#Admin Dashboard UI Kit
Explore tagged Tumblr posts
Text
Upgrade Project Design with Minimal lite Bootstrap Admin Template
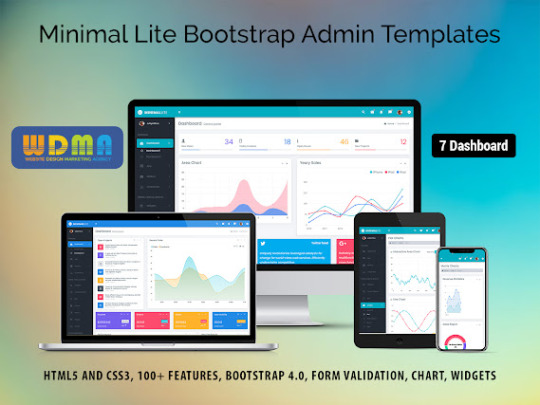
Minimal Lite – Responsive Web Application Kit boasts an extensive array of functionalities, including mobile responsiveness, flexible colour palettes, data presentation utilities, and intuitive interfaces. This Responsive Web Application Kit seamlessly integrates with numerous plugins and add-ons, enriching the administrative dashboard's capabilities. Minimal Lite comes complete with pre-built components, widgets, and styling alternatives, streamlining the development journey. Leveraging the Latest Bootstrap Beta Framework, alongside cutting-edge technologies HTML5 and CSS3, this Premium Admin Template ensures agility and adaptability. Lightweight and highly adaptable, it caters specifically to developers seeking customization options. For inquiries and acquisition of our sophisticated Bootstrap Admin Template.
#Responsive Web Application Kit#Responsive Admin Dashboard Template#Premium Admin Template#Bootstrap Admin Web App#Admin Dashboard Ui Kit#Dashboard Design#Admin Panel Dashboard#Admin Theme#WebApp Template#Dashboard UI Kit
0 notes
Text
A Comprehensive Guide to Bootstrap Admin & Dashboard Templates
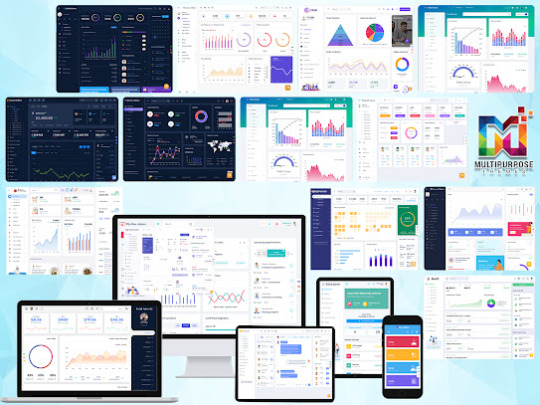
Dashboard Templates built with Bootstrap provide a quick and efficient way to create backend interfaces and web apps. With pre-built components, customizable layouts, and easy integration, a Bootstrap admin template helps developers skip repetitive coding tasks and focus on business logic. This comprehensive guide explores the key benefits of using Bootstrap for admin templates and dashboards.
Why Choose Bootstrap for Admin Templates
Built on top of the popular Bootstrap framework, Admin Dashboard UI Kit inherit several advantages:
Responsiveness Out-of-The-Box: With Bootstrap's mobile-first approach, components automatically adapt for desktop, tablet, and mobile screens. This saves ample development time.
Cross-Browser Consistency: Bootstrap templates render seamlessly across all modern browsers like Chrome, Firefox, Edge etc. There are no worries about CSS overrides.
Easy Customization: Variables and mixings allow easy styling changes sitewide. Tweaking colors, fonts, sizes to match brand requirements is straightforward.
Component Reuse: Bootstrap's UI library can be reused across templates and pages. No need to code repeating elements like cards, alerts, modal popups etc.
Continuous Updates: Bootstrap's open-source community steadily improves and extends functionalities. Admin templates built on Bootstrap allow leveraging the latest developments.
With these benefits, Bootstrap streamlines building admin backends and control panels, reducing cost and time-to-market.
#Dashboard Templates#Admin Dashboard UI Kit#Bootstrap UI Kit#Software Dashboard Design#Bootstrap UI framework#Responsive Web Application Kit#Bootstrap Admin Panel
0 notes
Text
#Playstation7 #framework #BasicArchitecture #RawCode #RawScript #Opensource #DigitalConsole
To build a new gaming console’s digital framework from the ground up, you would need to integrate several programming languages and technologies to manage different aspects of the system. Below is an outline of the code and language choices required for various parts of the framework, focusing on languages like C++, Python, JavaScript, CSS, MySQL, and Perl for different functionalities.
1. System Architecture Design (Low-level)
��� Language: C/C++, Assembly
• Purpose: To program the low-level system components such as CPU, GPU, and memory management.
• Example Code (C++) – Low-Level Hardware Interaction:
#include <iostream>
int main() {
// Initialize hardware (simplified example)
std::cout << "Initializing CPU...\n";
// Set up memory management
std::cout << "Allocating memory for GPU...\n";
// Example: Allocating memory for gaming graphics
int* graphicsMemory = new int[1024]; // Allocate 1KB for demo purposes
std::cout << "Memory allocated for GPU graphics rendering.\n";
// Simulate starting the game engine
std::cout << "Starting game engine...\n";
delete[] graphicsMemory; // Clean up
return 0;
}
2. Operating System Development
• Languages: C, C++, Python (for utilities)
• Purpose: Developing the kernel and OS for hardware abstraction and user-space processes.
• Kernel Code Example (C) – Implementing a simple syscall:
#include <stdio.h>
#include <unistd.h>
int main() {
// Example of invoking a custom system call
syscall(0); // System call 0 - usually reserved for read in UNIX-like systems
printf("System call executed\n");
return 0;
}
3. Software Development Kit (SDK)
• Languages: C++, Python (for tooling), Vulkan or DirectX (for graphics APIs)
• Purpose: Provide libraries and tools for developers to create games.
• Example SDK Code (Vulkan API with C++):
#include <vulkan/vulkan.h>
VkInstance instance;
void initVulkan() {
VkApplicationInfo appInfo = {};
appInfo.sType = VK_STRUCTURE_TYPE_APPLICATION_INFO;
appInfo.pApplicationName = "GameApp";
appInfo.applicationVersion = VK_MAKE_VERSION(1, 0, 0);
appInfo.pEngineName = "GameEngine";
appInfo.engineVersion = VK_MAKE_VERSION(1, 0, 0);
appInfo.apiVersion = VK_API_VERSION_1_0;
VkInstanceCreateInfo createInfo = {};
createInfo.sType = VK_STRUCTURE_TYPE_INSTANCE_CREATE_INFO;
createInfo.pApplicationInfo = &appInfo;
vkCreateInstance(&createInfo, nullptr, &instance);
std::cout << "Vulkan SDK Initialized\n";
}
4. User Interface (UI) Development
• Languages: JavaScript, HTML, CSS (for UI), Python (backend)
• Purpose: Front-end interface design for the user experience and dashboard.
• Example UI Code (HTML/CSS/JavaScript):
<!DOCTYPE html>
<html>
<head>
<title>Console Dashboard</title>
<style>
body { font-family: Arial, sans-serif; background-color: #282c34; color: white; }
.menu { display: flex; justify-content: center; margin-top: 50px; }
.menu button { padding: 15px 30px; margin: 10px; background-color: #61dafb; border: none; cursor: pointer; }
</style>
</head>
<body>
<div class="menu">
<button onclick="startGame()">Start Game</button>
<button onclick="openStore()">Store</button>
</div>
<script>
function startGame() {
alert("Starting Game...");
}
function openStore() {
alert("Opening Store...");
}
</script>
</body>
</html>
5. Digital Store Integration
• Languages: Python (backend), MySQL (database), JavaScript (frontend)
• Purpose: A backend system for purchasing and managing digital game licenses.
• Example Backend Code (Python with MySQL):
import mysql.connector
def connect_db():
db = mysql.connector.connect(
host="localhost",
user="admin",
password="password",
database="game_store"
)
return db
def fetch_games():
db = connect_db()
cursor = db.cursor()
cursor.execute("SELECT * FROM games")
games = cursor.fetchall()
for game in games:
print(f"Game ID: {game[0]}, Name: {game[1]}, Price: {game[2]}")
db.close()
fetch_games()
6. Security Framework Implementation
• Languages: C++, Python, Perl (for system scripts)
• Purpose: Ensure data integrity, authentication, and encryption.
• Example Code (Python – Encrypting User Data):
from cryptography.fernet import Fernet
# Generate a key for encryption
key = Fernet.generate_key()
cipher_suite = Fernet(key)
# Encrypt sensitive user information (e.g., account password)
password = b"SuperSecretPassword"
encrypted_password = cipher_suite.encrypt(password)
print(f"Encrypted Password: {encrypted_password}")
# Decrypting the password
decrypted_password = cipher_suite.decrypt(encrypted_password)
print(f"Decrypted Password: {decrypted_password}")
7. Testing and Quality Assurance
• Languages: Python (for automated tests), Jest (for JavaScript testing)
• Purpose: Unit testing, integration testing, and debugging.
• Example Unit Test (Python using unittest):
import unittest
def add(a, b):
return a + b
class TestGameFramework(unittest.TestCase):
def test_add(self):
self.assertEqual(add(10, 20), 30)
if __name__ == '__main__':
unittest.main()
8. Order of Implementation
• Phase 1: Focus on core system architecture (low-level C/C++) and OS development.
• Phase 2: Develop SDK and start building the basic framework for UI and input management.
• Phase 3: Work on backend systems like the digital store and integrate with front-end UI.
• Phase 4: Begin rigorous testing and debugging using automated test suites.
This comprehensive approach gives you a detailed idea of how each component can be built using a combination of these languages and technologies. Each section is modular, allowing teams to work on different aspects of the console simultaneously.
[Pixel Art/Sprites/Buildinodels/VictoriaSecretPixelArtKit #Playstation7 #DearDearestBrands]


#victoriasecretrunway#runwaybattle#victoria secret fashion#victoria secert model#victoriasecret#victoria secret#VictoriaSecretRunwayBattle#capcom victoriasecret#capcom videogames#playstation7#ps7#deardearestbrands#capcom#digitalconsole#python#script#rawscript#raw code#framework#VictoriaSecretVideoGame#deardearestbrandswordpress
2 notes
·
View notes
Text
Why Should You Go for a Ready-Made Cryptocurrency Exchange Script?
In this article about Why Should You Go for a Ready-Made Cryptocurrency Exchange Script by BlockchainX

Launching a cryptocurrency exchange represents a highly profitable business choice within the fast-moving blockchain and digital finance sector. The construction of one from the beginning stage takes an extended period of time alongside high financial costs and extensive technical expertise. The market offers pre-built cryptocurrency exchange scripts which provide a solution.
Crypto exchange software solutions provide businesses with a time-saving approach to launch their own trading platforms at affordable costs. The question remains whether this approach suits every business person. The following section explores various advantages of selecting a ready-made cryptocurrency exchange script which benefits both new economic entities and well-established financial corporations.
What Is a Cryptocurrency Exchange Script?
A cryptocurrency exchange script provides businesses with a pre-built software kit which contains mandatory features to operate an electronic trading exchange for digital currencies. It typically includes modules for:
User registration and login
Wallet integration
KYC/AML compliance
Trading engine
Admin dashboard
Security features
White-label availability in these scripts enables businesses to customize their branding as well as adapt certain features to meet their individual business requirements.
Top 6 Reasons to Choose a Ready-Made Crypto Exchange Script
Faster Time-to-Market
The process to develop a crypto exchange starts at six months but extends to a year based on how complex the system becomes. The deployment of a ready-made script takes a duration ranging from days to weeks.
Time serves as the crucial factor you need while attempting to outperform your competition and access new market openings. A pre-built script enables you to enter the market efficiently right away while collecting user feedback to refine your product without starting from scratch.
Cost-Effective Development
Creating customized exchanges demands that businesses hire full-stack developers alongside blockchain engineers alongside user interface designers as well as quality assurance testers along with additional staff. The development expenses range widely from $50,000 to $500,000 and above.
A ready-made script cuts down overall development expenses. The price of high-quality script varies between $5,000 and $50,000 according to the combination of features and support and license requirements. Small enterprises and startup companies gain access to the crypto market through affordable solutions.
Battle-Tested Security
Any crypto exchange needs security to be among its most vital components. Many scripts integrate security protocols from the start which include these features:
Two-Factor Authentication (2FA)
Data encryption
Secure wallet management
Anti-DDoS protection
Withdrawal whitelist features
The security of these scripts has been tested in live environments and continually improved because they were previously used in operational settings where a newly built system could be prone to security weaknesses.
Customizable and Scalable
People usually connect script usage with inflexibility but reality shows otherwise. Sunlight reveals that the majority of premium exchange scripts come with complete customization options. You can:
Add or remove features
Change the UI/UX
Integrate third-party APIs
Adjust trading pairs and liquidity sources
Incorporate payment gateways
The growth of your exchange allows you to enhance your platform features and operation scope. The script serves as a solid base that you will continue to enhance.
Built-in Compliance Tools
Regulations vary widely across regions. A high-quality script contains built-in security modules for KYC/AML inspection and GDPR compliance tools which help users maintain legal framework compliance.
Using a script that includes compliance features enables companies to reach users in multiple countries while avoiding later legal and development complexities.
Support and Maintenance
Professional crypto script providers maintain a commitment to supply extended customer support which incorporates system maintenance as well as application updates. Inside developers are not necessary to manage your complete technical operations because of the script's functionality. Expert support allows you to develop your business and acquire users without needing to handle the development directly
Conclusion
A fully developed trading platform script presents businesses with an innovative and protected entry point into the cryptocurrency ecosystem. The ready-made cryptocurrency exchange script becomes an optimal solution for businesses operating at different levels of growth including startups working with new models and fintech companies entering blockchain segments and entrepreneurs who need accelerated launches.
You can focus on expanding your operations while acquiring users and innovating when you select a trusted exchange script from a reliable provider even though proper script review remains essential.
In the world of crypto, speed and adaptability win. A pre-built exchange script presents itself as a top solution to gain market advantage.
#cryptocurrency#blockchain technology#cryptocurrency exchange script#cryptocurrency exchnage#ready made exchange script
0 notes
Text
Last Call! Iqonic March Madness Sale – Up to 50% OFF On Best Products
youtube
⏳ FINAL CALL! This is your last chance to grab the biggest discounts of the year! The Iqonic March Madness Sale is ending SOON! Get up to 50% OFF on top-selling digital products before it’s too late!
🔥 Best Deals You Can’t Miss: ✔️ Prokit – 50% OFF 🔥 The Ultimate Flutter UI Kit! ✔️ Streamit – Up to 50% OFF 🎬 Build Your Own Streaming Platform! ✔️ Graphina – 50% OFF 📊 Create Stunning Data Visualizations! ✔️ Handyman – 50% OFF 🛠️ The Ultimate Home Services App! ✔️ KiviCare – Up to 50% OFF 🏥 Power-Packed Clinic Management! ✔️ SocialV – Up to 50% OFF 🌍 Build Your Online Community! ✔️ Frezka – 50% OFF 💇♀️ The Ultimate Salon & Spa Solution! ✔️ Pawlly – 50% OFF 🐶 All-in-One Pet Care Solution! ✔️ KiviCare Laravel – 40% OFF 🔥 Advanced Clinic Management! ✔️ WPBookit – 40% OFF 📅 The Best Appointment Booking Plugin! ✔️ Streamit Laravel – 30% OFF 🎬 A Feature-Rich OTT Platform! ✔️ Hope UI – 40% OFF 💻 The Ultimate Admin Dashboard! ✔️ Vizion AI – 40% OFF 🎨 AI-Powered Creativity at Its Best!
🚨 Time is running out! Once the clock hits zero, these deals will be gone forever! Shop Now Before It’s Too Late!
For More Details Visit - https://bit.ly/4cf0uw1
MarchMadnessSale #MarchMadnessSale2025 #MarchSale #MarchSale2025 #saleyearend #yearendsale #Yearendsale2025 #MarchDeals #IqonicMarchMadnesssale #IqonicMarchsale #IqonicMarchDeals #IqonicMarchSale2024 #IqonicYearEndSale #yearenddeals #saleforprofessionals #salefordevelopers #developersdeals #developerssale #webdevelopersdeals #salefordevelopersanddesigners #saleonwordpressthemes #saleonflutterapps #wordpressthemes #flutterapps #UIKits #admindashboards #wordpressplugin #softwaredeals #softwaresale #marchsalefordevelopersanddesigners
0 notes
Text
Live preview must check
eCommUIUX Ecommerce & Inventory Admin Dashboard Bootstrap 5 HTML Temaplate
Start eCommerce and Inventory Management Platform with our eCommUIUX a Premium Bootstrap 5 Admin HTML Template
Designed specifically for eCommerce and Inventory Management Admin – web – dashboard applications, our eCommUIUX admin UI kit template offers a sleek, clean, trending design, and fully multipurpose responsive solution. This powerful admin ui template, built on the latest Bootstrap 5 framework, ensures a seamless user experience across major devices, from tablet and smartphones.
Clean and Professional Aesthetic: A visually appealing design that enhances brand credibility.
Flexible and Customizable: Easily tailor the template to your specific needs.
Webpack-Powered: Streamlined development workflow for efficient project management.
Partial View Codes: Reusable components for faster development and maintenance.
Ideal for: eCommerce Platforms, Online Product Selling Admin, Inventory Management Software, Virtual Multi user Store handling.Take your eCommerce platform to the next level with eCommUIUX.
Key Features:
50+ Ready-to-Use Pages: Includes a wide range of pre-built pages for dashboards, transactions, portfolio management, user profiles, and more.
14+ Customizable Styles: Offers a variety of pre-defined styles and color schemes to match your brand identity.
100+ UI Components: Provides a rich library of UI components, including charts, graphs, tables, and more.
Checkout now our product and portfolio 🏄♀️
💌 Email Us: [email protected]
😍 Social media : Instagram | Linked In | X/Twitter
🛍️ Adminuiux Product: Themeforest | Gumroad
Live preview must check
#ecommerce#bootstrap5#htmltemplates#inventorymanagement#adminuiux#admin#dashboard HTML#bootstrapthemes#ordermanagement#bootstra#css#trending#vira
0 notes
Text
20+ Free Admin Dashboard Templates for Figma – Speckyboy
New Post has been published on https://thedigitalinsider.com/20-free-admin-dashboard-templates-for-figma-speckyboy/
20+ Free Admin Dashboard Templates for Figma – Speckyboy
A great dashboard is both attractive and informative. Users should be able to get what they need effortlessly. The look should be clean and easy to understand. The result is something users want to visit time and again.
Designing a dashboard from scratch is a huge task, though. Things can get complicated in a hurry with so many widgets competing for attention. Who has the time to deal with all of this?
That’s what makes a Figma template so helpful. A beautiful and functional design is already in place. There are also components for you to use, duplicate, and customize. That means your project will be off to a running start.
Does it sound like something you could use? If so, check out our collection of free admin dashboard templates for Figma. There are options here for virtually every use case. Choose your favorite and get started!
You might also like our collection of web and mobile UI templates for Figma.
Give users an easy-to-navigate experience with this Figma UI template. It features a high-contrast color scheme, beautiful design components, and outstanding typography. Use it, and you’ll have a professional-grade dashboard in no time.
Download this Figma dashboard template and gain access to over 500 UI components. You’ll find charts, buttons, card layouts, navigation bars, and more. It provides the ultimate flexibility for your project.
Here’s a UI kit that includes everything you need to build a dashboard layout. It includes multiple screens in both light and dark modes. It also uses Figma variables for easier customization.
Crown is a dashboard template inspired by Material Design – Google’s open-source design system. This step makes everything seem intuitive and familiar. The components are colorful, and the layout is roomy.
This open-source dashboard template was designed to work with React. The package includes several templates and components with light and dark versions. It’s a versatile choice for building web applications.
Create an analytics-focused dashboard using this Figma template. It features a modern aesthetic and support for multiple color schemes. The template uses layers, making it easy to customize to suit your needs.
Kanban boards are great for organizing information for individuals and teams. This Figma template uses the concept to help you build a task management app. Use its clean design to improve communication and stay focused.
Sales Analytics Dashboard UI Kit has 16 predesigned screens for different use cases. You’ll also find plenty of widgets, well-organized layers, and an easy-to-customize setup. It’s also built for accessibility and meets WCAG 2 requirements.
The components included in this UI kit will make your dashboard project a breeze. It’s all here: dropdowns, modal windows, navigation, charts, form elements, and more. Use them to build a custom application that’s beautiful and functional.
Here’s a different take on the traditional dashboard screen. NewsNet focuses on content more than statistics. That makes it a great choice for company intranets or personalized web portals. There are several creative possibilities here.
This free dashboard UI kit focuses on finance. You might use it for a company’s accounting department or as part of an employee portal. The design is clean and easy to read.
Create a custom dashboard layout in minutes using these beautifully designed component cards. Mix and match them to display an array of stats and info. These colorful cards are flexible, and many include crisp graphics.
This stylish template is perfect for use as an analytics dashboard. It includes all the basics in a simple and colorful layout. Customize it to your heart’s content. You’ll save time without sacrificing quality.
BankDash is a free dashboard UI kit that includes over 300 screen layouts. It uses the latest Figma features such as variables and auto layout. That makes it a fit for virtually any type of project.
There’s a lot to like about this free dashboard template. It’s clean, colorful, and includes mobile and desktop viewports templates. You’ll find plenty of resources to get your project off the ground.
This free Figma dashboard template includes plenty of ready-made components. Each can be customized to fit your content and color scheme. Pick your favorites and build a user-friendly interface!
Do you want to build a collaborative dashboard? This calendar UI template will give you a terrific head start. It includes views for mobile and desktop. In addition, it outlines tasks in an easy-to-follow format.
Digesto is a dashboard template that focuses on content organization. It’s perfect for user portals, client reputation tracking, or any project where media is front and center. The template includes six screens and several attractive components.
This free open-source admin dashboard kit includes an atomic design system. The template features UI elements like tables, charts, forms, etc. You’ll also have access to light and dark versions in an easy-to-edit package.
With more than 350 global styles and 10,000+ components, Untitled UI is a powerful package. That provides plenty of options for building a dashboard to match your needs. If you can dream it, you can do it.
Use this dashboard UI kit for real estate and property management projects. Its well-organized layout will help users stay on top of their tasks in style. The kit includes one screen, a component set, and a style guide.
Form UI Kit uses a monochromatic color scheme to enhance legibility. It includes all the basics to build an attractive and functional dashboard. There’s enough here to cover a variety of needs.
Users of Tailwind CSS will want to check out this admin dashboard template. The kit includes four distinctive dashboard layouts and over 400 UI elements. It’s a great way to combine the popular CSS framework with your dashboard project.
Build a Beautiful Dashboard in Less Time
Dashboards are among the most important and most difficult design projects. Users depend on them to perform tasks and gather information. However, building an effective one requires excellent attention to detail.
The templates in this collection are designed to make your job easier. They provide a solid foundation to build upon. The design and layout are taken care of. That allows you to focus on executing your plan.
Now that you have so many outstanding templates within reach – what will you build?
More Dashboard Templates
If you’re looking for a fully designed HTML dashboard UI kit or template, we have many to choose from. You will find free Bootstrap templates here, and free Tailwind CSS templates here.
Related Topics
Top
#000#Accessibility#accounting#admin#Analytics#app#applications#atomic#attention#boards#Bootstrap#Building#buttons#Calendar#charts#collaborative#Color#communication#content#CSS#custom dashboard#Dark#dashboard#deal#Design#desktop#display#easy#Features#figma
0 notes
Text
Download Bootstrap 5 Admin & Dashboard themes Mobile App UI/UX HTML templates, Admin UI UX Dashboard HTML templates UI kit finance inventory, clinic, learning
0 notes
Text
Metronic HTML Template: Elevate Your Best Web Design Game
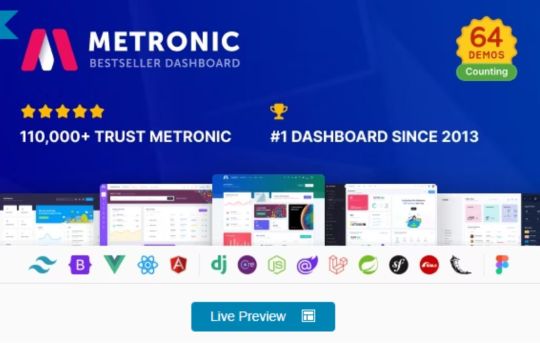
Are you looking for a reliable admin dashboard template to power your next project? Look no further than Metronic Html Template! This powerful tool is designed to help you create beautiful and intuitive admin interfaces that will impress your clients and users alike.
In this review, we’ll take a closer look at what makes Metronic Html Template such a great choice for developers and businesses alike. We’ll explore its features, functionality, and compatibility with popular frameworks like Tailwind, Bootstrap, React, Vue, Angular, Asp.Net & Laravel. So, let’s dive in!
Features
Metronic Html Template comes loaded with a wealth of features that make it an excellent choice for developers and businesses alike. Some of its standout features include:
– High Resolution: Metronic Html Template is optimized for high-resolution displays, so your dashboard will look crisp and clear on any device. – Responsive Layout: The template is designed to be fully responsive, so your dashboard will look great on any screen size.
– Well Documented: Metronic Html Template comes with comprehensive documentation to help you get up and running quickly.
– Compatible Browsers: The template is compatible with all popular web browsers, including Firefox, Safari, Opera, Chrome, and Edge.
– Compatible With: Metronic Html Template is compatible with Angular 13.x.x, AngularJS, ReactJS, Bootstrap 5.x, Bootstrap 4.x, and other popular frameworks.
– Admin Dashboard Template: Metronic Html Template is designed specifically for use as an admin dashboard template, so you can be sure it has all the features you need to create a powerful and intuitive dashboard.
– Admin Themes: The template comes with a range of pre-built themes to help you get started quickly.
– PHP Files: Metronic Html Template comes with all the PHP files you need to get started quickly.
– HTML Files: The template comes with a range of pre-built HTML files, so you can get started quickly.
– CSS Files: Metronic Html Template comes with a range of pre-built CSS files to help you customize your dashboard.
– Sass Files: The template includes Sass files for advanced customization.
– SCSS Files: The template includes SCSS files for advanced customization.
– JS Files: Metronic Html Template includes a range of pre-built JavaScript files to help you get started quickly.
Compatibility
Metronic Html Template is compatible with a wide range of popular frameworks and platforms, including:
– Tailwind – Bootstrap – React – Vue – Angular – Asp.Net & Laravel
This makes it an excellent choice for developers who want a flexible and versatile tool that can be used with a variety of different frameworks and platforms.
12 Advanced Apps For Real-world Demands
Complete CRUD solution with managable datatables, advance form controls, wizards flows and interactive modals for any project requirements you can imagine
Metronic UI Kit Develop Design Fast
Create cohesive user interfaces for single or multiple projects without having to start from scratch. Metronic UI Kit is helpful for designers who are just starting out or who are working on projects with tight deadlines.
Company made it! Smart & Low-cost!
One stop solution that boosts your projects’ design and development at shortest amount of time and at ridiculously low cost. In the past 10 years, hundreds of thousands of web apps successfully launched by Metronic that are used by hundreds of millions of end users everyday
Pricing
Metronic Html Template is available for purchase on ThemeForest for just $49. This includes a Regular License, which allows you to use the template in a single end product that is not charged for. If you need to use the template in a product that will be sold to end users, you can purchase an Extended License for $969.
If you purchase the Regular License, you’ll receive quality checking by Envato, future updates, and six months of support from keenthemes. You can also extend your support to 12 months for an additional fee.
Reviews
Mr. Levan Dvalishvili Chief (Software Architect) at solarspace.io said Hands down the most developer friendly package that I have worked with.. A+++++
platform we tried out Metronic. I can not overestimate the impact Metronic has had. Its accelerated development 3x and reduced QA issues by 50%. If you add up the reduced need for design time/resources, the increase in dev speed and the reduction in QA, it’s probably saved us $100,000 on this project alone, and I plan to use it for all platforms moving forward. The flexibility of the design has also allowed us to put out a better looking & working platform and reduced my headaches by 90%. Thank you KeenThemes! Jonathan Bartlett, Metronic Customer
Metronic is an incredible template. Even with purchasing an extended license, the cost savings is immeasurable. The code & CSS is well organized and while it is feature rich, it is not bloated. It was quickly integrated into our relatively complex product and had ready-made UX for our many use cases. Where we needed to extend functionality, this system made it incredibly easy. A tremendous job by the Metronic team. Fatica, Metronic Customer
Conclusion
In conclusion, Metronic Html Template is an excellent choice for developers and businesses alike. It comes loaded with features, is compatible with a wide range of popular frameworks and platforms, and is available at an affordable price. Whether you’re building a new dashboard from scratch or looking to upgrade an existing one, this Template is definitely worth considering.
So, what are you waiting for? Head over to ThemeForest and check out Metronic Html Template today!
#admin dashboard template#admin themes#angular#asp.net core#blazor#bootstrap#bootstrap 5#django#html#laravel#metronic#react#tailwind#tailwind css#vuejs
0 notes
Text
Power Apps Online Training | Microsoft Power Apps Course
Top 20 Microsoft PowerApps Tools
As of my last knowledge update in January 2022, there isn't a specific list of "Top 20 Microsoft PowerApps Tools" widely recognized or maintained by Microsoft. However, I can provide you with information about some key components and tools within the Microsoft Power Platform, which includes PowerApps. Please note that developments may have occurred since my last update, and it's always a good idea to check the official Microsoft documentation for the latest information. Here are some essential components and tools related to Microsoft PowerApps
Power Apps and Power Automate Training
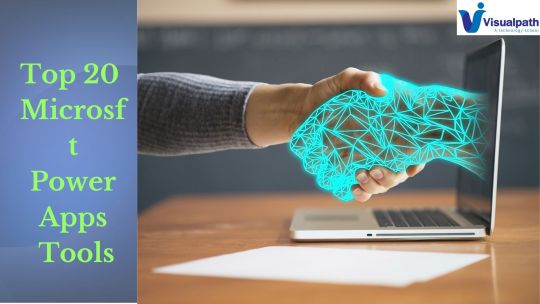
PowerApps Studio: The main development environment for creating and designing PowerApps.
Canvas Apps: PowerApps allows you to create custom apps using a canvas where you can design the user interface and functionality.
Model-Driven Apps: A type of app that is defined by its data model, with components like forms, views, and dashboards automatically generated from that model.
PowerApps Portals: Allows external users to interact with data stored in the Common Data Service.
Common Data Service (CDS): A database that allows you to securely store and manage data used by business applications.
Connectors: PowerApps supports various connectors to integrate with external services and data sources such as SharePoint, SQL Server, Microsoft 365, and more. - Microsoft Power Apps Online Training
AI Builder: Enables users to add artificial intelligence capabilities to their apps, such as object detection, prediction, and language understanding.
Power Automate: Formerly known as Microsoft Flow, it allows you to automate workflows between your apps and services.
Power Virtual Agents: Enables the creation of intelligent chatbots without requiring extensive coding.
Power BI Integration: PowerApps can be integrated with Power BI for powerful data visualization and analysis.
PowerApps Component Framework (PCF): Allows developers to build custom UI components for PowerApps.
- Microsoft Power Platform Online Training in Ameerpet
Power Platform Admin Center: Provides administrative capabilities for managing environments, resources, and monitoring usage.
PowerApps Mobile App: Allows users to access PowerApps on mobile devices.
Data Integration: PowerApps allows for the seamless integration of data from various sources.
Custom Connectors: Extend the capabilities of PowerApps by creating custom connectors to connect with external services.
Azure DevOps Integration: Enables integration with Azure DevOps for version control, continuous integration, and deployment.
Dataverse for Teams: A low-code data platform for Microsoft Teams that allows users to build custom apps.
ALM (Application Lifecycle Management): Tools and processes for managing the entire lifecycle of PowerApps applications.
- Power Apps Training in Ameerpet
Power Platform Center of Excellence (CoE) Starter Kit: A set of templates, apps, and flows for setting up a CoE to govern Power Platform usage within an organization.
Solution Checker: Helps ensure the quality and performance of your PowerApps solutions.
Keep in mind that the Power Platform is continually evolving, and new tools or features may have been introduced since my last update. Always refer to the official Microsoft documentation and community resources for the latest information on PowerApps and the Power Platform.
Visualpath is the Leading and Best Software Online Training Institute in Ameerpet, Hyderabad. Avail complete job-oriented Microsoft Power Platform Online Training by simply enrolling in our institute in Ameerpet, Hyderabad. You will get the best course at an affordable cost.
Attend Free Demo
Call on - +91-9989971070.
WhatsApp: https://www.whatsapp.com/catalog/919989971070
Visit: https://visualpath.in/microsoft-powerapps-training.html
#Power Apps and Power Automate Training#Microsoft Power Apps Online Training#Microsoft Power Apps Course#Power Apps Online Training#Power Apps Training#Power Apps Training Hyderabad#Power Apps Training in Ameerpet#Microsoft Power Platform Online Training in ameerpet
0 notes
Text
ALUI Developed by Thememakker - Bootstrap-Powered, Best-Ever Dashboard Template
In the fast-paced world of web development, having the right tools at your disposal can make all the difference. Thememakker, a leading web design company, brings you ALUI - an admin dashboard template that's set to revolutionize your web development projects. In this article, we'll delve into what makes ALUI a standout choice for developers.
Bootstrap Powered Excellence
ALUI powered by Bootstrap, the industry-standard framework that provides a solid foundation for web development. With Bootstrap, you can expect clean, responsive, and mobile-friendly designs, ensuring your website looks great on any device. Thememakker has taken Bootstrap and elevated it to the next level with ALUI, making it the ideal choice for any company's needs.
A Simple Three-Step Process
ALUI helps you streamline your web development process by following a straightforward, three-step process:
Download Template
First, ensure that your license aligns with your project requirements. Download the latest version of the ALUI template from Themeforest, and you're ready to kickstart your project.
Choose Template
Browse through a multitude of templates tailored for various technologies and business niches. ALUI offers a wide variety of options to cater to your specific needs.
Ready to Work
You can set up a starter kit for your preferred technology or select an HTML template that suits your project requirements. ALUI ensures you have the flexibility to choose what works best for you.
Discover ALUI's Features and functionalities
ALUI is feature-rich and comes with seamless technology integration and a range of benefits, including:
Powerful Dashboard Widgets
ALUI boasts incredibly powerful dashboard widgets developed by experienced professional designers. These widgets are designed with a standardized structure to future-proof your projects.
A few lines of code are all it takes to invoke these dynamic widgets, which support various technologies and breathe life into your dashboard.
Highly Standardized UI/UX with an Easy-to-Use Interface
ALUI's highly standardized styles come with centralized elements and widgets, making it a breeze to use these elements in your projects. Whether you're working on a Hospital UI or a HR-Project management widget, ALUI's design architecture ensures versatility.
Uniquely Handcrafted Layouts
ALUI offers over 20 handcrafted layouts created by passionate web designers at Thememakker. These layouts are designed to make your project visually stunning and highly functional.
Planned Smart Updates
ALUI keeps your project up to date effortlessly. By replacing styles without requiring any coding or structural changes, ALUI ensures your project remains current. Major updates are designed to affect the core structure minimally, making the process smooth and hassle-free.
Centralized Widget Library
ALUI provides access to a centralized widget library that houses styles for creating widgets from various niches. This means you can take widgets from different niches and use them in your project, transforming it into a multi-domain platform.
Seamlessly Integrated Layouts
Uniquely designed layouts of ALUI look stunning and are logically integrated into different platforms. Whether you're using Angular, ReactJS, Laravel, HTML, VueJS, or VueJS + Laravel, ALUI makes it easy to update routes and elements in your menus.
High Contrast Theme for Better Accessibility
ALUI features a pre-built high-contrast theme that enhances accessibility. When you adhere to ALUI's standards, this theme is automatically applied to all elements, including charts and labels.
10 Reasons to Choose ALUI Admin Dashboard Developed by Thememakker
Developer-Friendly: ALUI is built on the Bootstrap 5 Design Framework, making it easy for team members to understand and integrate niche components.
Feature-Rich: ALUI offers a broad range of features that can be added to your product, impressing end-users.
Billions of Theming Possibilities: Customize your theme to match your brand using dynamic theme options.
Suitable for All: ALUI is designed for professionals, brands, and countries, offering multiple themes, layouts, business segments, and more.
SUPER Time Saver: ALUI's flexible architecture and prebuilt layouts save you time and effort in theme integration.
Simple Yet Powerful: Create stunning dashboards with ease, making ALUI one of the most productive theme design on the market.
Tons of Pages: With over 5000 pages and a variety of widgets, ALUI caters to a wide range of needs.
Made by Developers, For Developers: Empower developers with a powerful UI Kit, making development more efficient.
Easy to Customize: Once you understand ALUI's architecture, customization is a breeze, allowing you to design new widgets and dashboards with dynamic theme options.
Lifetime Updates: ALUI offers lifetime updates to stay in line with the latest trends.
ALUI caters to a variety of technologies, including:
ReactJS: Prebuilt ReactJS dashboard with a starter kit.
Angular: Prebuilt Angular dashboard with a starter kit.
Laravel: Prebuilt Laravel dashboard with a starter kit.
HTML: A set of mighty HTML dashboard templates.
VueJS: Prebuilt VueJS dashboard with a starter kit.
VueJS + Laravel: Prebuilt VueJS + Laravel dashboard with a starter kit.
ASP .NET Core MVC: Prebuilt ASP .NET Core MVC starter kit (Coming soon).
1,000+ Satisfied Users
ALUI's designs have been loved by millions, with positive feedback from clients and users. It's a testament to the excellence of Thememakker's work.
ALUI - A New Standard in Dashboard Design
ALUI is not just an ordinary theme; it's a full library of widgets and layouts designed to take your web development services to the next level. With ALUI, you have the tools and flexibility to create stunning dashboards that meet your unique requirements.
FAQs Clear your all doubts
Do I need to purchase a license for each website?
Yes, you must purchase licenses separately for each of your websites. An extended license is required for commercial sites.
What is a regular license?
A regular license is suitable for end products that do not charge users for access or service. It can be used for a single end product.
What is an extended license?
An extended license is necessary for end products (web services or SAAS) that charge users for access or service. It can also be used for a single-end product.
Which license is applicable for SASS applications?
If you are charging users for using your SASS-based application, you must purchase an extended license for each product. A regular license is suitable when users access your service for free.
What are the lifetime updates for ALUI?
Lifetime updates are offered for all ALUI frameworks and libraries to keep them up to date with the latest trends, ensuring your projects remain current and competitive.
youtube
#ALUI Admin Dashboard#Thememakker#Web Development#Dashboard Template#Bootstrap-Powered#Developer-Friendly#Web Design#Feature-Rich Dashboard#Theming Options#Lifetime Updates#Web Development Tools#Bootstrap Framework#Layouts and Widgets#UI/UX Design#Developer Support#Dynamic Theme Options#Web Development Efficiency#Web Development Projects#User-Friendly Dashboard#Web Development Benefits#Web Development Revolution#Thememakker's ALUI#Responsive Design#Web Development Excellence#Widget Library#Web Development Flexibility#Hand-Crafted Layouts#High Contrast Theme#Smart Updates#Bootstrap Integration
0 notes
Text
Empower Your Web Development with Premium Admin Template : Aries Admin
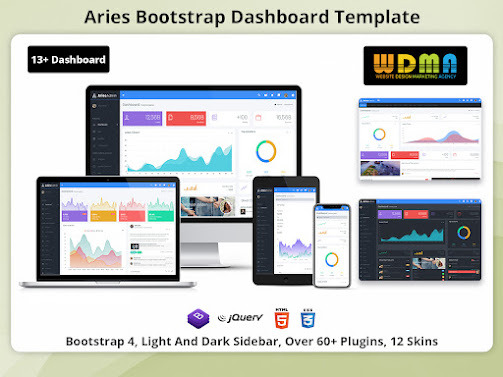
Introducing the Aries Premium Admin Template – meticulously engineered to cater to the dynamic needs of admin panels and dashboards. Featuring an extensive array of functionalities, our Responsive Web Application Kit boasts over 13 diverse dashboards tailored to empower your E-commerce operations. Today, our spotlight shines on the E-commerce dashboard, a powerhouse of data visualization. At its core lies the campaign chart, offering a comprehensive overview of impressions, top performers, conversions, and CPA metrics. This Responsive Admin Dashboard Template dashboard encapsulates vital insights ranging from new client acquisitions to product launches and invoicing activities. Seamlessly integrated modules like 'My New Clients,' 'New Products,' and 'New Invoices' provide real-time updates, ensuring you stay ahead of the curve.Beyond its E-commerce prowess, our admin template presents a plethora of features designed to streamline your workflow. Dive into the world of applications, leverage intuitive mailbox functionalities, and explore an extensive library of UI elements. With customisable widgets at your disposal, including dynamic blog widgets, charts, tables, and email templates, your possibilities are boundless. Furthermore, harness the power of maps and extensions to enhance user experience and extend functionality. Crafted with developers in mind, our combines versatility with ease of integration, enabling you to build robust solutions effortlessly.Experience the epitome of modern admin management with the Aries Admin Dashboard UI Kit – where innovation meets efficiency.
#Responsive WebApplication Kit#Responsive Admin Dashboard Template#Premium Admin Template#Bootstrap Admin Web App#Admin Dashboard Ui Kit
0 notes
Text
The Increasing Popularity of Cryptocurrency Dashboard

Cryptocurrencies have exploded in popularity over the last few years. What started as an obscure technology embraced by a niche community has transformed into a global phenomenon with a market capitalization of over $1 trillion. With this rapid growth, there has been increasing demand for Cryptocurrency Dashboard and services to help users track and manage their digital assets.
The Need for Cryptocurrency Dashboards
One area that has seen particular innovation is Cryptocurrency Dashboard Template. Cryptocurrency dashboards provide users with an easy way to get an at-a-glance view of the overall cryptocurrency market as well as the performance of their personal portfolio. The best cryptocurrency dashboards condense a wealth of complex data into simple, visually appealing graphics and charts that allow users to comprehend the volatile cryptocurrency landscape within seconds. For cryptocurrency companies and traders, having access to a well-designed dashboard is absolutely essential.
The Rise of Cryptocurrency Dashboard Templates
In response to this need, there has been an influx of cryptocurrency dashboard template options and themes that aim to provide an off-the-shelf solution. These dashboard templates, such as Crypto Admin Template and Bitcoin Dashboard Theme, enable companies to bypass the elaborate development process and launch a functional dashboard quicker and more affordably. They provide the foundational interface and integrate with cryptocurrency APIs to fetch data like prices, market capitalization, trade volume, and more. Users can then customize the look and feel of the dashboard to match their brand.
#Crypto Admin Templates#Cryptocurrency Admin Template#Crypto Admin Dashboard#Cryptocurrency Dashboard Template#Cryptocurrency Dashboard#Crypto Admin UI Kit
0 notes
Text
Power BI Admin Template Dashboard Web App with UI Framework

Power BI Admin Template Dashboard has a decent arrangement of elements that should make it a popular option. If you check out the online demo of this template set, you’ll get an accurate idea of what Power BI Responsive Bootstrap 5 Admin can do. In the demo, you’ll see a significant number of the valuable components and blocks in action, like tables, diagrams, timelines, and more. As Power BI Dashboard comes with a ton of jazzy icons, illustrate your pages with these elements is simple and easy. Works on all major web browsers, Desktop, iPhone, iPad, Tablet and all other smartphone devices.
MORE INFO / BUY NOW DEMO
Like Us
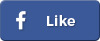
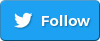
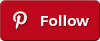
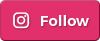
#Admin Dashboard#power bi admin dashboard#power bi admin template#webapp template#dashboard design#Bootstrap 5 Admin Templates#bootstrap admin templates#bootstrap admin template#Admin Templates#admin panel dashboard#responsive bootstrap 5 admin#responsive admin dashboard template#responsive web application kit#Bootstrap Admin Web App#bootstrap admin dashboard#responsive admin dashboard#Admin Dashboard UI Kit#admin templates bootstrap#Admin Dashboard templates#Dashboard Admin Templates
0 notes
Text
Crypto Admin Dashboard Bootstrap 5 Template UI Kit with Sass
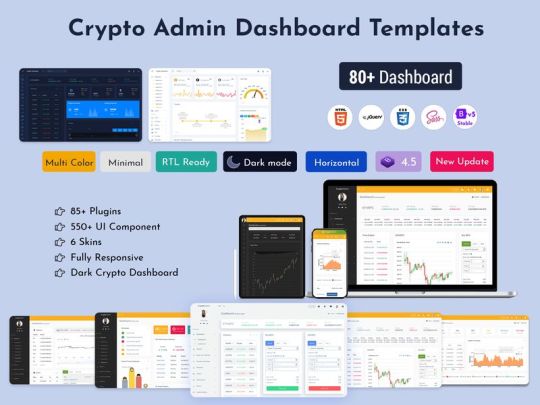
Bitcoin Crypto Admin Dashboard Bootstrap 5 Admin Template UI and WebApp Template is a fully responsive Cryptocurrency Dashboard Admin Template built with Bootstrap 5 and 4.2 Framework, modern web technology HTML5 and CSS3. Lightweight, SasS, and easy customizable which is basically designed for the developers who want to customize it. Crypto Admin can be used by developers developing web applications like custom admin panel, ICO, Bitcoin Dashboards, Bitcoin and other current website applications, project management system, admin dashboard, application backend, CMS, CRM, Stock chart, Stock Market, Business Analytics, business website, corporate, portfolio, blog, etc.
MORE INFO / BUY NOW DEMO
Like Us



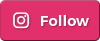
#bootstrap 5 dashboard#Bootstrap 5Admin Templates#Bootstrap Admin Dashboard#Bootstrap Admin HTML#Bootstrap Admin Template#Bootstrap Admin Templates#Admin Dashboard#Admin Dashboard responsive template#Cryptocurrency Admin Dashboard Template#admin dashboard templates#admin dashboard ui#Admin Dashboard UI Kit#Admin Templates Bootstrap#admin theme#Responsive Bootstrap 5 Admin#responsive dashboard#crypto dashboard#cryptocurrency#creative dashboard design#Crypto Tokenize Admin Template#Tokenize Admin Template#Tokenize CryptoCurrency Admin Templates#Bootstrap 5 Template#Crypto Admin Dashboard
0 notes
Text
Great News! 🎊 March Madness Sale Extended – Up to 50% OFF! 🚀
youtube
🎉 Great News! Our March Madness Sale is Extended Until April 3rd! 🚀 Get up to 50% OFF on our top-selling products and take your projects to the next level!
🔥 What’s on Sale? ✅ Premium Admin Dashboards & UI Kits 🎨 ✅ Top-Tier WordPress Plugins & Themes 🔥 ✅ Flutter Apps & Templates at Huge Discounts! 📱
💥 Don’t Miss This Extended Offer – Shop & Save Big Before It’s Gone!
For More Details Visit - bit.ly/4l3CgbU
MarchMadnessSale #MarchMadnessSale2025 #MarchSale #MarchSale2025 #saleyearend #yearendsale #Yearendsale2025 #MarchDeals
IqonicMarchMadnesssale #IqonicMarchsale #IqonicMarchDeals #IqonicMarchSale2024 #IqonicYearEndSale #yearenddeals #saleforprofessionals
salefordevelopers #developersdeals #developerssale #webdevelopersdeals #salefordevelopersanddesigners #saleonwordpressthemes #saleonflutterapps
#wordpressthemes #flutterapps #UIKits #admindashboards #wordpressplugin #softwaredeals #softwaresale #marchsalefordevelopersanddesigners
0 notes