#head first design patterns#java design patterns#best design patterns#how to use design patterns#pattern#pattern design#head first: design patterns#strategy design pattern#decorator design pattern#bridge design pattern#simple design pattern#design patterns fast
0 notes
Text
#coding#code#programming#programmer#academia#geek#programmer humor#software#software engineering#teaching
2 notes
·
View notes
Text
How Onerous Could or not it’s To Fully grasp Java? Review By way of Our Java Tutorial

Oracle provides a certification in Java foundations and a more advanced certificates in Oracle Java SE 8 Programmer. Certification with Oracle provides aspiring coders recognized acknowledgement of your expertise. Regardless of the chosen platform, Java is simple to start, but fairly an in depth language to grasp.
How can I learn Java quickly?
Top tips for Learning Java Programming 1. Learn the Basics. As with anything, knowing the basics about Java is the best place to start.
2. Practice Coding. To use the old cliché, practice makes perfect.
3. Set Your Algorithm Carefully.
4. Trace Your Codes on Paper.
5. Read Sources on Java Programming Regularly.
This implies that Java will sometimes have better performance. Explore programs of your pursuits with the high-quality requirements and suppleness you want to take your profession to the next stage. With the Knowledge Map, you will get a holistic image of the educational content material and see how all of the subjects are connected. Tracking your progress will assist you to achieve a greater understanding of what you’ve done and what you proceed to have to study to find a way to spherical out your knowledge.
Java On-line Programs
This design feature permits the builders to construct interactive purposes that may run easily. Platform Independent − Unlike many other programming languages together with C and C++, when Java is compiled, it isn't compiled into platform specific machine, quite into platform impartial byte code. This byte code is distributed over the online and interpreted by the Virtual Machine on whichever platform it is being run on. If you are a beginner at programming or know a programming language but also wish to study Java, then you'll have the ability to enroll on this course. It’s FREE and I guess it always stays free on Udemy and you should be part of this to study Java2021.
Captivate sponsors along with your story and they will be more inclined to fund your learning journey. In this course, you will get hands-on experience in creating video games. Well, this course will educate you the means to create a replica of this annoyingly satisfying sport. If they do not discover answers, this setback incessantly pushes people to quit learning Java . With the elevated variety of resources available, it becomes more difficult to find a finding out resolution that works for all. Simply put, the idea of the greatest way to be taught Java does not exist, but some choices might be the best for specific folks.
The Final Word Playbook On Technology Ability Development
There are hundreds of full, working examples and dozens of lessons. In my early days with Java, I always follow this e-book to be taught and practice. It helped me so much in my approach to turn into a professional Java developer. Another web site with utterly free programs for learning Java.
It provides free programs of Java programming language for both beginners and experienced programmers.
Projects Practice expertise in real-world eventualities utilizing your personal developer workspace.
Fear not - in this quick information, we are going to walk you thru the most effective books, programs, and on-line communities that’ll give you confidence and a way of direction in Java learning.
The majority of enormous enterprises heavily rely on Java for their back-end architecture. This has made builders contemplate optimizing their possibilities of success in Java by enrolling in on-line programs with Certificates. CodeJava.internet shares Java tutorials, code examples and pattern tasks for programmers in any respect ranges.
In this on-line java programming course free, the lectures and labs go beyond basic syntax by together with best practices and object oriented programming idioms. In addition, the subjects coated helps in getting ready for the Oracle Certified Associate, Java Programmer SE 8 exam . Java is an advanced programming language which is more appropriate for older youngsters to learn.
youtube
1 note
·
View note
Text
Apakah kita membutuhkan Builder Pattern di bahasa Kotlin?
Mungkin beberapa dari pembaca tidak mengenal pattern ini, tapi pasti pernah menemukannya dalam codebase kalian. Builder pattern dapat diidentifikasi dengan mudah ketika menemukan code seperti dibawah ini.
val human = CreatureBuilder() .addArm(2) .addLeg(2) .addBody() .addHead() .build()
atau juga
val groupLayout = LayoutBuilder() val component1 = Component() val component2 = Component() groupLayout.addComponent(component1) groupLayout.addComponent(component2) val layout = groupLayout.create()
Apakah kita masih membutuhkannya? Jawaban singkatnya, menurut saya, ialah “Tidak”. Jawaban ini mungkin debatable, tapi mari saya coba jelaskan terlebih dahulu.
Dalam bahasa pemograman, kita mengenal istilah fungsi constructor untuk sebuah class. Fungsi tersebut bisa memiliki berbagai parameter, yang kemudian melakukan assignment terhadap properti yang dimiliki class tersebut. Namun, jika sebuah class memiliki sangat banyak properti, besar kemungkinan fungsi constructor ini menjadi sangat panjang, padahal belum tentu kita butuh untuk mengubah semua nilai properti yang dimiliki. Misalnya sebuah kelas manusia seperti dibawah.
class Human { int armCount; int legCount; int eyeCount; int liverCount; int age; double height; double weight; Human( int armCount, int legCount, int eyeCount, int liverCount, int age, double height, double weight ) { } }
Untuk hampir semua manusia, kemungkinan kita hanya perlu mengubah age, height, dan weight. Namun, kita tetap harus memberikan semua nilai yg diminta constructor. Ketika ingin menginisiasi hanya dengan parameter tertentu, kita perlu membuat overload-nya. Itu berarti ada 128 (2^7) kombinasi constructor yang dibutuhkan, belum lagi jika class human bukan milik kita. Sehingga menambah fungsi sebanyak itu sangat tidak mungkin. Akhirnya, munculah Builder pattern ini, kita cukup membuat satu fungsi tambahan untuk semua properti yang dibutuhkan. Berarti, hanya 7 fungsi tambahan.
class HumanBuilder { int armCount = 0; int legCount = 0; int eyeCount = 0; int liverCount = 0; int age = 0; double height = 0; double weight = 0; HumanBuilder addArm(int count); HumanBuilder addLeg(int count); HumanBuilder addEye(int count); HumanBuilder addLiver(int count); HumanBuilder addAge(int count); HumanBuilder addHeight(int count); HumanBuilder addWeight(int count); Human build() { return new Human( armCount, legCount, eyeCount, liverCount, age, height, weight ); } }
Pattern ini membantu kita untuk membuat object dan hanya mengatur properti yang kita inginkan. Lalu membiarkan sisanya bernilai default.
Tapi dengan adanya konsep named parameter dan default value parameter. Semua kesulitan ini menjadi hilang. Dalam bahasa Kotlin, kita cukup menulis..
class Human ( val armCount: Int = 0, val legCount: Int = 0, val eyeCount: Int = 0, val liverCount: Int = 0, val age: Int = 0, val height: Double = 0, val weight: Double = 0 ) // usage fun main() { val a = Human() val b = Human(age = 10) val c = Human(armCount = 2, height = 100) }
Kita cukup mendefinisikan 1 constructor dan kita memiliki semua macam konfigurasi yang kita inginkan, sangat mudah. Hal inilah mengapa wajar bayak programmer "baru", melupakan pattern 1 ini. Karena mareka sudah terbiasa dengan kemudahan. Bukan karena mereka malas, sehingga tidak belajar. Hanya saja perkembangan teknologi memang sudah tidak membutuhkannya.
Tapi bagaimana dengan pattern Director - Builder. Dimana terdapat sebuah common builder yang kemudian di-direct step buildnya oleh director? Jawaban singkatnya, kita bisa beralih ke pattern lain, seperti factory pattern.
Cukup sekian.. ciao~~
1 note
·
View note
Text
FineReport best free data visualization software
Introduction:
While talking about data visualization software, there is a variety of them in the market. But it is not like every software that your eyes see is perfect or has all the features. So precisely, you need to be a little picky while choosing a data visualization software for yourself. Though there is much software available we at takethiscourse.net are going to talk about FineReport data visualization software that is available for anyone to take for free. This free software is quite flexible to integrate with different business systems which make data visualization in other systems easy.
FineReport data visualization software:
FineReport is a Business Intelligence reporting and dashboard software. It is highly suitable for managers, builders, and IT staff. And helps you create dashboards and reports using extraordinary data visualization effects. The software comes with a variety of features that are necessary to know if you want to use this software. So let us get to the features without wasting any time.
Features of FineReport software:
This high-quality software has the following features to offer.
Innovative design patterns:
FineReport designers offer three reporting design modes. With the help of these three designs, you can make impressive reports and dashboards. These reports and dashboards can be made in less than ten minutes. Thus this feature not only lets you make fantastic reports/dashboards but also in minimum time.
Fast data integration:
As finereport supports all mainstream databases. Which then means that you can combine data from different sources easily. Precisely, you can make comprehensive analyses with just one click using the finereport software.
Cool data visualization:
With all the features you get through this software. You also get access to 19 categories and 50 plus styles of self-developed HTML charts. Accompanying cool 3D and dynamic effects as well.
Smart data entry:
The software providing rich controls make it easier for you to input piles of data via forms directly into databases. Using thoughtful functions like data validation and temporary storage, it gets less difficult for you to input data.
Powerful decision-making system:
The web portal that finereport offers for enterprise reporting enables a very secure environment for many reports. Similar is the case with access control and automated reporting, etc which makes the decision-making system quite authentic and powerful.
Easy deployment and integration:
Finereport is known to be a 100% JAVA software. This means that it can be seamlessly integrated with a variety of systems through independent or embedded deployment.
Convenient mobile app:
As the software offers several features that are actually useful. Another one is providing convenient access to monitor business performance through mobile. When you have this option of updating the data and monitoring all the other business performance. It can get really easy for you to work more effectively and thus producing better results.
Large screen & dashboard:
By providing large screens and dashboards, you can without any further trouble track KPIs from many systems. These business systems include ERP, OA, and MES. By tracking KPIs from these different business systems, you can gain insights into these data and then act accordingly.
Conclusion:
Taking a good look at these features will convince you easily to use the Fine report data visualization software. So if you are a manager or a builder or belongs to an IT department, using this free software for making interactive reports and dashboards will be a great idea. So look at these features again and then decide whether this free finereport data visualization software is what you need in your organization or not. In Tableau desktop certification you will learn all aspects that mentioned above .
#Tableau dekstop specialist#tableau certification exam#tableau desktop certification#Tableau Server Certification#tableau exam#Tableau practice exams#Tableau certification dumps
1 note
·
View note
Link
0 notes
Text
7 Helpful Tricks to Making the Most of Your Java
Certainly! Here are seven helpful tricks to make the most of your Java programming:
Use Java Generics: Generics allow you to write reusable code by creating classes and methods that can work with different data types. This helps in achieving type safety and can prevent runtime errors. By leveraging generics, you can write more flexible and robust code.
Utilize Java Collections: The Java Collections Framework provides a set of interfaces and classes that make it easier to work with groups of objects. By using collections such as ArrayList, LinkedList, HashMap, or TreeSet, you can efficiently store, retrieve, and manipulate data. Understanding and utilizing these collections can greatly simplify your code.
Master Exception Handling: Exception handling is crucial for writing reliable and robust code. By properly handling exceptions, you can gracefully handle unexpected situations and prevent your program from crashing. Learn about different types of exceptions, use try-catch blocks, and make use of the finally block for cleanup tasks.
Apply Multithreading: Java offers robust multithreading capabilities, allowing you to perform multiple tasks concurrently. By using threads, you can improve the performance of your applications and make them more responsive. However, be mindful of synchronization issues and ensure thread safety when accessing shared resources.
Optimize with Java Streams: Java 8 introduced the Stream API, which provides a functional programming style for processing collections of data. Streams allow you to perform powerful operations like filtering, mapping, reducing, and sorting on data elements. By leveraging streams, you can write concise and expressive code while taking advantage of parallel processing when appropriate.
Take Advantage of Java Annotations: Annotations provide metadata about code elements and can be used for various purposes. They can be used for documentation, code generation, configuration, and more. Familiarize yourself with built-in annotations like @Override, @Deprecated, and @SuppressWarnings. Additionally, explore creating custom annotations for specific use cases.
Apply Design Patterns: Design patterns are proven solutions to common programming problems. They provide a structured approach to designing and organizing your code. Familiarize yourself with popular design patterns like Singleton, Observer, Factory, and Builder. Applying these patterns can improve the modularity, maintainability, and scalability of your Java code.
0 notes
Text
#adapter design pattern#adapter design pattern in java#adapter design pattern example#design patterns#Real example of adapter design pattern#adapter design pattern with java example#adapter design pattern for beginners#adapter design pattern with real example#adapter design pattern java#adapter pattern in java#adapter pattern with example#adapter pattern in software engineering adapter pattern in OOAD#adapter pattern explained#java design pattern#design pattern with example#abstract factory design pattern#prototype design pattern#builder design pattern#factory design pattern#singleton design pattern#structural design pattern#bridge design pattern#composite design pattern#decorator design pattern#facade design pattern#flyweight design pattern#proxy design pattern#behavior design patterns
0 notes
Text
Java making a contact book app

#Java making a contact book app update
#Java making a contact book app for android
#Java making a contact book app code
#Java making a contact book app series
Community support: Since Java is an older programming language, it has a large community of developers that share valuable insights and knowledge when it comes to Java app development.Ĭhapter #2: Must-Have for Java Mobile DevelopmentĪs discussed in the previous chapter, one of the greatest benefits to using Java is its wealth of open-source tools and libraries.Open-source: Over the years, Java has amassed a great collection of open-source libraries that make java mobile apps much easier to develop.For example, a Java app can serve users a login form while also running background processes. Multi-threaded: With Java you can write applications that conduct multiple tasks in separate threads.High-performing: While Java is an interpreted language, unlike C or C++ which are compiled, it nonetheless is high performing due to its own just-in-time compiler.Secure: Since Java is platform independent and has almost no interaction with the operating system, it makes the language much more secure than other programming languages.
#Java making a contact book app code
Platform independent: Since Java programs are first converted to bytecode by the Java compiler, the code can run on any machine that supports the Java runtime environment-making it platform-independent.Everything is an “object” that contains code and data. Object-oriented: Just like with Python, C++, and Ruby, Java is an object-oriented programming language.Java has many features that make it a popular choice among both web and mobile app developers. This language uses syntax that comes from other languages like C and C++. Java was originally created by Sun Microsystems, which has since become Oracle Corporation. In short, it’s always embraced the concept of “write once, run anywhere”. Java is a class-based, object-oriented programming language that was created to carry as few dependencies as possible so the compiled Java code could run on all Java-supported platforms without the need for recompilation. In this article, we’ll review why Java is still many developer’s go-to programming language and how it stacks up against other popular languages for Android, like Kotlin.
#Java making a contact book app for android
Now, I hope you’ll have fun! Start with Part 1: Scene Builder.Even with the emergence of Kotlin as the new preferred language for Android mobile app development since 2019, Java is still widely used. Then go through the tutorial to understand the code.
fast track: Import the source code for a tutorial part into your IDE (it’s an Eclipse project, but you could use other IDEs like NetBeans with slight modifications).
learn-a-lot track: Create your own JavaFX project from the ground up.
#Java making a contact book app series
This is quite a lot! So, after you you’ve completed this tutorial series you should be ready to build sophisticated applications with JavaFX.
Deploying a JavaFX application as a native package.
Saving the last opened file path in user preferences.
Create a custom popup dialog to edit persons.
Using TableView and reacting to selection changes in the table.
Using ObservableLists for automatically updating the user interface.
Structuring an application with the Model-View-Controller (MVC) pattern.
Using Scene Builder to design the user interface.
This is how the final application will look like: This tutorial walks you through designing, programming and deploying an address application.
#Java making a contact book app update
So I decided to rewrite the JavaFX 2 tutorial for JavaFX 8 (read about what changed in Update to JavaFX 8 - What’s New). Many people all over the world have been reading the tutorial and gave very positive feedback. Back in 2012 I wrote a very detailed JavaFX 2 tutorial series for my students.

0 notes