#advent of code 2023
Explore tagged Tumblr posts
Text
I'm using advent of code as an excuse to learn haskell, and made this pattern-matching map, which feels slightly abominable.
extractNumeral :: String -> (Maybe Char, String) extractNumeral ('o':'n':'e':xs) = (Just '1', 'e':xs) extractNumeral ('t':'w':'o':xs) = (Just '2', 'o':xs) extractNumeral ('t':'h':'r':'e':'e':xs) = (Just '3', 'e':xs) extractNumeral ('f':'o':'u':'r':xs) = (Just '4', xs) extractNumeral ('f':'i':'v':'e':xs) = (Just '5', 'e':xs) extractNumeral ('s':'i':'x':xs) = (Just '6', xs) extractNumeral ('s':'e':'v':'e':'n':xs) = (Just '7', 'n':xs) extractNumeral ('e':'i':'g':'h':'t':xs) = (Just '8', 't':xs) extractNumeral ('n':'i':'n':'e':xs) = (Just '9', 'e':xs) extractNumeral (x:xs) = if isNumeral x then (Just x, xs) else (Nothing, xs) extractNumeral [] = (Nothing, [])
I don't know if i'm proud or disappointed by this. This feels like it could be done in a smoother fashion, but then again one gotta do some kind of lookup of spelling for letters anyway.
How should I have done this? Also, is there some way to avoid the 's':'p':'e':'l':'l':'i':'n':'g':[] of letters like this?
22 notes
·
View notes
Text
advent of code 2023 day 1
so for the record i'm being pretty casual about this, i have stuff going on this month + my health is wonky as usual so i may not actually be doing this everyday, might do puzzles late, etc
anyway! i'm still a bit of a novice i think, but i decided i would try out this advent of code thing, seemed fun. i'm using html/javascript since that's what i have any experience in right now.
my solutions below the cut so you aren't spoiled if you want to do it yourself!
i decided to keep my part 1 and part 2 solutions separated, they're on the same document. i have some really basic HTML that looks like this:
and this was my solution for part 1 before words got involved:
for the record i have taught myself basic RegEx multiple times, and every time i forget nearly everything, so most of my time spent on this solution was refreshing myself on a little bit of RegEx. i think this is pretty straightforward - split the input by line, then loop through each line and remove anything that isn't a number, then take the first and last digit in each of the remaining strings, add them all together for the result.
the solution for part 2 on the other hand:
i actually found this somewhat frustrating because the problem itself didn't clearly explain whether something like "eightwothree" in the provided example should be "8 2 3" or "8 3"... my original solution disregarded overlapping words like that. this caused issues because if you had something like... i don't know, threeeightwo, my program would make the resulting two digit number 38 instead of 32, resulting in a wrong sum when adding all of them together.
once i realized the issue i was a bit stumped on how to deal with this and get the overlapping words to be included. i knew that i could manually loop through each position in the string, looking for any of the nine digits in either their letter or numeric form, and when it finds a result, push it to an array of results or whatever, but i really didn't want to do that because it would be unnecessarily bulky in my opinion. and i wanted to keep experimenting with RegEx instead. so after some googling i landed on amending the numPattern and changing
lines[i] = lines[i].match(numPattern);
to
lines[i] = Array.from(lines[i].matchAll(numPattern), (x) => x[1]);
and while it works great, i admittedly don't fully understand it. like, conceptually i get its intention and the end result it spits out, but i wish i understood what was happening under the hood with .matchAll() better. i tried to wrap my brain around it, but i'm too hungry and too sick feeling to properly teach myself right now, so i've decided to drop it and if it's still itching at me later, i'll come back when i feel better to understand it.
anyway, after that, still pretty straightforward - once it's taken out all of the numbers in both numeric and written format, it loops through the results and converts the words to digits, then joins all the digits together, then does the same thing as before with slicing off the first and last digit to make two digit numbers and adding them all together at the end with .reduce().
15 notes
·
View notes
Text
Advent of Code - Days 1 and 2
December is upon us, and this year I thought I'd forgo the chocolate advent calendars in favour of Advent of Code, a coding puzzle website that's been running for a few years. Every day of advent (1st of December to 25th December) you get a coding puzzle, with personalised input data so you can't just look up on the solution online. The problems all revolve around parsing text and doing maths, so you can use whatever programming language you want. There's a global leaderboard that you can race to get on (if you feel like getting up at midnight Eastern Time), or you can just do it casually in the name of learning.
This is the third year I've participated in Advent of Code, and hopefully the first year I manage to finish it. I've decided to solve the puzzles in Rust this year, partly because it's a language I want to get better at, and partly because I really enjoy writing it, but somehow I can always find very good reasons to write the other stuff I'm working on in Python.
My code is on GitHub, and I've written programmer's commentary under the cut.
Day 1
The first day of AoC tends to be fairly straightforward, and so it is this year with a simple find-the-character problem. In each line of the input string, we find the first digit and the last digit and use them to construct a two-digit number, so for instance "se2hui89" becomes "29." We start out by loading the input data, which the Advent of Code website gives you and which I downloaded into a .txt file.
include_str!() is an invaluable macro for Advent of Code players. At compile time, it will read the text file that you specify and load its contents into your program as a string. lines() is another useful function that gives you an iterator for the lines in a string - Advent of Code generally splits up its data line by line.
Since the problem itself was straightforward, I decided to liven things up a bit by creating a multi-threaded program. One thread finds the digit at the beginning of the string, and one finds the string at the end. Luckily, Rust has a lot of tools in the standard library to make concurrency easier.
The particular technique I'm using here is called Multiple Producer Single Consumer, where multiple threads can send data into a channel, and a single thread (in my case, the main one) consumes the data that's being sent into it. I'm using it because I'm storing the numbers I find in a vector, and Rust's ownership system prevents more than one thread from having access to the vector, so instead each thread will send the numbers through a channel, to be processed and added to the vector in the main thread.
The thread itself is very simple - for each line in the text, I iterate through the characters and test whether each one is a digit. Once I find the digit, I multiply it by ten (because it's the first digit of a two-digit number) and send it down the channel. I've also sent the index to keep track of which line I've analysed, since the other thread might be working on a different line and the consumer wouldn't be able to tell the difference. The thread looking for the last digit in the string is almost exactly the same, except it reverses the chars before iterating through them and it doesn't multiply the digit it finds by 10.
Finally, all that remained was to consume the digits and add them into the vector. When you're consuming a channel, you can use a for loop to process values from the channel until the producers stop sending values. And the index variable makes a return here, which we use to keep track of which number each digit is a part of.
The addition is to combine the two digits into a single two-digit number. The vector is filled with 0s when it gets initialised, so each digit that arrives is added to 0 (if it is the first digit to arrive) or to the other digit (if it is the second digit to arrive). For instance, in the string "se29tte7", if 7 arrives first, we get 0 + 7 (7), and then when 2 arrives we add on 20 (since it has been multiplied by 10 already) to get 27, which is the correct answer.
The second task was more complicated, because now I had to be keeping track of all the non-numeric characters to see if they would eventually form the word for a number. I started by writing a function to check if a string contained a number's word.
Originally, this function returned a boolean, but I needed a way to know which number that the word in the string referred to. The easiest way was to store the possible number-words in tuples, associated with the integer version of that number, and then just return that integer if a number-word was found.
The match is also more complicated, because now any character that isn't a digit might be part of a number-word instead. So if the character is not a digit, I added it to the string of non-digit characters I'd found so far and checked if there was a number-word in that string. Once we find something, as before, we multiply it by ten and send it down the channel.
The other thread is similar, but unlike task 1 I couldn't simply reverse the characters, because although I have to search through them right-to-left, the number-words are still written left-to-right. I ended up using the format!() macro to prepend new characters onto the string so that they would be in the correct order.
Day 2
I found Day 2's task more difficult. The trick to it lies in splitting out the different units of data - each game (separated by a line break) has multiple rounds (separated by semicolons), each round has multiple blocks (separated by commas), and each block has a colour and a count, which are separated by spaces. All of this splitting required a lot of nested for loops - three, to be exact.
But I started off by stripping off the beginning of the string which tells you what game you're playing. I realised that, since the games are in order in the input text, you don't actually need to read this value; if you're iterating through the lines of the input like I was, you can just use the index to keep track of the current game. I also made a variable to keep track of whether or not a game is possible (I assume it is until the program proves that it isn't).
Mutable variables like that one make it really easy to shoot yourself in the foot. In an earlier version of the program, I changed that variable to false whenever I found an impossible block in a game. All well and good, but I forgot to prevent the variable from being changed back to true when a possible block was found in that same impossible game. As such, my code only marked games as impossible if the last round were impossible, causing it to wildly over-estimate the number of possible games.
The solution I found to that blunder involved a separate block_possible variable which I used to keep track of whether each block was possible, and then, if the block was impossible then the game would be marked as impossible. Possible blocks do nothing, because they can't account for any other blocks in that same game that might be impossible.
Quick side-note - I've used a lot of panic!() and unwrap() in this solution. Just for the beginners who might not know this: in general, using those functions like this is incredibly bad practice, because they will crash the program if there's anything unexpected in the input. This program's first bug was a crash which happened because there was a single blank line in the input file that shouldn't have been there. I'm only doing this because the program is designed to work with very specific input, so I can make assumptions about certain things when I process the text.
After that little bit of pattern matching, the only thing left is to add the game's ID to the vector which is keeping track of all the possible games. Something I learned at this point is that the index variable I've been using to keep track of the game's ID has a type of usize, which isn't interchangeable with Rust's other integer types like u32 and i32, so I had to convert it explicitly.
And that's that for task 1! It took me a lot of attempts to solve, because I probably should have had lunch first and made a lot of stupid mistakes, including:
Accidentally using count() where I should have used sum() on an iterator.
Badly indenting that if statement in the last image so that I was accidentally adding each ID to the list three or four times per line.
Forgetting to remove some debugging code that threw off the whole result.
But task 2 was comparatively much easier. The main difference was that instead of setting one variable to see if a game was possible, I set three variables to track the minimum number of bricks in each colour needed per game. It worked first try, but I'm not completely happy with it - in particular, I feel like this match statement was a little too verbose:
But all in all I think it went well and I am excited to try my hand at tomorrow's problem.
1 note
·
View note
Text
Unlock Special Tatcha Event: Fukubiki Offers of Minis and Product Favorites | Press - Affiliate
Hey Everyone! I am so excited to announce that I am now officially a Tatcha skincare affiliate – which means I can bring you exclusive discounts and upcoming event information! If you’re new to the world of skincare, let me give you the inside scoop on Tatcha! Tatcha is a much loved, cult level luxury skincare brand from Japan which focuses on the best ingredients and scientific knowledge in…
#2023#2024#Advent Calendar Gift Ideas#advertising on blogs#Amazon#Amazon Discounts#Amazon Prime Day Sale#Amazon Prime Day Sale Best Of#Amazon Prime Early Access Sale#Amazon Prime Early Access Sale Link#Amazon Prime Free Trial Code#Amazon Prime Free Trial Link#Beauty Blog#beauty blogging help#Beauty Discounts#Beauty Discounts 2022#Beauty Influencer#Beauty Review#become a brandbassador#Become an ambassador#becoming an influencer#Best Beauty Calendars#Best Beauty Offers June 2023#Best Discounts on Amazon Prime Sale#Brandbassador#BrandBassador App Review#Brandbassador Appl#Brandbassador legit#Cult Beauty Discount#Cult Beauty Discount Code
0 notes
Text
It's the home stretch for supporting Science Education
Oh hi. It's me again, the squid biologist on a mission to make it easier for people to connect with science. I run a small nonprofit called Skype a Scientist! We match scientists with classrooms, scout troops, robotics clubs, libraries, and more for virtual Q&As about science. We serve 4000-5000 classrooms every year! We offer this FOR FREE. We also run the tumblr-coded Squid Facts Hotline.
To support our program in 2025, we're selling FROG FACTS advent calendars. Every day you can scratch-off the sparkles to reveal facts about frogs! Please buy one! They're cute and fun and help me give science education away for free.
I unfortunately haven't gotten any grants to support our program for 2025, BUT!! these advent calendars are helping us keep the lights on! If you already bought one, THANK YOU! If you have shared a post about these calendars, that has been INCREDIBLY helpful. If you can't help us financially, reposting is so so helpful!
If you want to support our program, you can donate directly here. We're a 501.c.3 so if you live in the US, donations are tax deductible!
You can also read about our work in detail in our 2023 annual report here!
Thank you all for your support 💕🦑💕
2K notes
·
View notes
Text










🎄💾🗓️ Day 9: Retrocomputing Advent Calendar - The Apple Lisa 🎄💾🗓️
The Apple Lisa, introduced on January 19, 1983, was a pioneering personal computer notable for its graphical user interface (GUI) and mouse input, a big departure from text-based command-line interfaces. Featured a Motorola 68000 CPU running at 5 MHz, 1 MB of RAM (expandable to 2 MB), and a 12-inch monochrome display with a resolution of 720×364 pixels. The system initially included dual 5.25-inch "Twiggy" floppy drives, later replaced by a single 3.5-inch Sony floppy drive in the Lisa 2 model. An optional 5 or 10 MB external ProFile hard drive provided more storage.
The Lisa's price of $9,995 (equivalent to approximately $30,600 in 2023) and performance issues held back its commercial success; sales were estimated at about 10,000 units.
It introduced advanced concepts such as memory protection and a document-oriented workflow, which influenced future Apple products and personal computing.
The Lisa's legacy had a huge impact on Apple computers, specifically the Macintosh line, which adopted and refined many of its features. While the Lisa was not exactly a commercial success, its contributions to the evolution of user-friendly computing interfaces are widely recognized in computing history.
These screen pictures come from Adafruit fan Philip " It still boots up from the Twiggy hard drive and runs. It also has a complete Pascal Development System." …"mine is a Lisa 2 with the 3.5” floppy and the 5 MB hard disk. In addition all of the unsold Lisa machines reached an ignominious end."
What end was that? From the Verge -
In September 1989, according to a news article, Apple buried about 2,700 unsold Lisa computers in Logan, Utah at a very closely guarded garbage dump. The Lisa was released in 1983, and it was Apple’s first stab at a truly modern, graphically driven computer: it had a mouse, windows, icons, menus, and other things we’ve all come to expect from “user-friendly” desktops. It had those features a full year before the release of the Macintosh.
Article, and video…
youtube
Check out the Apple Lisa page on Wikipedia
, the Computer History's article -
and the National Museum of American History – Behring center -
Have first computer memories? Post’em up in the comments, or post yours on socialz’ and tag them #firstcomputer #retrocomputing – See you back here tomorrow!
#applelisa#retrocomputing#firstcomputer#applehistory#computinghistory#vintagecomputers#macintosh#1980scomputers#applecomputer#gui#vintagehardware#personalcomputers#motorola68000#technostalgia#twiggydrive#floppydisk#graphicalinterface#applefans#computinginnovation#historictech#computerlegacy#techthrowback#techhistory#memoryprotection#profiledrive#userinterface#firstmac#computermilestone#techmemories#1983tech
113 notes
·
View notes
Text
⋆ Softpascalitos Masterlist ⋆
This is a regularly updated masterlist of all my works, posted on tumblr + ao3! They're sorted by character with warnings/tags, please still read the full descriptions before reading! My entire blog is 18+ / MDNI.
⭐ - my personal favorites ⛓️ - smut 🍂 - hurt/comfort 💌 - fluff
i write for: javier peña, pedro pascal, agent ortega, joel miller, oberyn martell, marcus acacius, dieter bravo, silva, frankie morales, din djarin, agent whiskey, javi g, tim rockford, marcus acacius, Renaldo (SNL Skit)
last update: 10th of march 2025 If you have requests/questions/feedback or just want to say hi, feel free to pop into my asks! ✮
I do not give permission to have my work copied, translated, rewritten, put into any AI programs (including CAI!), or reposted without my direct agreement.
✮⋆˙ Main Fics ˙⋆✮
To Dig a Grave - Tumblr / AO3 ⭐🍂⛓️ [29k+]
Summary: Twenty-one years after the outbreak, you come to Wyoming looking for something and end up in Jackson after a stranger saves your life. But he doesn't stay a stranger. Turns out Joel Miller is looking for something too. It feels like a fresh start. But when bad luck seems to follow you, Joel is the only one to turn to, forcing both of you to confront your feelings about your pasts- and each other. Tags: Hurt/Comfort, Grief/Mourning, Angst, Friends to Lovers, Slow Burn, Age Difference, Smut, More Tags to be added
Dulcissima - Tumblr / AO3 ⭐🍂⛓️ [55k+]
Summary: General Acacius finds himself entranced by a highly valued priestess of Rome – A Vestal Virgin. But you both have taken vows that make sure your paths may never cross. Until they do. Aka a fix-it fanfic where Acacius survives the Colosseum. Tags: Hurt/Comfort, Smut, Slow Burn, Ancient Rome, Age Difference
We got your back - Tumblr / AO3 ⭐🍂 [6k+]
Summary: You work as a new DEA agent alongside Peña and Murphy. A not-so-kind colleague reveals more about you than you would like. Peña takes you under his wing. (Currently being reworked) Tags: Hurt/Comfort, Slow Burn
Specials Kinktober 2023 31 pieces of (mostly smut) - read on AO3 / Tumblr Advent Calendar 2023 25 Pieces including moodboards, hcs and fics - read on AO3 / Tumblr
✮⋆˙ Javier Peña ˙⋆✮
Pregnancy Sex with Javier Peña - Tumblr / AO3 ⭐⛓️ [2.6k]
Summary: You're pregnant, Javier is overprotective. The problem: You're also really fucking horny. Tags: Smut, Explicit, Aftercare, Pregnancy, Established Relationship, PWP
Beyond Saving - Tumblr / AO3 ⭐🍂 [1.3k]
Summary: Javier is on office duty when he learns that someone close to you has passed, causing both of you to spiral. Tags: ❗ Dead Dove: Do not Eat ❗, Mention of Suicide, Grief, Hurt/Comfort, Angst, Minor Character Death, Trauma, Mental Breakdowns
Kinktober - Hate Sex - Tumblr / AO3 ⛓️ [2.3k]
Summary: When a raid goes wrong, Javier Peña gets pissed. You expect him to take it out on you. You dont expect him to fuck you so good. Aka a steamy office romance with a side of hate sex. Tags: Smut, Explicit, Hate Sex, Unsafe Sex, Rough Sex, Spanking
Kinktober - Humiliation - Tumblr / AO3 ⛓️ [2.2k]
Summary: Javier takes you home after a night out. You worry about waking Steve who lives upstairs- until Javier notices the way you are staring at his gun. (Thats not code for his dick, Im talking about his actual gun). Aka Agent Peña fucks you on his couch using something other than his dick. Tags: Smut, Explicit, Gun Kink, Rough Sex, Praise Kink, Spanking, Dirty Talk
Kinktober - Tying a tie - Tumblr / AO3 🍂 [1.6k]
Summary: Javier resigns from the DEA. You both reflect on your life in Colombia while you help him get ready. You also discuss what is about to follow. Tags: Hurt/Comfort, Trauma, Anxiety, Established Relationship, Crying, Sad Javier Peña
Peluda - Tumblr / AO3 ⭐💌 [1.7k]
Summary: A snowstorm hits Bogotá and you bring back a surprise visitor. Javi is not amused. But, it leads to a realization about himself- and about you. Tags: Fluff, Nicknames, Soft Javi, Snow, Established Relationship
Nochevieja - Tumblr / AO3 ⭐⛓️ [3k]
Summary: Javi's plan for New Years Eve was just him, a glass of whiskey and the stack of files on his desk. Turns out yours involve a red dress, champagne and fucking in the office. Tags: Smut, Semi-Public Sex, Vaginal Sex, Oral Sex, Drunk Sex, New Years
✮⋆˙ Marcus Acacius ˙⋆✮
Healing Hands - Tumblr / AO3 ⭐⛓️ [2k]
Summary: Acacius returns home with an injury—and you try to care for him. But his ideas of healing (and baths) are a little ... different. Especially when you finally have some time to yourselves. Tags: Explicit, Smut, Hurt/Comfort, Fingering, Creampie, Bathing
✮⋆˙ Joel Miller ˙⋆✮
Kinktober - Pegging - Tumblr / AO3 ⭐⛓️ [3k]
Summary: You and Joel run into a sex store on patrol. They have everything one needs to give their older, grumpy partner a good pregging. Aka Joel Miller gets his ass fucked for the first time in his life.Tags: Explicit, Smut, Pegging, First Time, Aftercare
Baby, I'm your National Anthem - Tumblr / AO3 ⭐⛓️ [3k]
Summary: You are back from college for the summer and your family happens to throw the annual Fourth of July Barbecue for your street. Your next-door neighbor and dad's best friend Joel Miller is invited—and you decide to wear a bold outfit. It definitely catches his attention. Tags: Smut, DBF!Joel, Age Difference, Semi-Public Sex, Dirty Talk
Kinktober - Nonsexual Ageplay - Tumblr / AO3 🍂💌 [2k]
Summary: When Joel brings back a book on trauma from patrol, something catches your eye. Having had too much of your childhood taken away by the outbreak, you find a way to get some of it back. Aka a soft Joel Miller making his partner feel safe enough to try nonsexual ageplay. Tags: SFW, Past Trauma, Age Difference, Nonsexual Ageplay
Kinktober - Wax Play - Tumblr / AO3 ⛓️🍂 [1.9k]
Summary: Joel and you are paired up for patrol. There are a lot of things unsaid, a snowstorm rolling in and some candles. Go figure (or go read i guess).Tags: Smut, Explicit, Hurt/Comfort, Snowed in
Kinktober - Somnophilia - Tumblr / AO3 ⛓️ [2k]
Summary: In 2003, Joel Miller is busy with dishes, paperwork and raising a child, leaving little time for his relationship. When he gets restless and sneaks over to her house, finding his girl asleep, he remembers a conversation they had about consent. Tags: Smut, Explicit, Explicit Consent, Age Difference, Established Relationship, Secret Relationship, Aftercare, Crempie, Pre-Outbreak
Kinktober - Playing with hair - Tumblr / AO3 ⭐🍂💌 [1k]
Summary: Joel works in construction in Jackson. During his lunch breaks, he always comes home to you. When you notice a talent of his you hadn't known about, he opens up about the past. Tags: Jackson Era, Established Relationship, Bathing/Washing, Domestic Fluff, Hurt/Comfort, Joel play guitar, Good Parent Joel
Kinktober - Massaging - Tumblr / AO3 🍂 [2.2k]
Summary: When Joel comes home after a long day of work, you crave nothing more than him. Until you're both reminded of his age. Tags: Hurt/Comfort, Established Relationship, Age difference, Massage, Kissing, Healthy relationships
Kinktober - Familiar Scents - Tumblr / AO3 ⛓️ [2.4k]
Summary: Over the span of many years, Joel Millers scent always stays the same. It starts when he takes you for a hike before the Outbreak- and continues for long after. Tags: Friends to lovers, Age difference, Eventual Romance, Eventual Smut, Fingering, P in V Sex
Kinktober - Tooth Brushing - Tumblr / AO3 ⭐🍂 [2.4k]
Summary: Grief is cruel and just because you and Joel live in the safe haven that is the Jackson community it does not mean you're immune to it. Possibly the saddest (but also kinda best) thing I have written so far. Tags: Hurt/Comfort, Angst, Character Death, Established Relationship, Grief/Mourning, Protective Joel, Survivors Guilt
Kinktober - Crossdressing - Tumblr / AO3 ⛓️💌 [2k]
Summary: Joel Miller has been wanting to try a different piece of clothing for a long time. It's not until you that he feels supported enough to do so. Turns out, you both really fucking like it.Tags: Smut, Fluff, Insecurity, Soft Joel, Slight Mommy Kink, Jackson Era, Sub Joel Miller
Kinktober - (Public) Help with button - Tumblr / AO3 ⛓️ [2.4k]
Summary: What happens in a dimly lit corner on the lap of Joel Miller at the town dance, stays at the town dance. Almost. Tags: Smut, Explicit, Dirty Talk, Vaginal Fingering, Public Sex, Teasing, Established Relationship, Age difference, Jackson Era
Kinktober - Daddy - Tumblr / AO3 ⛓️🍂 [2.6k]
Summary: Joel has been noticing a few things about your relationship that make him wonder about your feelings regarding your father. As you open up about your issues, he's sweet, supportive and makes you feel better in the way only he can. Tags: 2003!Joel Miller, Pre-Outbreak, Smut, Explicit, Hurt/Comfort, Daddy Kink, Daddy Issues, Crying, Fingering, Cock Warming, P in V Sex, Secret Relationship
Kinktober - Free Use - Tumblr / AO3 ⛓️ [1.9k]
Summary: Joel comes home urgently needing some relief. Its a good thing youre there- and the first time Joel makes use of an ... interesting agreement. Tags: Smut, Explicit, Free Use, Rough Sex, Light Dom/Sub, Dirty Talk, Angry Sex, Shower Sex, Baking, Female Reader, Established relationship
Snowy Surprise - Tumblr / AO3 ⛓️💌 [2.2k]
Summary: Joel takes advantage of your lunch break on patrol for ... other activities. Afterwards, a promise he made about christmas decorations comes back to haunt him. Tags: Smut, Explicit, Fluff, Soft Joel, Established Relationship, Fingering, Dirty Talk, Semi-Public Sex
Here cums Santa Claus - Tumblr / AO3 ⛓️💌 [1.8k]
Summary: Jackson needs a Santa Claus - and Joel is the perfect fit. Getting to have you on his lap is just a bonus. Aka the one where Joel is dressed up as Santa Claus and you get to ride him. Tags: Smut, Explicit, Fluff, Soft Joel, Established Relationship, Fingering, Dirty Talk, P in V Sex, Costume Kink, Riding, Creampie
✮⋆˙ Silva ˙⋆✮
Kinktober - Armpit + Orgasm Denial - Tumblr / AO3 ⛓️ [1.6k]
Summary: When Silva comes home after a long day on the ranch, he smells amazing. He also distracts you from cooking in a quite special way. Tags: Explicit, Smut, Sweat, Praise Kink, Established Relationship, Oral Sex, P in V Sex
Kinktober - Dancing together - Tumblr / AO3 ⭐🍂 [1.4k]
Summary: When a particularly hard rain hits your little ranch, so does the sadness. Luckily Silva is there to make it better.(This fills a few gaps in Silvas life in a way that ties in with the movie.) Tags: Established Relationship, Domestic, Angst, Hurt/Comfort, Parent Silva, Romance, Kissing in the rain, Slow dancing
✮⋆˙ Oberyn Martell ˙⋆✮
Kinktober - Collaring - Tumblr / AO3 ⛓️🍂 [2k]
Summary: Oberyn gets you a special present, one that both of you will enjoy. But things dont always go as planned. Tags: Explicit, Smut, Established Relationship, Safeword Use, Hurt/Comfort
Kinktober - Breeding - Tumblr / AO3 ⭐⛓️🍂 [2k]
Summary: Oberyn and her have been trying for a baby to no avail. Ever the loving viper, he comes up with an idea. Tags: Explicit, Smut, Established Relationship, Emotional Hurt/Comfort, Breeding, Dirty Talk, Praise Kink
Kinktober - Threesome - Tumblr / AO3 ⛓️ [4.5k]
Summary: You are in charge of serving wine to the dornish folk at the kings wedding. A couple catches your eye and it may not be as one-sided as you thought at first. Aka the steaming hot threesome with Oberyn and Ellaria we all need. Tags: Smut, Explicit, Creampie, Threesome (FFM), Servant Reader, Aftercare, Porn with Plot
Kinktober - Washing hair - Tumblr / AO3 🍂 [1.7k]
Summary: A few weeks after you and Oberyn begin to try conceiving and days before he leaves for Kings Landing, he finds you cooling down in the baths during a hot day. Tags: Hurt/Comfort, Angst, Mild Smut, Bathing/Washing, Pregnancy, Established Relationship
Kinktober - Pregnancy - Tumblr / AO3 ⛓️🍂 [1.4k]
Summary: Oberyn has been more cautious around her now that the due date is near. He has to realize it's not what she wants. And who can deny the wishes of a pregnant woman? Tags: Smut, Pregnancy Sex, P in V Sex, Oral Sex, Romance, Fluff, Domestic, Established Relationship, Female Reader
✮⋆˙ Jack Daniels ˙⋆✮
Kinktober Day Eighteen - Spanking + Whipping - Tumblr / AO3 ⭐⛓️ [2.2k]
Summary: When you mess up during a mission, Jack doesn't want to have to report his own girlfriend. Since he is your higher-up, you work out an agreement- a punishment by Whiskey himself. Tags: Smut, Explicit, Spanking, Whipping, Dirty Talk, Praise Kink, Crying, Established Relationship, Rough Sex, Dom/Sub Undertones
Kinktober Day Twentythree - Deepthroating + Facesitting - Tumblr / AO3 ⛓️ [1.9k]
Summary: You've never deepthroated anyone in your life- but you're eager to make your man feel as good as possible. You receive a proper thank you as well. Tags: Smut, Explicit, Dirty Talk, Praise Kink, Deepthroating, Facesitting, Rough Sex, Oral Sex, Aftercare, Established Relationship
✮⋆˙ Pedro Pascal ˙⋆✮
Here with me - Tumblr / AO3 🍂💌 [1.2k]
Summary: During his time in Morrocco, Pedro finds himself in need of reassurance. You are happy to help. Tags: Emotional Hurt/Comfort, Established Relationship, Insecurities, Age Difference
I'll look after you - Tumblr / AO3 ⭐🍂💌 [2k]
Summary: Pedro is sick (but of course he doesn't admit it). You look after him. Hurt/Comfort (but the twist is that you're the one doing the comforting). Tags: Hurt/Comfort, Sickfic, Emotional, Established Relationship
✮⋆˙ Agent Ortega ˙⋆✮
Agents don't have favorites - Tumblr / AO3 ⛓️🍂 [2.6k]
Summary: Agent Ortega visits the Emerald Palace, but finds the woman tending to the horses more interesting than those tending to the men. After he leaves for a while, he comes back to an unwanted surprise. Aka its emotional but also they fuck.Tags: Explicit, Referenced Non-Con Elements, Hurt/Comfort, Smut
Kinktober - Shoe Shining + NTR (Cheating) - Tumblr / AO3 ⭐⛓️🍂 [2.2k]
Summary: Ortega returns to Brimstone. When he gets a shoe shine from a past flame, who is now married, things get complicated. Tags: Explicit, Hurt/Comfort, Smut, Cheating, Rough Sex, Spanking, Aftercare, Creampie, Dirty Talk
✮⋆˙ Dieter Bravo ˙⋆✮
Kinktober - Titfucking - Tumblr / AO3 ⛓️🍂 [1.7k]
Summary: Dieter is alone in quarantine and begs you to come join him. Even with a few obstacles, you treat him the way he deserves. Tags: Explicit, Smut, Titfucking, Emotional Hurt/Comfort
Kinktober - Watersports - Tumblr / AO3 ⛓️ [1.7k]
Summary: Dieter is on edge because of an upcoming premiere and as his personal assistant, you try to keep him calm as well as sober. There is one thing that may help. Tags: Smut, Explicit, Established Relationship, Semi-Public, Assistant Reader, Alcohol, Watersports, Dom/Sub Undertones
✮⋆˙ Frankie Morales ˙⋆✮
Kinktober Day Six - Frottage - Tumblr / AO3 ⛓️ [1.4k]
Summary: After a flying lesson, you find yourself drawn to the man in the pilot seat. Luckily for you, Frankie knows exactly what you need. Tags: Smut, Explicit, Dirty Talk, Established Relationship
✮⋆˙ Din Djarin ˙⋆✮
Kinktober Day Nine - Gloryhole - Tumblr / AO3 ⛓️ [1.6k]
Summary: Din just wants some quick pleasure. You just want to enjoy your job for once. Both of you get more than you bargained for. Tags: Smut, Explicit, Prostitution, Oral Sex, Strangers, Semi-Public
✮⋆˙ Javi Gutierrez ˙⋆✮
Kinktober Day Twentyone - Lingerie - Tumblr / AO3 ⛓️💌 [2.1k]
Summary: Javi usually gets the movie memorabilia he loves so much for his birthday. This year, he gets something infinitely better. Tags: Smut, Explicit, Established Relationship, Birthday Sex, Lingerie, Kissing, Fluff, Praise Kink, Dirty Talk, Vaginal Fingering, P in V Sex
✮⋆˙ Tim Rockford ˙⋆✮
Kinktober Day Twentynine - Breathplay - Tumblr / AO3 ⛓️ [2.1k]
Summary: After a successful case, everyone goes out to celebrate. Everyone except your boss, Tim Rockford. But, with an empty office to make use of, you both find your own way to celebrate. Tags: Smut, Explicit, Established Relationship, Choking, Coworker Reader, Female Reader, Office Sex, Semi-Public Sex, Secret Relationship
#pedro pascal x you#pedro pascal x reader#pedro pascal x y/n#pedro pascal fanfiction#javier peña x reader#javier pena fic#masterlist#softpascalito#joel miller x reader#joel miller / reader#javier pena fanfiction#din djarin / you#din djarin x reader#din djarin / reader#din djarin#fanfic#fanfiction#ao3 fanfic#ao3 author#tim rockford / you#tim rockford x you#silva / you#silva / reader#javi g / you#javi gutierrez#javi gutierrez / reader#agent whiskey / you#agent whiskey x you#frankie morales / you#frankie morales / reader
304 notes
·
View notes
Text

Vending Machines: From Pokémon Collaborations to Disaster Preparedness
Location: Gumyoji, Minami Ward, Yokohama, Japan Timestamp: 17:45・2024/04/09
Fujifilm X100V with 5% diffusion filter ISO 400 for 1/250 sec. at ƒ/2 Classic Negative film simulation
Japan boasts an impressive array of vending machines, numbering around 2.6 million as of December 2023. In the span of the last four decades, I've witnessed significant shifts in this vending landscape. Notably, there has been a decline in machines vending alcohol and cigarettes, paralleled by a rise in machines offering both hot and cold beverages from a single unit. Among these innovations, my personal favorite is the advent of machines equipped with QR code scanning capabilities, allowing seamless payment through an app on my smartphone.
In my photo, two distinct elements immediately captured my eye: the presence of a Pokémon character adorning the vending machine, and upon closer inspection, the machine's disaster response capabilities.
The Ito En beverage company has partnered with the popular Pokémon Go game for a joint promotion of the game and Ito En drinks. Beyond its promotional features, this vending machine also serves a crucial role in the local community as a disaster response vending machine. In the event of a severe earthquake and subsequent power outage, it can dispense drinks at no cost, providing essential relief to those affected by the crisis.
While I sincerely hope to never have to utilize the services of a disaster response vending machine, it's reassuring to know that some large corporations are stepping up to make their services and products accessible to society during times of crisis.
Check out my full write-up (a concise 2-minute read), which includes a glossary and references for further reading (https://www.pix4japan.com/blog/20240409-vending).
#ストリートスナップ#横浜#弘明寺商店街#災害救援自販機#pix4japan#FujifilmX100V#streetphotography#Japan#Yokohama#vendingmachine
28 notes
·
View notes
Text
Advent of Code 2023 Reminder
Thursday 30th November 2023
Just a reminder that Advent of Code 2023 starts tomorrow! (Totally didn't have a reminder set for a whole year because I forgot to try it last year...)
I personally don't know if I'll be able to complete all challenges (or even remember to do it in the first place) but trying is better than doing nothing at all~!
I look forward to it, haven't done it before~!
Link: Advent of 2023 💻
⤷ ♡ my shop ○ my mini website ○ pinned ○ navigation ♡
#xc: side note post#codeblr#coding#progblr#programming#studyblr#studying#computer science#tech#comp sci
70 notes
·
View notes
Text
OK, who will be up for this idea? CODEBLR CODING ADVENT CALLENDAR! ( "BEST IDEA EVER!" - Everyone) So basically, Advent of Code is a online competition of solving a daily programming puzzle the best. As they write on their website "please don't use AI to get on the global leaderboard.". So anyone who wants to be an asshole, can just use AI and get on the leaderboard. Which basically means the leaderboards are worthless. BUT! It is still fun to do. It is just more fun together with people SO I HAD AN IDEA! How about we on the codeblr discord makes a channel to facilitate this? Where each of us uploads github repository links and talk about our solutions and how we are thinking about the problem and how our code works? I think that would be SUPER fun (I would not be able to participate every day. But on the days I have time I will definitely join in!!!) (I was inspired by xiacodes post: )
Link to the competition website:
29 notes
·
View notes
Text
✨2023: A Summary✨
Post your most popular and/or favourite edit/gifset/analysis for each month (it’s okay to skip months!)
Tagged by @daymork - Thanks for wanting to see how my analyses 'popped off and what my personal favs are!' 💖
January
most popular — Bad Buddy/My School President parallel - not an analysis but people do love a good parallel!
favourite(s) — Never Let Me Go discussion - I love when something I write inspires others and it sets off a long thread where people keep adding to it. | Another Bad Buddy/My School President parallel - not technically my post but I love the additions I brought together on this.
February
most popular/favourite(s) — The colour-coding of Bed Friend - another long thread where others joined in.
March
most popular/favourite(s) — A ramble about Moonlight Chicken - which includes a little analysis of the show as it ended.
April
most popular — "What exactly does being blue/red/yellow/etc. coded mean?" - I don't get asks often but I was humbled that someone came to me to understand what colour-coding meant.
favourite(s) — Chains of Heart colours series - I was late to the series, and it ended disappointingly, but the colours were brilliant. | Colour-coded folks in UMG - not a bl but they also colour-coded the leads.
May - n/a - I didn't really write any big posts/analysis this month.
June
most popular — "It's the right moment to kiss!" Our Skyy 2 x BBS x ATOTS - Aof calling himself out as well as us bl fans.
favourite(s) — Colours in Our Skyy 2 BBS x ATOTS - I couldn't resist writing about it since it stayed consistent to how they used colours in the original series.
July
most popular — The casting of Be My Favourite - the realisation that the 'odd pairing' of Krist and Gawin was integral to the story being told.
favourite(s) — Be My Favourite ep 9 - the ep that got me thinking about the characters even more. | Kawi and his sense of Self - where I dip my toe into philosophy, theories of The Self, and how memory plays a part in that.
August
most popular/favourite(s) — Laws of Attraction ep 5 - I loved everything about this series but this was the one that got the most attention - maybe because it featured Silvy a lot? 😂
September
most popular — Friends don't let friends go - which pairs with Only friends stay (posted in Oct), and which say a lot about the group and Boston.
favourite(s) — Clowned correctly Laws of Attraction - I just love when something flippant I say is (sort of) right in the end!
October
most popular — The Rainbow Best sweater - not an analysis, nor any created art...but the sweater itself is art, as is that so many characters and actors have worn it.
favourite(s) — Getting emotional over Kiseki: Dear to Me - this was the ep that hit me in the feels. | The Thai Communal Wardrobe items #6 and #3 - again not analysis or art but the start of the communal wardrobe list!
November
most popular — Mhok's Fart Proudly t-shirt - this had me going back to my Bad Buddy days of analysing t-shirts.
favourite(s) — Horney Hockey part 1 and part 2 - more t-shirts, more fantastic additions. | A thought on Night and Day - I'm still so curious about Day and Night's relationship and I can't wait to find out what their deal is. | Band aids on Rung's car and the goldfish slippers the symbolism of Mhok and day's 'damage' and the healing that they're doing. | "It is only with the heart that one can see rightly" | The t-shirts are definitely t-shirting - these last two are essentially reflecting on the same thing - the sentiments in The Little Prince story that Mhok reads out in ep 1 of Last Twilight.
December
most popular — Mhok is Day's eyes - a brilliant use of colour in Last Twilight.
favourite(s) — Couple bracelets - just me excited over possibilities. | Most memorable items of clothing in bl series in 2023 - looking back through the year. | The Thai Communal Wardrobe bl advent - this was a fun series to do...and it only encompassed about a third of the items on the list, so I'll be posting them in dribs and drabs throughout the year.
--
Tagging people who also tend to write analysis or meta posts, I'd love to see which were a hit with the masses and also which were your favourites: @wen-kexing-apologist @grapejuicegay @lurkingshan @btwinlines @twig-tea @rocketturtle4 @waitmyturtles @telomeke and @respectthepetty
26 notes
·
View notes
Note
i dont see why a stamp as a premium perk is so bad, theyve already had stamps as merch code exclusives (merch that is way more expensive and hard to get than a single month of premium, i might add). and besides, a huge chunk of stamps are already unobtainable, or so expensive they may as well be unobtainable, for the vast majority of players. so i fail to see how this makes any difference to the hobby of stamp collecting on neo. i dont have premium so i will be missing out on this one, but i love collecting stamps. its one of my main hobbies on the site, but i just think people are hugely overreacting to this when its not really anything new. no one will ever have every stamp anyways, theres no point in being so upset over another really expensive one.
the difference between rare item codes and the advent calendar is that historically the AC has been an "equalizer". it's always been available to all accounts (with the exception of side accounts given the NP prize), and the prizes are given equally regardless. that's why rereleases of items like the candychan stamp in 2023 were so monumental, because it allows for EVERYONE to enjoy something nice for the holidays.
tnt turning this not only into a premium/non premium player divide, but dangling the item in front of players' faces to try and entice them to purchase premium (since you can't receive past items if you sign up for premium after the day they're given) is the issue, not just having premium exclusive stamps or bonuses.
they knew that this would be an issue of premium players having an edge over non premium players too, because they specified that there wouldn't be any premium exclusive battledome items. if it was equivalent to item codes then they wouldn't have bothered.
#ask box#txt#it's artificial scarcity that benefits premium players specifically during a site event that has always been the great equalizer#and i hate that they try to get people to sign up by showing the premium exclusive item like a carrot on a stick#c'mon guys there's less scummy ways to keep the lights on than fomo#also i used the word premium and not paying because i'm literally a paying player and i've gotten zero bonuses for “helping site costs”
5 notes
·
View notes
Text
RHXO Christmas Gift Guide 2023! (PR - Affiliated & Gifted)
Stuck on what to get for someone? Or what to put on your wish lists? Here is my Ultimate 2023 Gift Guide! Discover my curated guide on what would be great gifts to give and receive this year - all neatly sorted into one place!
Stuck on what to get for someone? Or what to put on your wish lists?Here is my Ultimate 2023 Gift Guide! Discover my curated guide on what would be great gifts to give and receive this year – all neatly sorted into beauty, makeup, skincare, haircare and lifestyle for you to uncover your favourite! I’ve also sourced the cutest gift wrap, novelty-but-useful-gifts and stocking fillers to meet all…
View On WordPress
#2023#2024#2025#Advent Calendar Gift Ideas#advertising on blogs#Amazon#Amazon Discounts#Amazon Prime Day Sale#Amazon Prime Day Sale Best Of#Amazon Prime Early Access Sale#Amazon Prime Early Access Sale Link#Amazon Prime Free Trial Code#Amazon Prime Free Trial Link#Aurelia London - 12 Day Advent Calendar#Beauty#Beauty Advent Calendars#Beauty Bay Discount Code#Beauty Bay Discount Code 2023#Beauty Bay Discount Codes#Beauty Bay Discount Codes 2017#Beauty Bay Discount Codes 2022#Beauty Bay Discount Codes 2023#Beauty Blog#beauty blogging help#Beauty Discounts#Beauty Discounts 2022#Beauty Discounts 2023#Beauty Influencer#Beauty Review#Beauty Works - 12 Day Luxury Advent Calendar.
0 notes
Text
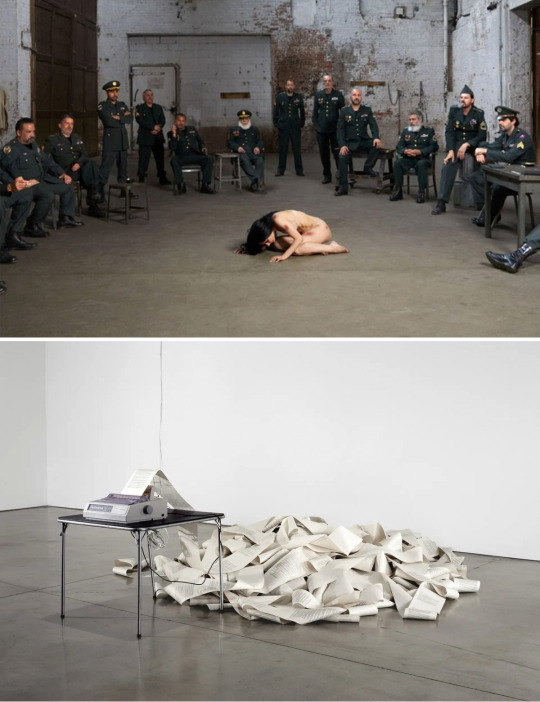
Top, Shirin Neshat, The Fury, 2022, Two-channel video installation, HD video monochrome. Exhibition at Gladstone Gallery, January 26 - March 4, 2023. Via. Bottom, Hans Haacke, News, 1969/2008, RSS newsfeed, paper, and printer. Part of the exhibition Coded: Art Enters the Computer Age, 1952–1982 at LACMA, February 12 - July 2, 2023. Via.
For the 'Prospect 69' exhibition in the Kunsthalle, Düsseldorf, Haacke drew up a concept he elucidated as follows: 'A telex machine installed in the Düsseldorf Kunsthalle prints all the news communicated by the German press agency DPA. The printouts will be put on display for further reading one day after being communicated, and on the third day the rolls of paper will be labelled and dated, then stored in plexiglass containers.' Via.
--
Baudrillard, by contrast, suggests we are no longer immersed in the society of spectacle that is governed by images shaping reality but in the ‘contagion of the virtual’. I would argue that images can no longer be explained or apprehended using the mirror formula to offer a clear distinction between reality and phantasy. Their overwhelming presence in mass media, virtual reality and social networks shapes identities and the perception of the self. However, individuals are not merely passive consumers of these images. They have also become active spectators, interacting with images and using them to perform their identity.
Basia Sliwinska, from The Female Body in the Looking-Glass: Contemporary Art, Aesthetics and Genderland, 2016. Via.
--
Around this magical time of the year, Brad Troemel used to post an Instagram advent calendar of his most popular memes. He doesn’t do that any longer but does regularly post carousels of found stories that are a brilliant portrait of our present and a rich resource. I also enjoyed, and was moved drunkenly to tears one night by, the closing montage of his “Cloutbombing” report, made by Jak Ritger, perhaps because I appear in it myself. I also enjoyed Crisis Acting’s carousels of found videos, which form a more uplifting image of humanity and the world – it’s amazing that they’re able to find and put these out every day – and their longer edits with music too; I like this one ascendant, completely illuminated cut of Hyperballad, made I don’t know by whom; and another cut of an old German comedian lying in a shallow stream while Aphex Twin plays, which I’ll probably never find again; and this video of Chinese musicians, found via Sean Tatol; and these guys that play ambience on the street across Lower Manhattan; and this clip from Connor Clarke, with Ice Spice.
Dean Kissick, 👍 Video carousels, for Spike Tops and Flops 2023.
8 notes
·
View notes
Text
How Large Language Models (LLMs) are Transforming Data Cleaning in 2024
Data is the new oil, and just like crude oil, it needs refining before it can be utilized effectively. Data cleaning, a crucial part of data preprocessing, is one of the most time-consuming and tedious tasks in data analytics. With the advent of Artificial Intelligence, particularly Large Language Models (LLMs), the landscape of data cleaning has started to shift dramatically. This blog delves into how LLMs are revolutionizing data cleaning in 2024 and what this means for businesses and data scientists.
The Growing Importance of Data Cleaning
Data cleaning involves identifying and rectifying errors, missing values, outliers, duplicates, and inconsistencies within datasets to ensure that data is accurate and usable. This step can take up to 80% of a data scientist's time. Inaccurate data can lead to flawed analysis, costing businesses both time and money. Hence, automating the data cleaning process without compromising data quality is essential. This is where LLMs come into play.
What are Large Language Models (LLMs)?
LLMs, like OpenAI's GPT-4 and Google's BERT, are deep learning models that have been trained on vast amounts of text data. These models are capable of understanding and generating human-like text, answering complex queries, and even writing code. With millions (sometimes billions) of parameters, LLMs can capture context, semantics, and nuances from data, making them ideal candidates for tasks beyond text generation—such as data cleaning.
To see how LLMs are also transforming other domains, like Business Intelligence (BI) and Analytics, check out our blog How LLMs are Transforming Business Intelligence (BI) and Analytics.

Traditional Data Cleaning Methods vs. LLM-Driven Approaches
Traditionally, data cleaning has relied heavily on rule-based systems and manual intervention. Common methods include:
Handling missing values: Methods like mean imputation or simply removing rows with missing data are used.
Detecting outliers: Outliers are identified using statistical methods, such as standard deviation or the Interquartile Range (IQR).
Deduplication: Exact or fuzzy matching algorithms identify and remove duplicates in datasets.
However, these traditional approaches come with significant limitations. For instance, rule-based systems often fail when dealing with unstructured data or context-specific errors. They also require constant updates to account for new data patterns.
LLM-driven approaches offer a more dynamic, context-aware solution to these problems.

How LLMs are Transforming Data Cleaning
1. Understanding Contextual Data Anomalies
LLMs excel in natural language understanding, which allows them to detect context-specific anomalies that rule-based systems might overlook. For example, an LLM can be trained to recognize that “N/A” in a field might mean "Not Available" in some contexts and "Not Applicable" in others. This contextual awareness ensures that data anomalies are corrected more accurately.
2. Data Imputation Using Natural Language Understanding
Missing data is one of the most common issues in data cleaning. LLMs, thanks to their vast training on text data, can fill in missing data points intelligently. For example, if a dataset contains customer reviews with missing ratings, an LLM could predict the likely rating based on the review's sentiment and content.
A recent study conducted by researchers at MIT (2023) demonstrated that LLMs could improve imputation accuracy by up to 30% compared to traditional statistical methods. These models were trained to understand patterns in missing data and generate contextually accurate predictions, which proved to be especially useful in cases where human oversight was traditionally required.
3. Automating Deduplication and Data Normalization
LLMs can handle text-based duplication much more effectively than traditional fuzzy matching algorithms. Since these models understand the nuances of language, they can identify duplicate entries even when the text is not an exact match. For example, consider two entries: "Apple Inc." and "Apple Incorporated." Traditional algorithms might not catch this as a duplicate, but an LLM can easily detect that both refer to the same entity.
Similarly, data normalization—ensuring that data is formatted uniformly across a dataset—can be automated with LLMs. These models can normalize everything from addresses to company names based on their understanding of common patterns and formats.
4. Handling Unstructured Data
One of the greatest strengths of LLMs is their ability to work with unstructured data, which is often neglected in traditional data cleaning processes. While rule-based systems struggle to clean unstructured text, such as customer feedback or social media comments, LLMs excel in this domain. For instance, they can classify, summarize, and extract insights from large volumes of unstructured text, converting it into a more analyzable format.
For businesses dealing with social media data, LLMs can be used to clean and organize comments by detecting sentiment, identifying spam or irrelevant information, and removing outliers from the dataset. This is an area where LLMs offer significant advantages over traditional data cleaning methods.
For those interested in leveraging both LLMs and DevOps for data cleaning, see our blog Leveraging LLMs and DevOps for Effective Data Cleaning: A Modern Approach.

Real-World Applications
1. Healthcare Sector
Data quality in healthcare is critical for effective treatment, patient safety, and research. LLMs have proven useful in cleaning messy medical data such as patient records, diagnostic reports, and treatment plans. For example, the use of LLMs has enabled hospitals to automate the cleaning of Electronic Health Records (EHRs) by understanding the medical context of missing or inconsistent information.
2. Financial Services
Financial institutions deal with massive datasets, ranging from customer transactions to market data. In the past, cleaning this data required extensive manual work and rule-based algorithms that often missed nuances. LLMs can assist in identifying fraudulent transactions, cleaning duplicate financial records, and even predicting market movements by analyzing unstructured market reports or news articles.
3. E-commerce
In e-commerce, product listings often contain inconsistent data due to manual entry or differing data formats across platforms. LLMs are helping e-commerce giants like Amazon clean and standardize product data more efficiently by detecting duplicates and filling in missing information based on customer reviews or product descriptions.

Challenges and Limitations
While LLMs have shown significant potential in data cleaning, they are not without challenges.
Training Data Quality: The effectiveness of an LLM depends on the quality of the data it was trained on. Poorly trained models might perpetuate errors in data cleaning.
Resource-Intensive: LLMs require substantial computational resources to function, which can be a limitation for small to medium-sized enterprises.
Data Privacy: Since LLMs are often cloud-based, using them to clean sensitive datasets, such as financial or healthcare data, raises concerns about data privacy and security.

The Future of Data Cleaning with LLMs
The advancements in LLMs represent a paradigm shift in how data cleaning will be conducted moving forward. As these models become more efficient and accessible, businesses will increasingly rely on them to automate data preprocessing tasks. We can expect further improvements in imputation techniques, anomaly detection, and the handling of unstructured data, all driven by the power of LLMs.
By integrating LLMs into data pipelines, organizations can not only save time but also improve the accuracy and reliability of their data, resulting in more informed decision-making and enhanced business outcomes. As we move further into 2024, the role of LLMs in data cleaning is set to expand, making this an exciting space to watch.
Large Language Models are poised to revolutionize the field of data cleaning by automating and enhancing key processes. Their ability to understand context, handle unstructured data, and perform intelligent imputation offers a glimpse into the future of data preprocessing. While challenges remain, the potential benefits of LLMs in transforming data cleaning processes are undeniable, and businesses that harness this technology are likely to gain a competitive edge in the era of big data.
#Artificial Intelligence#Machine Learning#Data Preprocessing#Data Quality#Natural Language Processing#Business Intelligence#Data Analytics#automation#datascience#datacleaning#large language model#ai
2 notes
·
View notes
Text
3 December 2023, Sunday
90 days of productivity
Things I have done:
🦉 Duolingo: German
📧 E-Mails (🥳)
🖥 Advent of Code
📔 Language and Literature Homework
🗒 Literature Revision
🗒 English Revision
📺 Video essay on the cruelty of infinite growth
💬 Discussion about philosophy
💬 Discussion about books with my brother
🤩 Philosophy
📚 Warum gibt es alles und nicht nichts? by Richard David Precht
Winter Studying Challenge
3rd December - What is your least favourite thing about winter?
Chapped lips and cracked skin on hands (yes, I'm aware of the existence of chapsticks and creams, they barely help)
Winter Wonderland Studyblr Challenge
DAY 9. Is there something that you specially like/dislike about holiday season?
I like the atmosphere of the holidays, the dishes, gifts and meeting some of my relatives. The commercialization of Christmas destroys this unique atmosphere, in my opinion. And I don't like rush and chaos.
#studyblr#studying#study motivation#50 days of productivity#50dop#100 days of productivity#100dop#ww studyblr challenge#winter studying challenge#2023#december
10 notes
·
View notes