#Design patterns
Explore tagged Tumblr posts
Text
Coding tutorial: Chain-of-responsibility pattern
A peasant, knight and king walk into a software design tutorial, and are here to teach you the chain-of-responsibility pattern. Learn how to create a chain of handlers which can handle different request types.
This tutorial shows you how to code the chain-of-responsibility pattern in the Visual Studio development environment, using a console application and the C++ programming language.
The chain-of-responsibility pattern passes on a request to a chain of handlers one at a time. Each handler can handle different requests. So if the first handler can’t handle the request, then it will pass it on to the next handler. Once a request is handled, the chain ends. Since there is no longer a need to pass on the request.
It is also possible that the request doesn’t get handled by any of the handlers. Since each handler can handle 0, 1 or many requests of different types.
See the full tutorial here.
Console output:
#cpp#coding#programming#gamedev#indiedev#tutorial#visual studio#software#software design#software development#game development#design patterns#cppprogramming
20 notes
·
View notes
Text

𝗁𝗍𝗍𝗉s://instagram.com/sarahsignslondon
11 notes
·
View notes
Note
40 for the ask game
40. what’s the most interesting item you own?
hmmmmmmmm……
Ooh! Not sure if this is a good answer, but in college when I bought the cheapest used copy of the famous Go4 Design Patterns textbook I could find, I was surprised to find that it had been signed by 3 of the 4 authors!

just needs Erich Gamma to complete the set!
full list for those who want to play
2 notes
·
View notes
Text
Normally I just post about movies but I'm a software engineer by trade so I've got opinions on programming too.
Apparently it's a month of code or something because my dash is filled with people trying to learn Python. And that's great, because Python is a good language with a lot of support and job opportunities. I've just got some scattered thoughts that I thought I'd write down.
Python abstracts a number of useful concepts. It makes it easier to use, but it also means that if you don't understand the concepts then things might go wrong in ways you didn't expect. Memory management and pointer logic is so damn annoying, but you need to understand them. I learned these concepts by learning C++, hopefully there's an easier way these days.
Data structures and algorithms are the bread and butter of any real work (and they're pretty much all that come up in interviews) and they're language agnostic. If you don't know how to traverse a linked list, how to use recursion, what a hash map is for, etc. then you don't really know how to program. You'll pretty much never need to implement any of them from scratch, but you should know when to use them; think of them like building blocks in a Lego set.
Learning a new language is a hell of a lot easier after your first one. Going from Python to Java is mostly just syntax differences. Even "harder" languages like C++ mostly just mean more boilerplate while doing the same things. Learning a new spoken language in is hard, but learning a new programming language is generally closer to learning some new slang or a new accent. Lists in Python are called Vectors in C++, just like how french fries are called chips in London. If you know all the underlying concepts that are common to most programming languages then it's not a huge jump to a new one, at least if you're only doing all the most common stuff. (You will get tripped up by some of the minor differences though. Popping an item off of a stack in Python returns the element, but in Java it returns nothing. You have to read it with Top first. Definitely had a program fail due to that issue).
The above is not true for new paradigms. Python, C++ and Java are all iterative languages. You move to something functional like Haskell and you need a completely different way of thinking. Javascript (not in any way related to Java) has callbacks and I still don't quite have a good handle on them. Hardware languages like VHDL are all synchronous; every line of code in a program runs at the same time! That's a new way of thinking.
Python is stereotyped as a scripting language good only for glue programming or prototypes. It's excellent at those, but I've worked at a number of (successful) startups that all were Python on the backend. Python is robust enough and fast enough to be used for basically anything at this point, except maybe for embedded programming. If you do need the fastest speed possible then you can still drop in some raw C++ for the places you need it (one place I worked at had one very important piece of code in C++ because even milliseconds mattered there, but everything else was Python). The speed differences between Python and C++ are so much smaller these days that you only need them at the scale of the really big companies. It makes sense for Google to use C++ (and they use their own version of it to boot), but any company with less than 100 engineers is probably better off with Python in almost all cases. Honestly thought the best programming language is the one you like, and the one that you're good at.
Design patterns mostly don't matter. They really were only created to make up for language failures of C++; in the original design patterns book 17 of the 23 patterns were just core features of other contemporary languages like LISP. C++ was just really popular while also being kinda bad, so they were necessary. I don't think I've ever once thought about consciously using a design pattern since even before I graduated. Object oriented design is mostly in the same place. You'll use classes because it's a useful way to structure things but multiple inheritance and polymorphism and all the other terms you've learned really don't come into play too often and when they do you use the simplest possible form of them. Code should be simple and easy to understand so make it as simple as possible. As far as inheritance the most I'm willing to do is to have a class with abstract functions (i.e. classes where some functions are empty but are expected to be filled out by the child class) but even then there are usually good alternatives to this.
Related to the above: simple is best. Simple is elegant. If you solve a problem with 4000 lines of code using a bunch of esoteric data structures and language quirks, but someone else did it in 10 then I'll pick the 10. On the other hand a one liner function that requires a lot of unpacking, like a Python function with a bunch of nested lambdas, might be easier to read if you split it up a bit more. Time to read and understand the code is the most important metric, more important than runtime or memory use. You can optimize for the other two later if you have to, but simple has to prevail for the first pass otherwise it's going to be hard for other people to understand. In fact, it'll be hard for you to understand too when you come back to it 3 months later without any context.
Note that I've cut a few things for simplicity. For example: VHDL doesn't quite require every line to run at the same time, but it's still a major paradigm of the language that isn't present in most other languages.
Ok that was a lot to read. I guess I have more to say about programming than I thought. But the core ideas are: Python is pretty good, other languages don't need to be scary, learn your data structures and algorithms and above all keep your code simple and clean.
#programming#python#software engineering#java#java programming#c++#javascript#haskell#VHDL#hardware programming#embedded programming#month of code#design patterns#common lisp#google#data structures#algorithms#hash table#recursion#array#lists#vectors#vector#list#arrays#object oriented programming#functional programming#iterative programming#callbacks
19 notes
·
View notes
Text
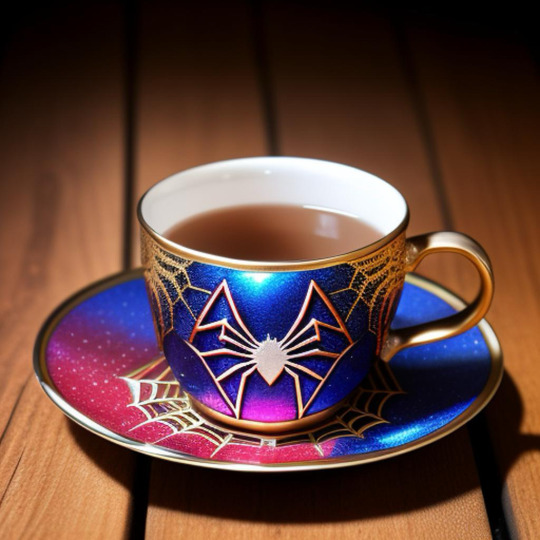

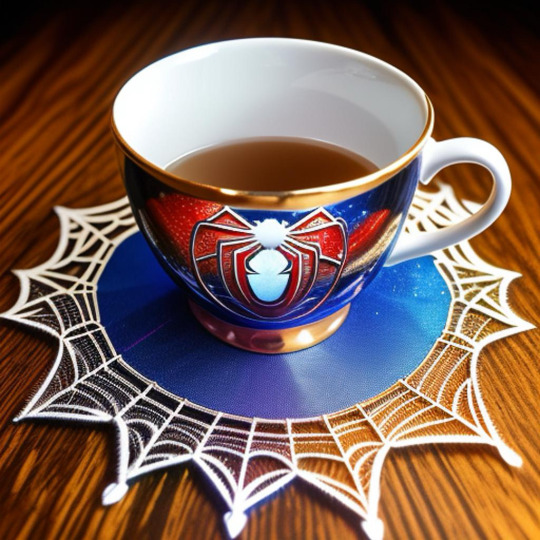
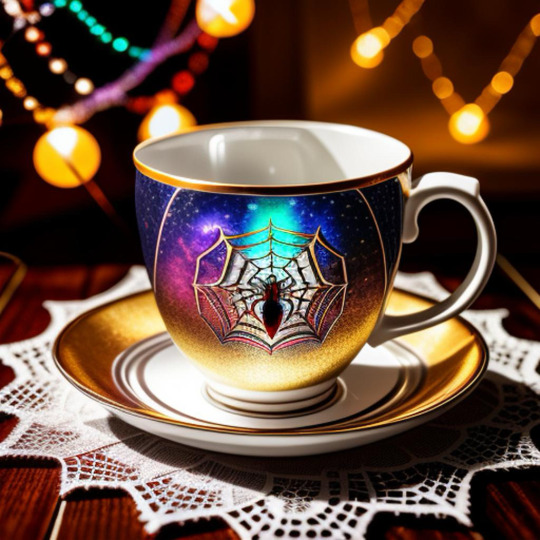
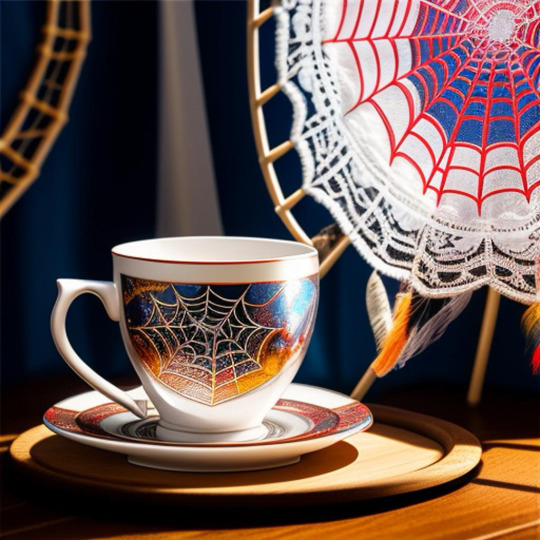
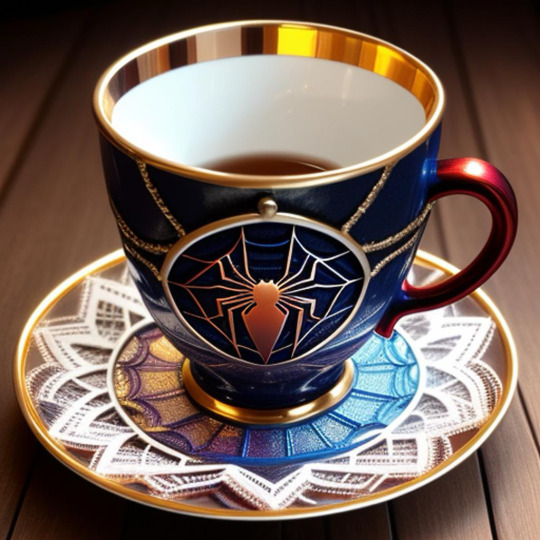

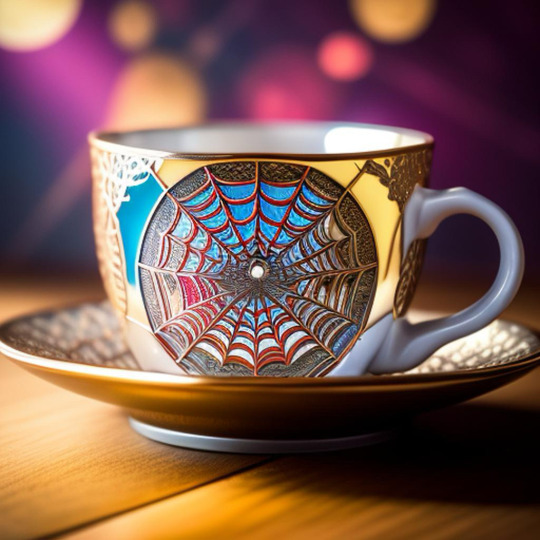
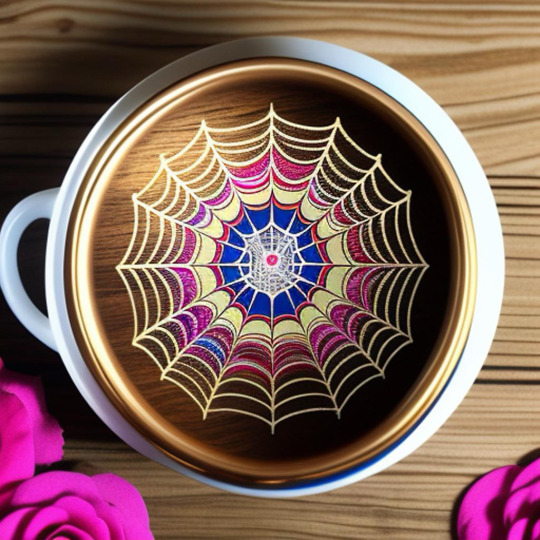
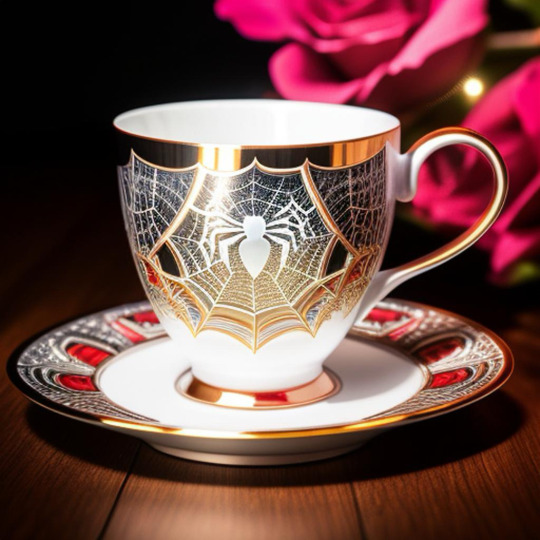
I wasn’t a die hard fan of Spidey when the 2000’s and my teenage years collided back then.
#ai art#ai artwork#ai art experimenting#ai art practicing#ai art generated#ai art generator#keywords regenerated#picsart#hobby#habit#spider man phase#spider webs#marvel#tea cups#embroidery#design patterns#dream catchers#hoops#silverware#porcelain cups#tea sets#glassware#tea time#spider symbol#coffee tables#tea party#roses#expensive#outdoor lighting#plates
4 notes
·
View notes
Text
Records and data transfer in Java
So a colleague of mine was curious about the Data Transfer Object pattern, DTOs and specifically Records in Java, so I decided to write smth about that. You can find it here:
#coding#codeblr#development#developers#code#ladyargento#web development#webdev#programming#java#design patterns#learning
4 notes
·
View notes
Text

Glemt’s design page!
#punypapy#tadc original character#tadc oc#the digital circus#oc backstory#ocs#oc artist#oc design#oc art#my ocs#my art#the amazing digital circus oc#digital circus#design#design patterns#design page#glemt
3 notes
·
View notes
Text
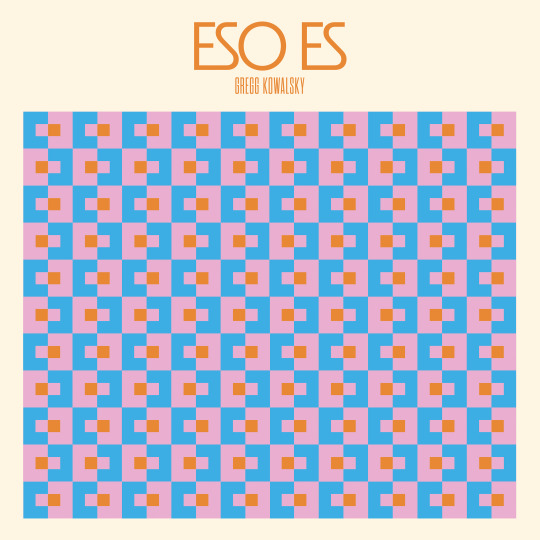

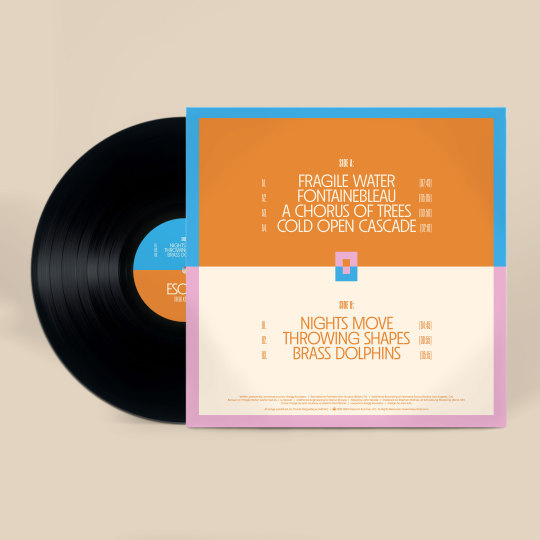
#mexican summer#ambient#gregg kowalsky#electronic music#drone#Eso Es#album cover#album art#graphic design#design patterns
3 notes
·
View notes
Photo
my copy of the gang of four Design Patterns book is signed by 3/4 authors
it was just the random used copy i got when i took Design Patterns in college

449K notes
·
View notes
Text



Started a new vest project this week ! Test swatch + bottom section ⛪🌿
#knitting#tracery vest#wipinsanity#<--- this is the pattern designer you can find them on ravelry and their own site fyi#textile#bottom section is normally tall corrugated ribs but I wanted to change propotions a little#so i joined the ribbing into archways and added a row of windows
29K notes
·
View notes
Text
Oscar de la Renta: 'Crafted like a mosaic, discover the making-of the #odlrfall2024 stained glass gown — ushering in a a new House-signature embroidery technique.'

Constructed from hundreds of polyamide panes, hand-sewn together in an Art Nouveau style reminiscent of Tiffany glass. Ready-to-wear: £36,546.


#oscar de la renta#fashion#savoir faire#metiers d'art#video#process video#fall 2024#stained glass#couture embroidery#construction#atelier#2024#pattern#surface pattern#surface pattern design#pattern design#textile design#textiles#wisteria#flowers#floral#polyamide#plastic#art nouveau#tiffany glass#louis comfort tiffany#art history#design history
32K notes
·
View notes
Text
Coding tutorial: Observer pattern
The observer pattern has an object named the ‘subject’ which maintains a list of ‘observers’. The subject will notify all the observers when an event occurs. The observers can then choose how they wish to respond to the event.
In this tutorial you will code a zookeeper and animals. The zookeeper represents the subject of the observer pattern. Whilst the animals represent the observers.
The zookeeper will notify the animals when he arrives, and the animals will respond in there own unique way.
To follow along to this tutorial, you can either just read it and apply the knowledge to your programming language. Or you can use Visual Studio, by creating a solution, then create a project with a console application. Then run the project to see the output in the console window.
Walkthrough and full code example on the blog:
#cpp#programming#coding#gamedev#indiedev#visual studio#tutorial#software#software design#software development#design patterns
24 notes
·
View notes
Text
Toast Cross Stitch Pattern – A Tasty Trio for Your Kitchen Stitch Wall
Start your day the stitchy way with this adorable Toast Cross Stitch Pattern, featuring a delicious breakfast trio: egg, avocado, and salmon on toast! Whether you’re a brunch lover or just obsessed with food-themed stitching, this modern kitchen-friendly design is the perfect way to add some flavor to your embroidery collection.

Clean lines and bright colors make this a satisfying and cheerful piece to stitch—ideal for gifting, kitchen décor, or adding a foodie twist to your crafting corner. This is a PDF cross stitch pattern available instantly on Etsy, so you can get started on your breakfast stitch without delay!
Pattern Details:
Design: Three toast toppings – egg, avocado, and smoked salmon 🥑🍳🐟
Style: Modern, minimalist, and oh-so-cute!
Format: Instant PDF download
Includes:
Color & symbol charts
List of DMC thread colors
Easy-to-read layout for phone, tablet, or print
Note: This is a digital pattern only—no physical items (fabric/floss) are included.
🔗❤️Grab your favorite Aida cloth and stitch up this kitchen-ready Toast Cross Stitch Chart—available now on Etsy.
Hashtags: #ToastCrossStitch #BreakfastEmbroidery #ModernCrossStitch #KitchenDecorCrafts #PDFCrossStitchPattern #AvocadoToast #EggCrossStitch #SalmonOnToast #BrunchVibes #StitchTheKitchen #EtsyPatternFinds #FoodieCrossStitch #MinimalistEmbroidery #CuteCrossStitch #CrossStitchLove #DIYKitchenDecor
Cross Stitch and Joy may earn a small commission at no extra cost to you via trusted partners and affiliates. Availability is accurate as of time blog post date/time.
#cross stitch#creative design#diy crafts#stitiching#toast#breakfast#kitchen decor#wall art#etsy#etsy finds#patterns#design patterns#embrodiery#needlework
0 notes
Text
Abstraction in design patterns
Abstraction in design patterns is a fundamental concept that focuses on hiding complex implementation details and exposing only essential information or functionalities. It allows you to work with objects or systems at a higher level of understanding, without needing to know how they work internally. Think of it like driving a car: you know how to steer, accelerate, and brake, but you don't need to understand the intricate workings of the engine, transmission, or other internal components to drive effectively.
Here's a breakdown of what abstraction means in the context of design patterns:
Simplification: Abstraction simplifies complex systems by breaking them down into manageable, understandable units. It reduces cognitive overload by focusing on what an object does, not how it does it.
Generalization: Abstraction allows you to treat different objects in a uniform way, as long as they share a common interface or abstract class. This promotes code reusability and flexibility. For example, you might have different types of payment processors (credit card, PayPal, etc.), but you can interact with them through a common "PaymentProcessor" interface.
Information Hiding: Abstraction hides the internal state and implementation details of an object from the outside world. This protects the integrity of the object and prevents external code from becoming dependent on specific implementation details, which could make the system brittle and difficult to change.
Creating a Contract: An abstract class or interface defines a contract that concrete classes must adhere to. This ensures consistency and predictability in how objects interact. Anyone using an object that implements a specific interface knows what methods to expect and how they will behave.
Enabling Polymorphism: Abstraction is crucial for polymorphism, which allows objects of different classes to be treated as objects of a common type. This is a powerful concept that enables flexible and extensible designs.
How Abstraction is Used in Design Patterns:
Many design patterns rely heavily on abstraction. Here are a few examples:
Factory Pattern: The Factory pattern abstracts the process of object creation. Instead of directly instantiating concrete classes, you ask a factory to create the objects for you. This decouples the client code from the specific classes being created.
Strategy Pattern: The Strategy pattern allows you to choose an algorithm at runtime. The different algorithms are abstracted behind a common interface, so the client code can switch between them without needing to know the specific implementation of each algorithm.
Facade Pattern: The Facade pattern provides a simplified interface to a complex subsystem. It hides the complexity of the subsystem behind a single, easy-to-use object.
Observer Pattern: The Observer pattern allows objects to be notified of changes in the state of another object. The details of how the notification is implemented are abstracted away, so the observer doesn't need to know how the subject manages its state.
In summary: Abstraction in design patterns is about creating simplified views of complex systems, hiding implementation details, and focusing on essential functionalities. It's a powerful tool for building flexible, maintainable, and reusable code.
1 note
·
View note