#Data structures
Explore tagged Tumblr posts
Text
so I'm reading Gankra's "Learn Rust With Entirely Too Many Linked Lists" and the introduction feels like
STOP USING LINKED LISTS
DATA ELEMENTS WERE NOT SUPPOSED TO BE GIVEN POINTERS
YEARS OF COMPUTER SCIENCE yet NO REAL-WORLD USE FOUND for using anything other than Vec
Want to add and remove elements from the front and back just for a laugh? We have a tool for that: It's called "VecDeq"
"It might take a long time to look at any element but I'll make it up with all the merges, inserts, and splits I'll be doing" - Statements dreamed up by the utterly Deranged
LOOK at what Functional Programmers have been demanding your Respect for all this time, with all the LISP machines & tape readers we built for them (This is REAL Computer Science, done by REAL Computer Scientists)
???????????
"Hello I would like element.next.next.next.next.next.next.next.next please"
They have played us for absolute fools
165 notes
·
View notes
Text
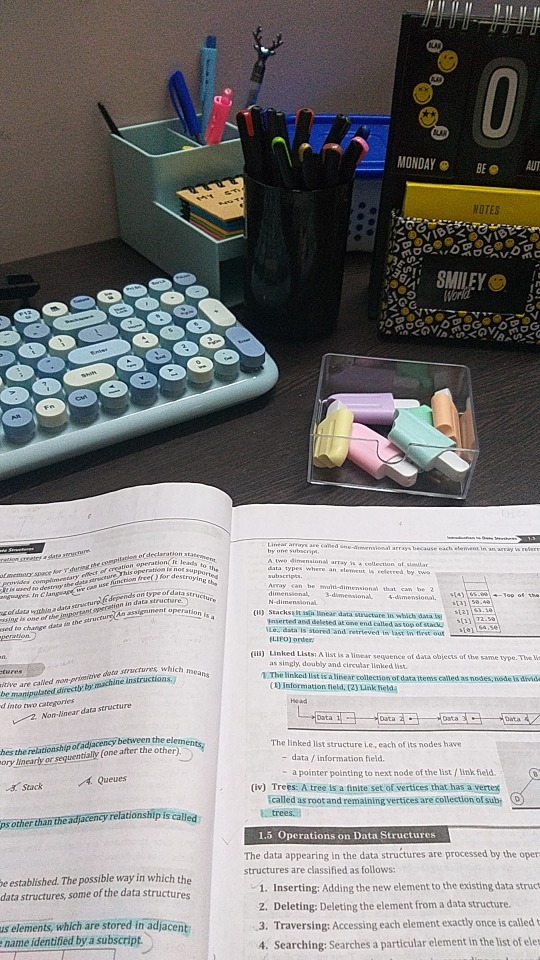
August 7, 2023
Uni 'A' has begun! Today we were introduced to data structures, and the teacher's accent was so heavy I zoned out too many times T-T
Planning on doing a pre-read before her class so it's more of a recall session rather than learning it new.

Things I did today:
Recalled basics of C programming on a sheet of paper
Light reading on introduction to data structures
Created a new template for my digital planner

@studaxy and I had a very productive 40 minutes :D
The days ahead look bright :)
🎧I Know Places - Taylor Swift
#dailyfoxposts#studyblr#codeblr#coding#studyspo#study#note taking#computer science#data structures#university
105 notes
·
View notes
Text
What is Data Structure in Python?
Summary: Explore what data structure in Python is, including built-in types like lists, tuples, dictionaries, and sets, as well as advanced structures such as queues and trees. Understanding these can optimize performance and data handling.
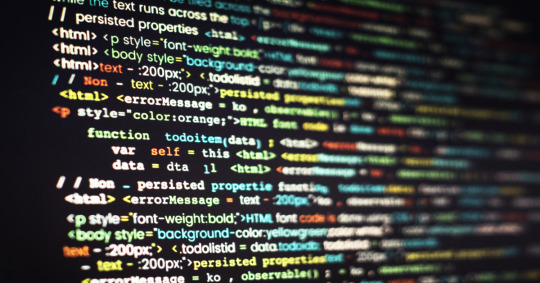
Introduction
Data structures are fundamental in programming, organizing and managing data efficiently for optimal performance. Understanding "What is data structure in Python" is crucial for developers to write effective and efficient code. Python, a versatile language, offers a range of built-in and advanced data structures that cater to various needs.
This blog aims to explore the different data structures available in Python, their uses, and how to choose the right one for your tasks. By delving into Python’s data structures, you'll enhance your ability to handle data and solve complex problems effectively.
What are Data Structures?
Data structures are organizational frameworks that enable programmers to store, manage, and retrieve data efficiently. They define the way data is arranged in memory and dictate the operations that can be performed on that data. In essence, data structures are the building blocks of programming that allow you to handle data systematically.
Importance and Role in Organizing Data
Data structures play a critical role in organizing and managing data. By selecting the appropriate data structure, you can optimize performance and efficiency in your applications. For example, using lists allows for dynamic sizing and easy element access, while dictionaries offer quick lookups with key-value pairs.
Data structures also influence the complexity of algorithms, affecting the speed and resource consumption of data processing tasks.
In programming, choosing the right data structure is crucial for solving problems effectively. It directly impacts the efficiency of algorithms, the speed of data retrieval, and the overall performance of your code. Understanding various data structures and their applications helps in writing optimized and scalable programs, making data handling more efficient and effective.
Read: Importance of Python Programming: Real-Time Applications.
Types of Data Structures in Python
Python offers a range of built-in data structures that provide powerful tools for managing and organizing data. These structures are integral to Python programming, each serving unique purposes and offering various functionalities.
Lists
Lists in Python are versatile, ordered collections that can hold items of any data type. Defined using square brackets [], lists support various operations. You can easily add items using the append() method, remove items with remove(), and extract slices with slicing syntax (e.g., list[1:3]). Lists are mutable, allowing changes to their contents after creation.
Tuples
Tuples are similar to lists but immutable. Defined using parentheses (), tuples cannot be altered once created. This immutability makes tuples ideal for storing fixed collections of items, such as coordinates or function arguments. Tuples are often used when data integrity is crucial, and their immutability helps in maintaining consistent data throughout a program.
Dictionaries
Dictionaries store data in key-value pairs, where each key is unique. Defined with curly braces {}, dictionaries provide quick access to values based on their keys. Common operations include retrieving values with the get() method and updating entries using the update() method. Dictionaries are ideal for scenarios requiring fast lookups and efficient data retrieval.
Sets
Sets are unordered collections of unique elements, defined using curly braces {} or the set() function. Sets automatically handle duplicate entries by removing them, which ensures that each element is unique. Key operations include union (combining sets) and intersection (finding common elements). Sets are particularly useful for membership testing and eliminating duplicates from collections.
Each of these data structures has distinct characteristics and use cases, enabling Python developers to select the most appropriate structure based on their needs.
Explore: Pattern Programming in Python: A Beginner’s Guide.
Advanced Data Structures
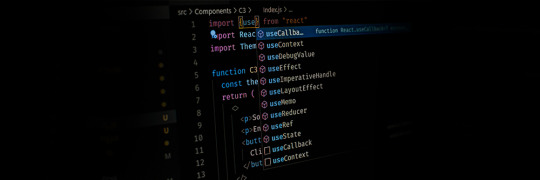
In advanced programming, choosing the right data structure can significantly impact the performance and efficiency of an application. This section explores some essential advanced data structures in Python, their definitions, use cases, and implementations.
Queues
A queue is a linear data structure that follows the First In, First Out (FIFO) principle. Elements are added at one end (the rear) and removed from the other end (the front).
This makes queues ideal for scenarios where you need to manage tasks in the order they arrive, such as task scheduling or handling requests in a server. In Python, you can implement a queue using collections.deque, which provides an efficient way to append and pop elements from both ends.
Stacks
Stacks operate on the Last In, First Out (LIFO) principle. This means the last element added is the first one to be removed. Stacks are useful for managing function calls, undo mechanisms in applications, and parsing expressions.
In Python, you can implement a stack using a list, with append() and pop() methods to handle elements. Alternatively, collections.deque can also be used for stack operations, offering efficient append and pop operations.
Linked Lists
A linked list is a data structure consisting of nodes, where each node contains a value and a reference (or link) to the next node in the sequence. Linked lists allow for efficient insertions and deletions compared to arrays.
A singly linked list has nodes with a single reference to the next node. Basic operations include traversing the list, inserting new nodes, and deleting existing ones. While Python does not have a built-in linked list implementation, you can create one using custom classes.
Trees
Trees are hierarchical data structures with a root node and child nodes forming a parent-child relationship. They are useful for representing hierarchical data, such as file systems or organizational structures.
Common types include binary trees, where each node has up to two children, and binary search trees, where nodes are arranged in a way that facilitates fast lookups, insertions, and deletions.
Graphs
Graphs consist of nodes (or vertices) connected by edges. They are used to represent relationships between entities, such as social networks or transportation systems. Graphs can be represented using an adjacency matrix or an adjacency list.
The adjacency matrix is a 2D array where each cell indicates the presence or absence of an edge, while the adjacency list maintains a list of edges for each node.
See: Types of Programming Paradigms in Python You Should Know.
Choosing the Right Data Structure
Selecting the appropriate data structure is crucial for optimizing performance and ensuring efficient data management. Each data structure has its strengths and is suited to different scenarios. Here’s how to make the right choice:
Factors to Consider
When choosing a data structure, consider performance, complexity, and specific use cases. Performance involves understanding time and space complexity, which impacts how quickly data can be accessed or modified. For example, lists and tuples offer quick access but differ in mutability.
Tuples are immutable and thus faster for read-only operations, while lists allow for dynamic changes.
Use Cases for Data Structures:
Lists are versatile and ideal for ordered collections of items where frequent updates are needed.
Tuples are perfect for fixed collections of items, providing an immutable structure for data that doesn’t change.
Dictionaries excel in scenarios requiring quick lookups and key-value pairs, making them ideal for managing and retrieving data efficiently.
Sets are used when you need to ensure uniqueness and perform operations like intersections and unions efficiently.
Queues and stacks are used for scenarios needing FIFO (First In, First Out) and LIFO (Last In, First Out) operations, respectively.
Choosing the right data structure based on these factors helps streamline operations and enhance program efficiency.
Check: R Programming vs. Python: A Comparison for Data Science.
Frequently Asked Questions
What is a data structure in Python?
A data structure in Python is an organizational framework that defines how data is stored, managed, and accessed. Python offers built-in structures like lists, tuples, dictionaries, and sets, each serving different purposes and optimizing performance for various tasks.
Why are data structures important in Python?
Data structures are crucial in Python as they impact how efficiently data is managed and accessed. Choosing the right structure, such as lists for dynamic data or dictionaries for fast lookups, directly affects the performance and efficiency of your code.
What are advanced data structures in Python?
Advanced data structures in Python include queues, stacks, linked lists, trees, and graphs. These structures handle complex data management tasks and improve performance for specific operations, such as managing tasks or representing hierarchical relationships.
Conclusion
Understanding "What is data structure in Python" is essential for effective programming. By mastering Python's data structures, from basic lists and dictionaries to advanced queues and trees, developers can optimize data management, enhance performance, and solve complex problems efficiently.
Selecting the appropriate data structure based on your needs will lead to more efficient and scalable code.
#What is Data Structure in Python?#Data Structure in Python#data structures#data structure in python#python#python frameworks#python programming#data science
5 notes
·
View notes
Text
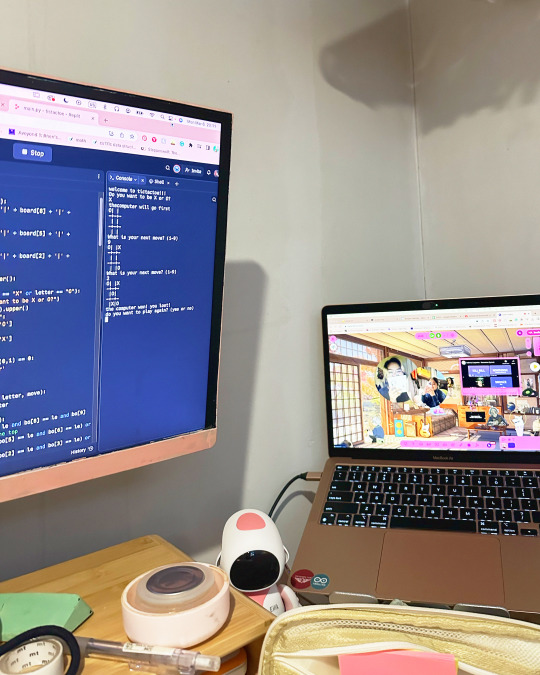
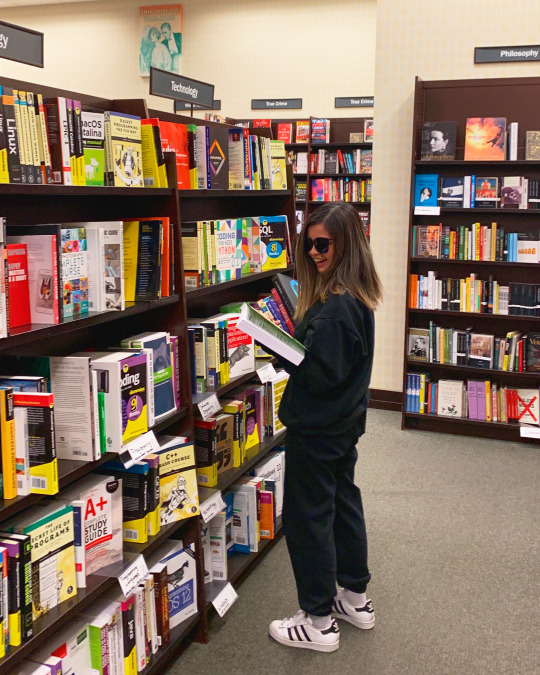
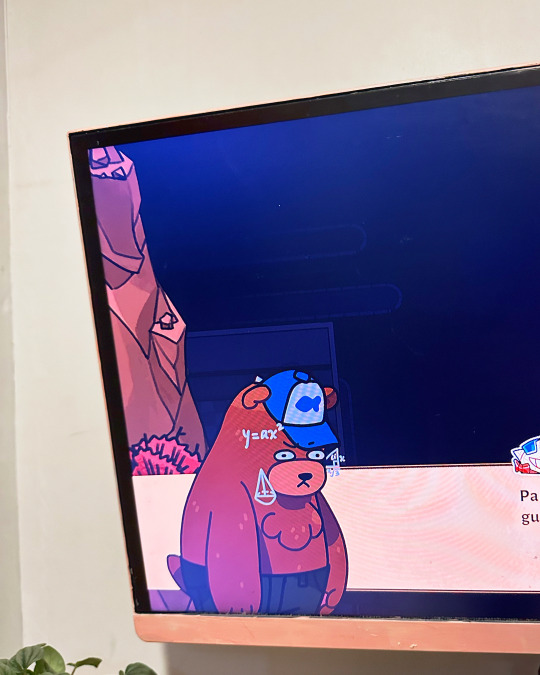
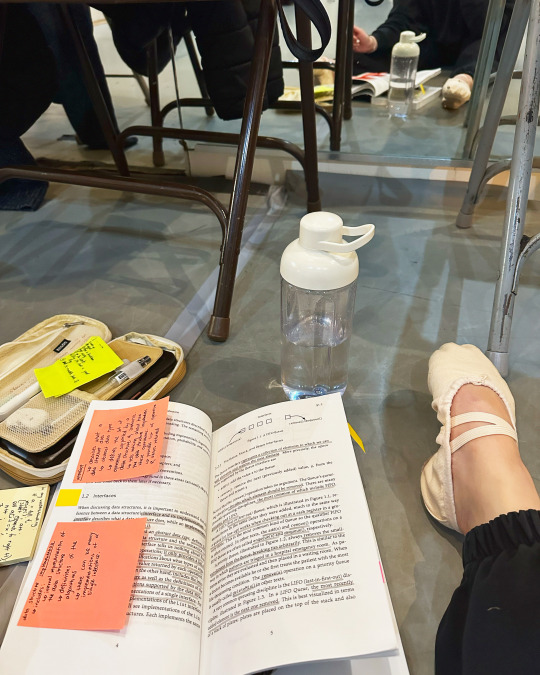
focus...
#computer science#comp sci#computer engineering#ballet#ballet student#engineering#math#math major#university#college student#studyblr#high achiever#study motivation#engineering major#women in stem#stem student#stem major#annotated books#data structures#algorithms#code#codeblr
130 notes
·
View notes
Text
Normally I just post about movies but I'm a software engineer by trade so I've got opinions on programming too.
Apparently it's a month of code or something because my dash is filled with people trying to learn Python. And that's great, because Python is a good language with a lot of support and job opportunities. I've just got some scattered thoughts that I thought I'd write down.
Python abstracts a number of useful concepts. It makes it easier to use, but it also means that if you don't understand the concepts then things might go wrong in ways you didn't expect. Memory management and pointer logic is so damn annoying, but you need to understand them. I learned these concepts by learning C++, hopefully there's an easier way these days.
Data structures and algorithms are the bread and butter of any real work (and they're pretty much all that come up in interviews) and they're language agnostic. If you don't know how to traverse a linked list, how to use recursion, what a hash map is for, etc. then you don't really know how to program. You'll pretty much never need to implement any of them from scratch, but you should know when to use them; think of them like building blocks in a Lego set.
Learning a new language is a hell of a lot easier after your first one. Going from Python to Java is mostly just syntax differences. Even "harder" languages like C++ mostly just mean more boilerplate while doing the same things. Learning a new spoken language in is hard, but learning a new programming language is generally closer to learning some new slang or a new accent. Lists in Python are called Vectors in C++, just like how french fries are called chips in London. If you know all the underlying concepts that are common to most programming languages then it's not a huge jump to a new one, at least if you're only doing all the most common stuff. (You will get tripped up by some of the minor differences though. Popping an item off of a stack in Python returns the element, but in Java it returns nothing. You have to read it with Top first. Definitely had a program fail due to that issue).
The above is not true for new paradigms. Python, C++ and Java are all iterative languages. You move to something functional like Haskell and you need a completely different way of thinking. Javascript (not in any way related to Java) has callbacks and I still don't quite have a good handle on them. Hardware languages like VHDL are all synchronous; every line of code in a program runs at the same time! That's a new way of thinking.
Python is stereotyped as a scripting language good only for glue programming or prototypes. It's excellent at those, but I've worked at a number of (successful) startups that all were Python on the backend. Python is robust enough and fast enough to be used for basically anything at this point, except maybe for embedded programming. If you do need the fastest speed possible then you can still drop in some raw C++ for the places you need it (one place I worked at had one very important piece of code in C++ because even milliseconds mattered there, but everything else was Python). The speed differences between Python and C++ are so much smaller these days that you only need them at the scale of the really big companies. It makes sense for Google to use C++ (and they use their own version of it to boot), but any company with less than 100 engineers is probably better off with Python in almost all cases. Honestly thought the best programming language is the one you like, and the one that you're good at.
Design patterns mostly don't matter. They really were only created to make up for language failures of C++; in the original design patterns book 17 of the 23 patterns were just core features of other contemporary languages like LISP. C++ was just really popular while also being kinda bad, so they were necessary. I don't think I've ever once thought about consciously using a design pattern since even before I graduated. Object oriented design is mostly in the same place. You'll use classes because it's a useful way to structure things but multiple inheritance and polymorphism and all the other terms you've learned really don't come into play too often and when they do you use the simplest possible form of them. Code should be simple and easy to understand so make it as simple as possible. As far as inheritance the most I'm willing to do is to have a class with abstract functions (i.e. classes where some functions are empty but are expected to be filled out by the child class) but even then there are usually good alternatives to this.
Related to the above: simple is best. Simple is elegant. If you solve a problem with 4000 lines of code using a bunch of esoteric data structures and language quirks, but someone else did it in 10 then I'll pick the 10. On the other hand a one liner function that requires a lot of unpacking, like a Python function with a bunch of nested lambdas, might be easier to read if you split it up a bit more. Time to read and understand the code is the most important metric, more important than runtime or memory use. You can optimize for the other two later if you have to, but simple has to prevail for the first pass otherwise it's going to be hard for other people to understand. In fact, it'll be hard for you to understand too when you come back to it 3 months later without any context.
Note that I've cut a few things for simplicity. For example: VHDL doesn't quite require every line to run at the same time, but it's still a major paradigm of the language that isn't present in most other languages.
Ok that was a lot to read. I guess I have more to say about programming than I thought. But the core ideas are: Python is pretty good, other languages don't need to be scary, learn your data structures and algorithms and above all keep your code simple and clean.
#programming#python#software engineering#java#java programming#c++#javascript#haskell#VHDL#hardware programming#embedded programming#month of code#design patterns#common lisp#google#data structures#algorithms#hash table#recursion#array#lists#vectors#vector#list#arrays#object oriented programming#functional programming#iterative programming#callbacks
15 notes
·
View notes
Text
Insert a singly linked list in lexiographic order
P1 <- P2<- pt
while (P1 <> null) and (Ip. info > P1.info) loop
P2<-P1
P1 <- P1. link
End loop
If Pt = P1 then
Ip.link <- Pt
Pt<-IP
Else
P2.link <- Ip
Ip.Link <- Pt
End if
9 notes
·
View notes
Text
youtube
Data Structure and Algorithms in JAVA | Full Course on Data Structure
In this course, we are going to discuss Data Structures and Algorithms using Java Programming. The data structure is a way to store and organize data so that it can be used efficiently. It is a set of concepts that we can use in any programming language to structure the data in the memory. Data structures are widely used in almost every aspect of computer science i.e. operating systems, computer science, compiler design, Artificial Intelligence, graphic,s and many more. Some examples of Data structures that we are going to cover in this course are arrays, linked lists, stack, queue, Binary Tree, Binary Search Tree, Graphs, etc. Apart from knowing these data structures, it's also important to understand the algorithmic analysis of a given code. Different Sorting and searching techniques will be talked about with their implementation in java programming. Lastly, this course contains information on the Greedy approach, Dynamic approach, and divide and Conquer approach to programming.
#youtube#free education#education#educate yourselves#technology#educate yourself#data structures#data analytics#Data Structure and Algorithms in JAVA#javaprogramming#Data Structure and Algorithms#how to think like a programmer#programming classes#programming
3 notes
·
View notes
Text
a struct and a costruct
3 notes
·
View notes
Text


// August 18th, 2024
Yesterday I broke in tears because I think I’m going to fail Data Structures and Algorithms this semester. It’s just, too much. Not the topics I have to study, not even that I don’t actually enjoy practicing it, oh no. It’s that I don’t think I’m going to be able to send all of my assignments on time and they affect the final grade a lot. The materials for all three subjects get released only on Wednesdays at noon and it’s expected that an almost impossible amount of assignments are delivered before the following Tuesday at 8 p.m.
Why can’t I, for instance, do the work, send it as soon as I have it ready, and they can just take into account that I actually followed on the lessons and studied hard for the two exams before the final? It’s just… I don’t know. It seems ridiculous to me. I would be doing my best even without having that stupid pressure of doing the assignments on time, like any other person who is serious about theirs studies. I know I probably sound like a stupid kid, but people going to this university at night usually have a job already, otherwise, why wouldn’t we be taking the lessons on mornings/afternoons? Don’t they think about those cases? Incredible.
And I know one can probably think, “well, why don’t you drop that subject and follow up others and retake this one further in the future?
And the answer to that is, a) I’m feeling old to keep postponing my graduation (35 is becoming my least worst shot) and b) I have more plans, like for other important aspects of my life as well. And… ugh. The pressure. It’s too much.
I spoke to my sweet boyfriend about all these worries I have and he’s happy with both me telling him what I feel and how hard I am working with this. “Honey, it’s just the second week of the semester. You got this”. Cuddles and sushi were provided, and even when that didn’t wash the worries or pressure away, I felt loved. And cared for. I’m so lucky to have him in my life.
Sorry for the rant guys. I get that this is just the way it is and that these are the terms for getting a good grade, and if I don’t like it I can just leave it and try again, but… I just needed to get some of it out of my chest 🥲
But hey, at least I was able to write my very first little programs in C yesterday. Yay 🍷
#studyblr#my post#shelikesrainydays#pressure#being and adult and a student is so freaking hard#personal rant#data structures#algorithms#C
2 notes
·
View notes
Text
Starting today, July 22, 2024, I'm committing to thoroughly learn Data Structures and Algorithms (DSA) with a focus on Java, aiming to complete the course within 100 days while also practicing problems on LeetCode and GeeksforGeeks (GFG).
3 notes
·
View notes
Text
Software Technical Interview Review List
Data Structures
Arrays (and Java List vs ArrayList)
String
Stack
Queue
LinkedList
Algorithms
Sorting (Bubblesort, Mergesort, Quicksort)
Recursion & Backtracking
Linear and Binary Search
String/Array algos
Tree traversal
Dynamic Programming
Graph algos (DFS, BFS, Dijksta's and Kruskals)
OOP fundamentals
Polymorphism
Inheritance
Encapsulation
Data abstraction
SOLID and GRASP
Explanations & example questions:
Strings and Arrays [ 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 ]
Stacks and Queues [ 1 | 2 ]
LinkedList [ 1 | 2 ]
Sorting & searching [ 1 | 2 | 3 | 4 | 5 | 6 | 7 ]
Recursion and Backtracking [ 1 | 2 | 3 | 4 ]
Dynamic Programming [ 1 | 2 | 3 | 4]
Graphs [ 1 | 2 | 3 ]
Tree [ 1 | 2 ]
General DS&A info and questions [ 1 | 2 | 3 | 4 | 5 ]
OOP review & questions [ 1 | 2 | 3 ]
#ive been procrastinating this coding assessment for my interview so bad 😭😭#im just scared of messing up cause i need this internship#But its due soon so im really buckling down now >:)#object oriented programming#algorithms#data structures#software engineering#ref#resource#mypost
15 notes
·
View notes
Text
📢 Mastering DSA in Java? Here are some essential practice questions:
1️⃣ Reverse a String 2️⃣ Implement a Stack 3️⃣ Find the Middle of a Linked List 4️⃣ Check for Balanced Parentheses 5️⃣ Binary Search in a Sorted Array
Follow : Algo2Ace.com
Boost your coding skills today! 🚀 #Java #DSA #CodingInterview #LearnToCode #TechTips
2 notes
·
View notes
Text
im trying so hard to understand linked lists 😭😭😭😭😭😭😭
13 notes
·
View notes
Text
What Dijkstra's two-stack algorithm does to Left parenthesis of Infix expression is everyone does to me
.
.
.
IGNORE 🤡
6 notes
·
View notes
Text
Become Coding Expert with Data Structure Training Institute
Are you looking to take your programming skills to the next level?
Master the foundations of computer science with our comprehensive data structures and algorithms training!
Why Data Structures Matter: Data structures form the backbone of efficient and organized programming. Understanding how to manipulate and utilize data is crucial for solving complex problems and creating optimized algorithms. Whether you're a beginner or an experienced coder, diving deep into data structures is a game-changer for your coding journey.
At Kochiva, the premier Data Structure Training Institute in India, we provide in-depth training on essential data structures like arrays, linked lists, stacks, queues, trees, graphs, and more. Our expert instructors with years of industry experience will help you truly understand how these data structures work and how to implement them efficiently in any programming language. You’ll gain hands-on experience with coding challenges and projects designed to hone your skills.
Don't just learn data structures; become a coding maestro! 🎓 Enroll in Kochiva now and sculpt your coding prowess with the best in the industry.
Your coding adventure awaits! 🚀💻
#kochivamarketing#kochivalearning#online courses#kochivacourses#onlineclasses#data strategy#datascientist#data structures#instituteinindia
6 notes
·
View notes
Text
FIFO is ass
Bruh bro just took a major L going from FILO to this
5 notes
·
View notes